How to remove all whitespace from a string?
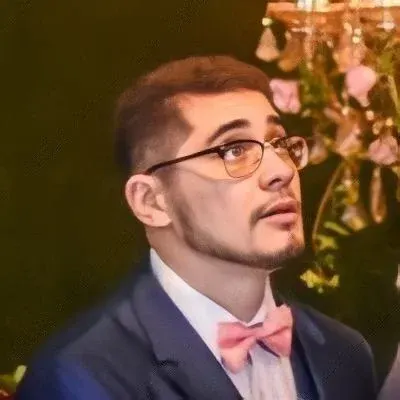

🧹 HOW TO REMOVE ALL WHITESPACE FROM A STRING? 🧹
Are you tired of dealing with pesky whitespace in your strings? Want to clean up your data and make it more presentable? Look no further! In this guide, we will show you easy ways to remove all whitespace from a string using different programming languages. Let's dive in and make your strings sparkle! 💫
💡 Understanding the Problem
Before we jump into the solutions, let's understand the problem at hand. Imagine you have a string like this:
" xx yy 11 22 33 "
And you want to remove all the whitespace from it, resulting in:
"xxyy112233"
🖥️ Languages and Solution Approaches
Python 🐍
Python provides a straightforward solution using the replace()
method. Here's an example code snippet:
string_with_whitespace = " xx yy 11 22 33 "
string_without_whitespace = string_with_whitespace.replace(" ", "")
print(string_without_whitespace)
Output:
"xxyy112233"
JavaScript 🌐
In JavaScript, you can achieve the same result using a regular expression and the replace()
method. Here's an example code snippet:
const stringWithWhitespace = " xx yy 11 22 33 ";
const stringWithoutWhitespace = stringWithWhitespace.replace(/\s/g, "");
console.log(stringWithoutWhitespace);
Output:
"xxyy112233"
Java ☕
In Java, you can utilize the replaceAll()
method with a regular expression to remove whitespace from a string. Here's an example code snippet:
String stringWithWhitespace = " xx yy 11 22 33 ";
String stringWithoutWhitespace = stringWithWhitespace.replaceAll("\\s", "");
System.out.println(stringWithoutWhitespace);
Output:
"xxyy112233"
🎉 Conclusion and Call-to-Action
Congratulations! You now know how to remove all whitespace from a string using Python, JavaScript, and Java. Bid farewell to messy strings and say hello to clean, elegant data. 🎊
Now it's your turn to put this knowledge into practice! Try it out with your own strings and see the magic happen. Don't hesitate to share your experience or ask any further questions in the comments section below. Let's make whitespace-free strings the new trend in the programming world! 💪💻
Happy coding! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
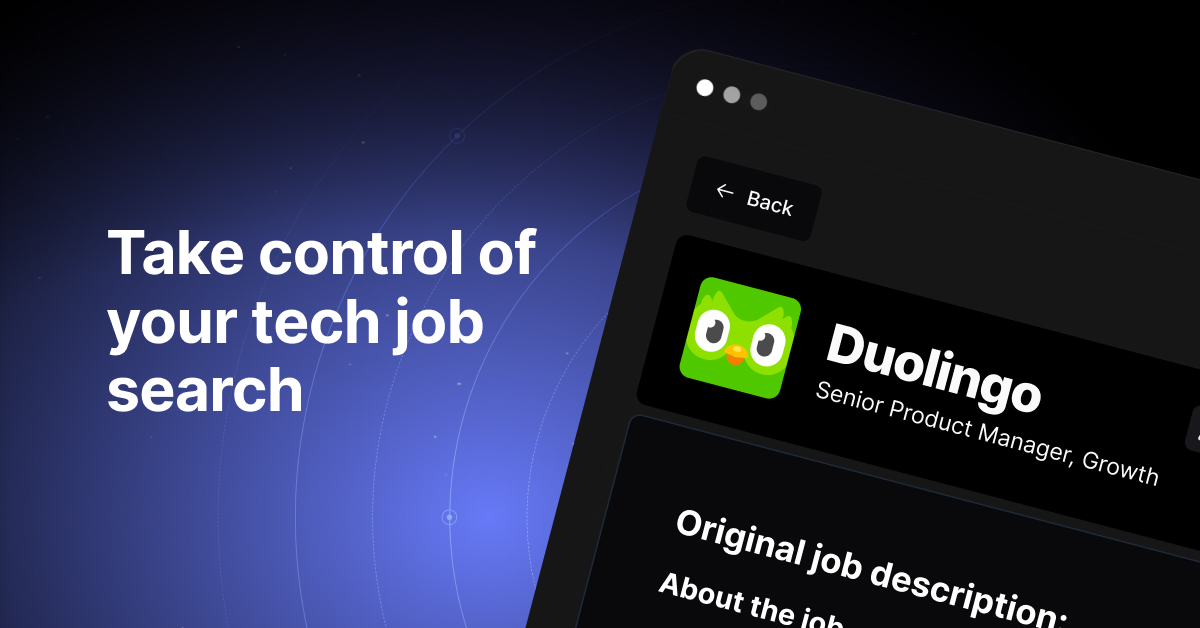