How to split data into training/testing sets using sample function
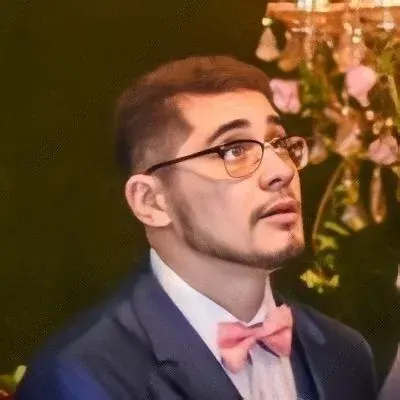
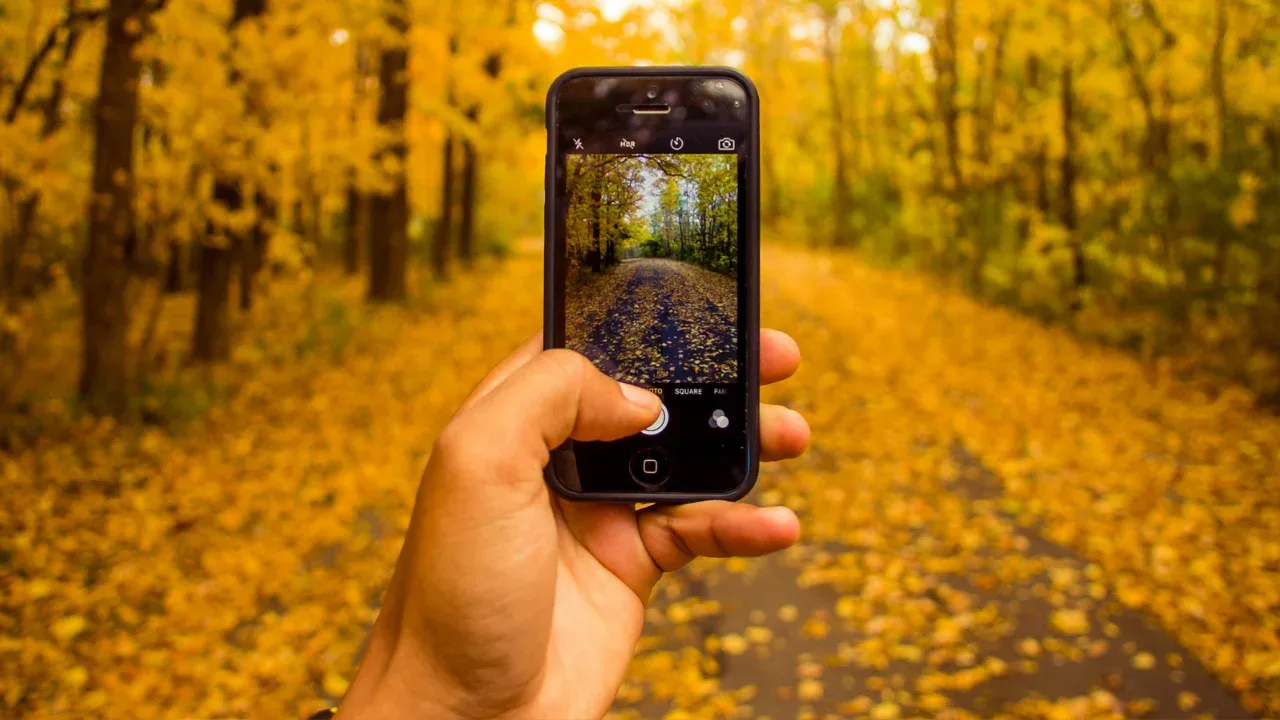
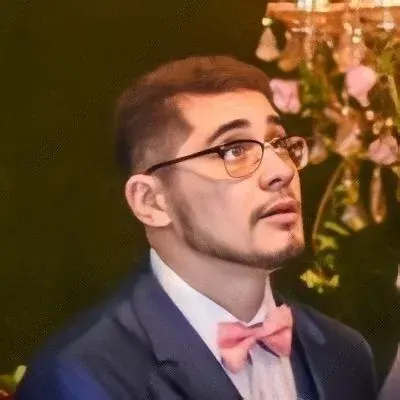
Splitting Data into Training/Testing Sets in R using the 'sample' Function
If you are new to R and have been struggling with splitting your dataset into training and testing sets, you have come to the right place! In this blog post, we will guide you through the process of using the sample
function to achieve this goal. 🚀
Understanding the sample
function
The sample
function in R is a powerful tool that allows you to randomly select elements from a vector or data frame. With the proper usage, it can help us create a representative training/testing split for our dataset. Let's take a closer look at its syntax:
sample(x, size, replace = FALSE, prob = NULL)
x
: This represents the vector or data frame from which you want to sample. In our case, it would be the dataset you are working with.size
: Here, you specify the number of samples you want to draw from the givenx
. This will be used to determine the size of your training/testing sets.
Now that we have a better understanding of the sample
function, let's dive into the steps to split your data.
Step-by-Step Guide to Splitting Your Data
Step 1: Load your dataset
Before we begin, make sure your dataset is loaded into R. You can use various methods to load your data, such as read.csv()
, read.table()
, or any other relevant function depending on your file type.
Step 2: Set the seed (optional)
If you want to reproduce the same training/testing split every time, it's a good practice to set the seed using the set.seed()
function. This guarantees that the random sampling will be consistent across different runs of your code.
Step 3: Split the data
Now, let's implement the sample
function to split your dataset into training and testing sets:
set.seed(42) # Optional step to ensure reproducibility
training_indices <- sample(nrow(dataset), round(0.75 * nrow(dataset)), replace = FALSE)
training_set <- dataset[training_indices, ]
testing_set <- dataset[-training_indices, ]
In the above code snippet,
nrow(dataset)
returns the total number of rows in your dataset.We multiply
0.75
by the total number of rows to determine the desired size of the training set.The
replace = FALSE
argument ensures that each observation is selected only once, preventing duplicates.training_indices
stores the randomly selected row indices for the training set.Lastly, we use negative indexing (
-training_indices
) to select the remaining rows for the testing set.
And voilà! You have successfully split your data into a training set (75%) and a testing set (25%) using the sample
function.
Troubleshooting Common Issues
Issue 1: "Error in sample.int"
Sometimes, you might encounter an error message like "Error in sample.int" when executing the sample
function. This error typically occurs when the x
argument is not correctly specified. Make sure you pass in a valid vector or data frame as a parameter.
Issue 2: Imbalanced dataset
If your dataset is imbalanced, meaning the number of observations for different classes is uneven, you might end up with an imbalanced split. In such cases, it is advisable to use stratified sampling techniques to ensure a representative split.
Conclusion and Call-to-Action
Congratulations! You have learned how to split your data into training and testing sets using the sample
function in R. We hope this guide has made the process clear and hassle-free for you. Now, go ahead and implement this technique in your code to enhance your machine learning models or data analysis projects.
If you found this post helpful, don't forget to share it with your fellow R enthusiasts and leave a comment below sharing your experience with data splitting or any related questions. We would love to hear from you! 😊