How to use R"s ellipsis feature when writing your own function?
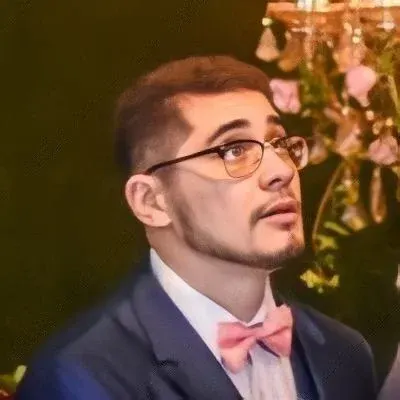
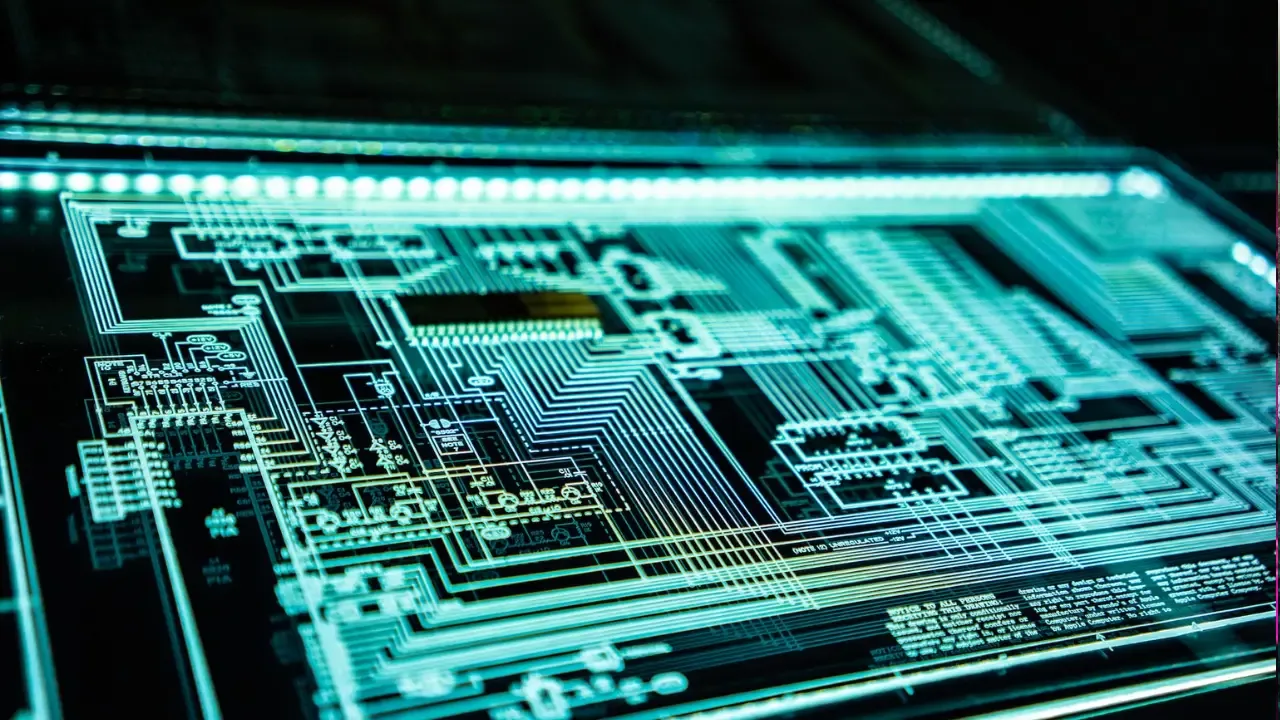
How to use R's ellipsis feature when writing your own function? 📝🔬
Have you ever wanted to write a function in R that can take a variable number of arguments? If so, you're in luck! 🍀 The R language has a nifty feature called the ellipsis (...
) that allows you to do just that. 🎉
Understanding the ellipsis feature 🤔
The ellipsis feature in R allows you to define a function that can accept any number of arguments. When you see the ellipsis in a function's signature, like this:
my_function(...) {
# function body here
}
It means that the function can take multiple arguments, and you can access these arguments within the function using the ...
symbol.
How to unpack the ellipsis 📦
To unpack the ellipsis and convert it into a more usable form, such as a list, you can use the as.list(substitute(list(...)))[-1L]
syntax. Let's break down what this does:
substitute(list(...))
captures the arguments passed to the function as an unevaluated expression.as.list(...)
converts the expression into a list.[-1L]
removes the first element of the list, which is the expression itself.
Putting it all together, you can define a helper function like this:
get_list_from_ellipsis <- function(...) {
as.list(substitute(list(...)))[-1L]
}
Example usage 🖥️
Let's say you want to write a function called my_ellipsis_function
that takes multiple arguments and consolidates them into a single output list. You can use the get_list_from_ellipsis
function we defined earlier to achieve this. Here's an example:
my_ellipsis_function <- function(...) {
input_list <- get_list_from_ellipsis(...)
output_list <- lapply(input_list, FUN=do_something_interesting)
return(output_list)
}
my_ellipsis_function(a=1:10, b=11:20, c=21:30)
In the example above, my_ellipsis_function
takes three arguments (a
, b
, and c
), which are passed as a list to input_list
using get_list_from_ellipsis
. Then, we can perform some interesting processing on each element of the input_list
using lapply
and return the resulting list.
Choosing between as.list(substitute(list(...)))[-1L]
and list(...)
🤷♂️
You might be wondering why we used as.list(substitute(list(...)))[-1L]
instead of simply using list(...)
. While both approaches achieve similar results, there are some practical differences to consider.
as.list(substitute(list(...)))[-1L]
captures the unevaluated expression and gives you more control over how you handle the arguments.list(...)
converts the arguments directly into a list without capturing the expression. This can be more convenient in some cases, but it may limit your flexibility in handling the arguments.
In most cases, using as.list(substitute(list(...)))[-1L]
would be the safer and more flexible option, especially when dealing with complex function arguments.
Conclusion and next steps 👏✍️
Congratulations! You now know how to use R's ellipsis feature when writing your own functions. This powerful feature allows you to create functions that can handle a variable number of arguments, making your code more flexible and reusable. 🚀
To further enhance your R coding skills, you can explore more about function arguments, such as default values, named arguments, and type checking. Keep experimenting and happy coding! 💻😊
Have you used R's ellipsis feature in your own functions before? How has it helped you? Share your experiences and any additional tips in the comments below! Let's learn and grow together! 👇🌱
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
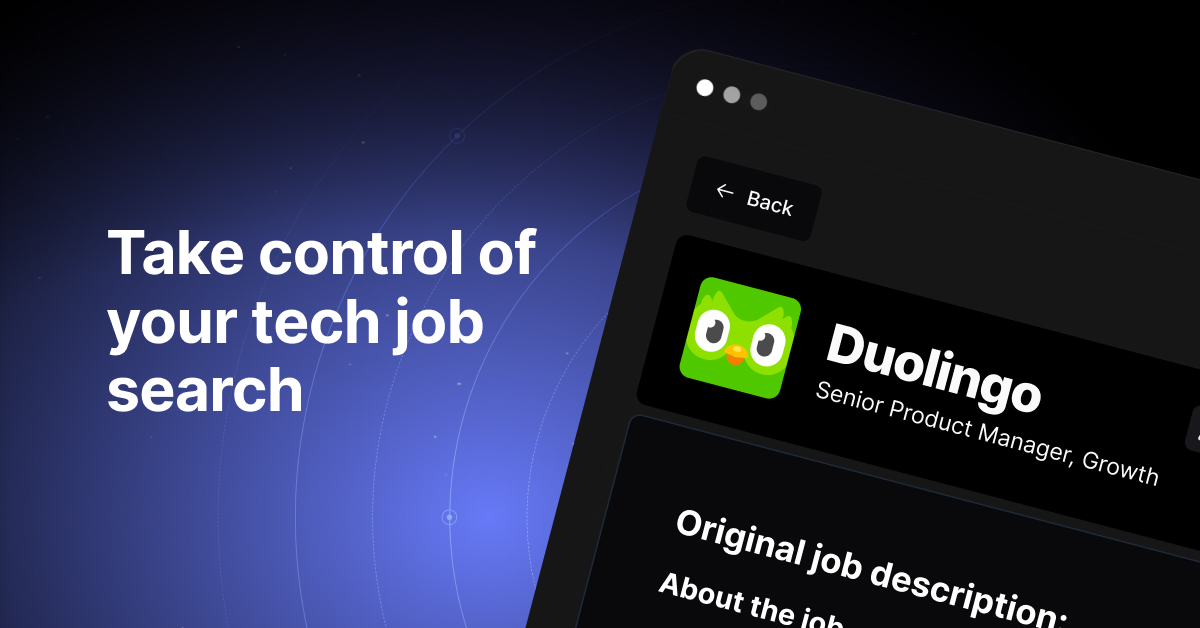