How to join (merge) data frames (inner, outer, left, right)
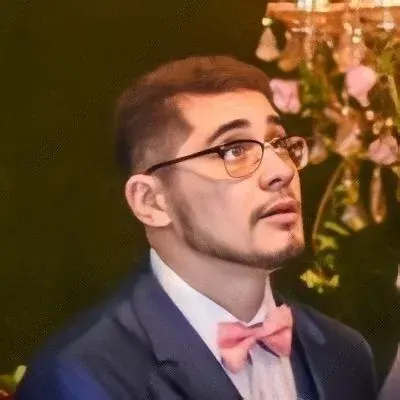
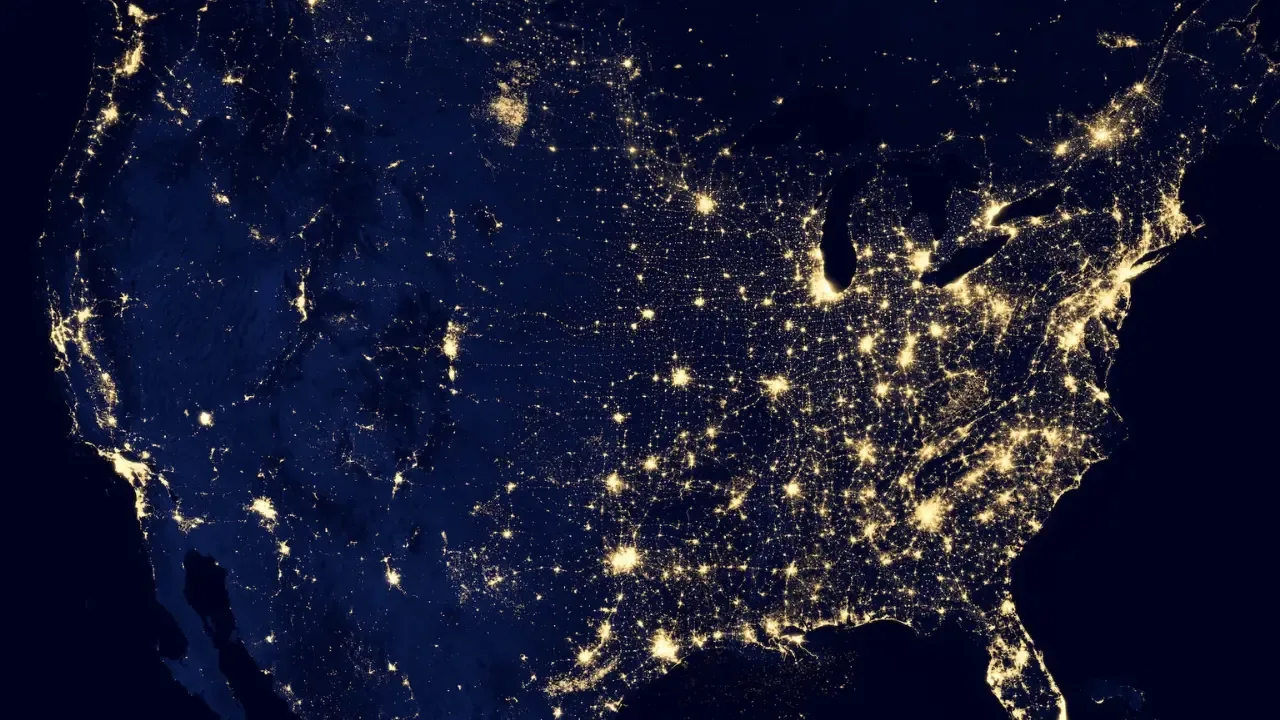
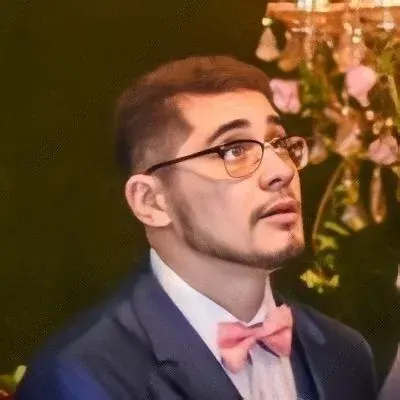
How to Join (Merge) Data Frames 🔄📊
Have you ever found yourself struggling to combine data frames in R? Do you need to perform different types of joins like inner join, outer join, left join, or right join? Look no further! In this blog post, we will guide you through the process of joining (merging) data frames using database-style joins in R. 📚💻
The Problem 😕
Let's start with a common scenario. Suppose we have two data frames: df1
and df2
. df1
contains information about customers and the products they purchased, while df2
contains information about customers and their states. Here's what they look like:
df1 = data.frame(CustomerId = c(1:6), Product = c(rep("Toaster", 3), rep("Radio", 3)))
df2 = data.frame(CustomerId = c(2, 4, 6), State = c(rep("Alabama", 2), rep("Ohio", 1)))
df1
# CustomerId Product
# 1 1 Toaster
# 2 2 Toaster
# 3 3 Toaster
# 4 4 Radio
# 5 5 Radio
# 6 6 Radio
df2
# CustomerId State
# 1 2 Alabama
# 2 4 Alabama
# 3 6 Ohio
Now, the question arises: How do we combine these data frames, performing different types of joins? Let's explore the solutions! 🚀
The Solution 💡
Inner Join ⚙️
An inner join returns only the rows where the keys in the left table have matching keys in the right table. In other words, it combines the data frames by the common values in a specific column. To perform an inner join in R, we can use the merge()
function:
inner_join_result = merge(df1, df2, by = "CustomerId")
inner_join_result
The output will be:
CustomerId Product State
1 2 Toaster Alabama
2 4 Radio Alabama
3 6 Radio Ohio
Outer Join 🔄
An outer join returns all rows from both tables, joining records from the left table that have matching keys in the right table. In R, we can achieve an outer join by setting the all
parameter to TRUE
in the merge()
function:
outer_join_result = merge(df1, df2, by = "CustomerId", all = TRUE)
outer_join_result
The output will be:
CustomerId Product State
1 1 Toaster <NA>
2 2 Toaster Alabama
3 3 Toaster <NA>
4 4 Radio Alabama
5 5 Radio <NA>
6 6 Radio Ohio
Left Join ⬅️
A left join returns all rows from the left table and any rows with matching keys from the right table. In R, this is achieved by setting all.x
parameter to TRUE
in the merge()
function:
left_join_result = merge(df1, df2, by = "CustomerId", all.x = TRUE)
left_join_result
The output will be:
CustomerId Product State
1 1 Toaster <NA>
2 2 Toaster Alabama
3 3 Toaster <NA>
4 4 Radio Alabama
5 5 Radio <NA>
6 6 Radio Ohio
Right Join ➡️
A right join returns all rows from the right table and any rows with matching keys from the left table. In R, we can achieve a right join by setting all.y
parameter to TRUE
in the merge()
function:
right_join_result = merge(df1, df2, by = "CustomerId", all.y = TRUE)
right_join_result
The output will be:
CustomerId Product State
1 2 Toaster Alabama
2 4 Radio Alabama
3 6 Radio Ohio
Extra Credit 💯
SQL Style Select Statement 🗃️
Apart from joining data frames, you might also want to select specific columns from the merged data frame. In SQL, you can achieve this using a select statement. In R, we can use the $
operator or the subset()
function to select specific columns from the merged data frame. Here's an example:
selected_columns = inner_join_result$Product
selected_columns
Or
selected_columns = subset(inner_join_result, select = Product)
selected_columns
Both of these methods will give you the following output:
[1] Toaster Toaster Toaster Radio Radio Radio
Levels: Radio Toaster
Conclusion 🎉
Joining data frames in R doesn't have to be intimidating or confusing. By using the merge()
function and understanding different types of joins, you can effortlessly combine your data frames and gain valuable insights. So give it a try with your own data frames and start unlocking the power of data merging! 💪
If you found this blog post helpful, let us know in the comments below. We'd love to hear about your experiences and any other questions you may have. Keep exploring and happy coding! 😃📈👨💻