How to write trycatch in R
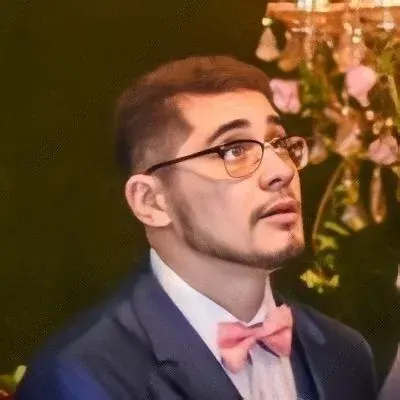
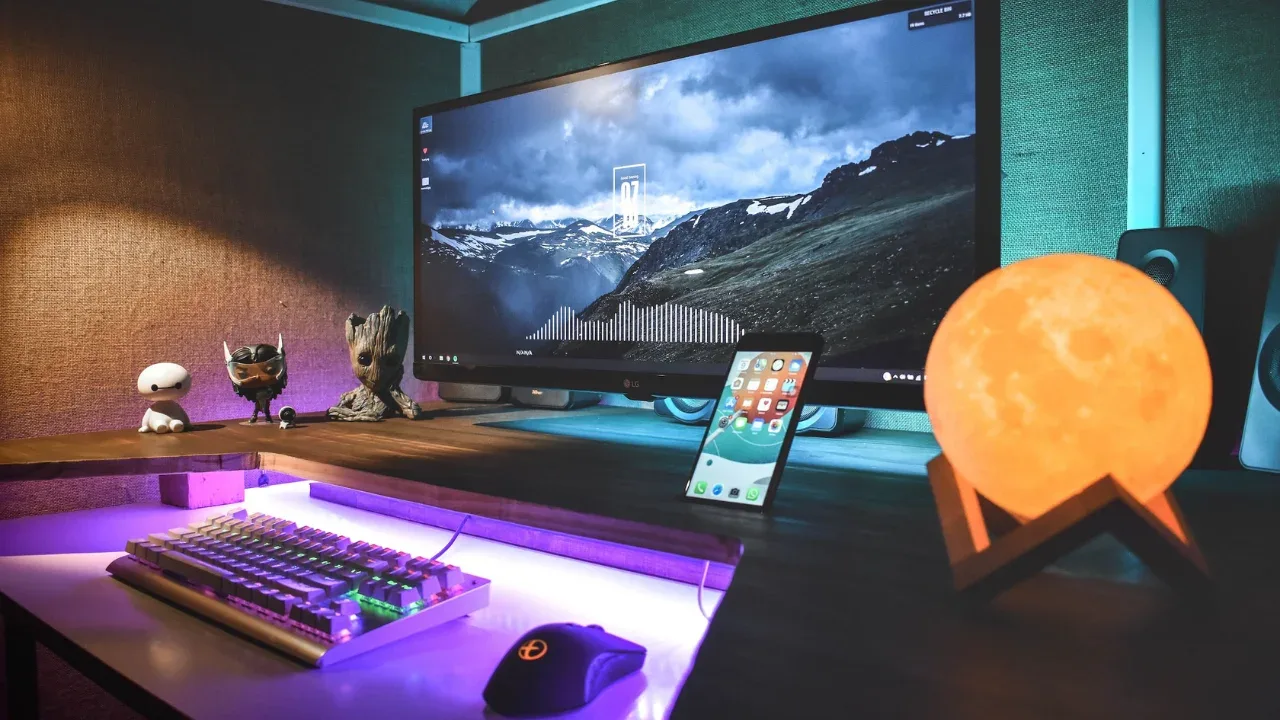
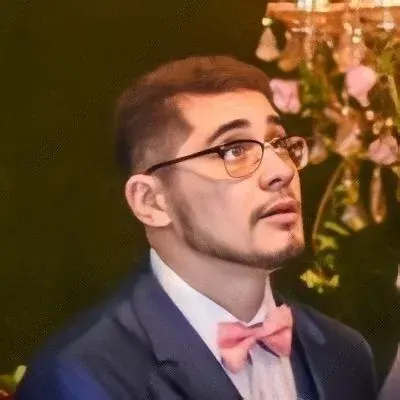
How to Write Try-Catch in R: Dealing with Errors in Web Downloading
π Hey there, R enthusiasts! ππ» Are you tired of your code breaking every time you encounter an error while downloading data from the web? Well, fret no more! π
ββοΈ Today, we're going to learn how to use the powerful trycatch
function in R to handle these errors gracefully and keep your code running smoothly. ππ¨
The Problem: Dealing with Errors in Web Downloading
Let's start by setting the scene. You have some URLs that you want to download data from. πβ¨ You might have a list of URLs stored in a vector, like this:
url <- c(
"http://stat.ethz.ch/R-manual/R-devel/library/base/html/connections.html",
"http://en.wikipedia.org/wiki/Xz"
)
Great! You successfully downloaded the data from these URLs using the readLines
function. ππ
y <- mapply(readLines, con = url)
However, what if you encounter an incorrect or non-existent URL? π± Take a look at this example:
url <- c("xxxxx", "http://en.wikipedia.org/wiki/Xz")
Uh-oh! π¬ The first URL, url[1]
, is non-existent. We don't want our code to come to a screeching halt just because one URL is wrong. Instead, we want to handle these errors gracefully and continue downloading the remaining URLs. But how can we achieve that? π€
The Solution: Using Try-Catch to Handle Errors
The answer lies in the mighty trycatch
function! π¦ΈββοΈπ¦ΈββοΈ This powerful function allows us to catch and handle errors without interrupting the execution of our code. Let's see how it works in practice:
trycatch(expr, error = function(e) {})
The trycatch
function takes two arguments:
expr
: The expression you want to evaluate and potentially catch errors from.error
: A function that will be executed if an error occurs. Here, you can define the actions you want to take when an error happens.
Now, let's apply this to our web downloading scenario:
download_url <- function(url) {
tryCatch({
readLines(url)
}, error = function(e) {
message(paste("Error downloading", url, ":", conditionMessage(e)))
return(NULL)
})
}
urls <- c("xxxxx", "http://en.wikipedia.org/wiki/Xz")
results <- lapply(urls, download_url)
In this code snippet, we've defined a function download_url
that wraps the tryCatch
function around the readLines
call. If an error occurs, the error
function will be executed. Inside the error
function, we print a custom error message using message
and return NULL
to indicate that the download failed.
We then use the lapply
function to apply the download_url
function to each URL in the urls
vector. This ensures that even if one URL fails, the code continues executing for the remaining URLs.
Handling Specific Errors
Now that we've got a basic understanding of how to use trycatch
, let's address the specific problems you mentioned:
Handling wrong URLs: To detect and handle wrong URLs specifically, we can check the error message using
conditionMessage(e)
inside theerror
function. Here's an updated version of thedownload_url
function:download_url <- function(url) { tryCatch({ readLines(url) }, error = function(e) { if (grepl("could not resolve host", conditionMessage(e))) { message("Web URL is wrong, can't get") } else { message(paste("Error downloading", url, ":", conditionMessage(e))) } return(NULL) }) }
If the error message contains the string "could not resolve host", we know that the URL is wrong. In that case, we print the custom error message "Web URL is wrong, can't get". Otherwise, we print the general error message including the URL.
Continuing downloading: As mentioned earlier, using
lapply
will automatically continue downloading for the remaining URLs even if one of them fails. So, we're all good on that front! π
A Call to Action: Share Your Success Stories!
Congratulations! ππ You've now mastered the art of using trycatch
in R to handle errors while downloading data from the web like a true coding superhero! π¦ΈββοΈπ¦ΈββοΈ
But wait! We'd love to hear about your own experiences with handling errors in R. Have you come across any tricky situations? How did you solve them? Share your stories in the comments below and let's learn from each other! ππ‘
Stay tuned for more awesome R tips and tricks on our blog. Until next time, happy coding! ππ₯