For each row in an R dataframe
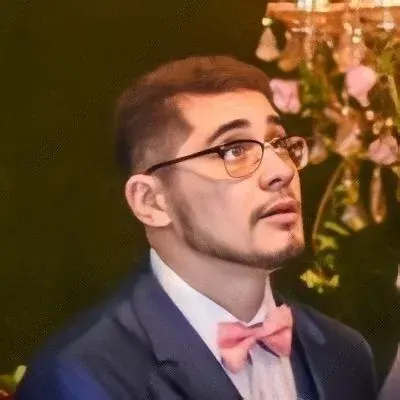
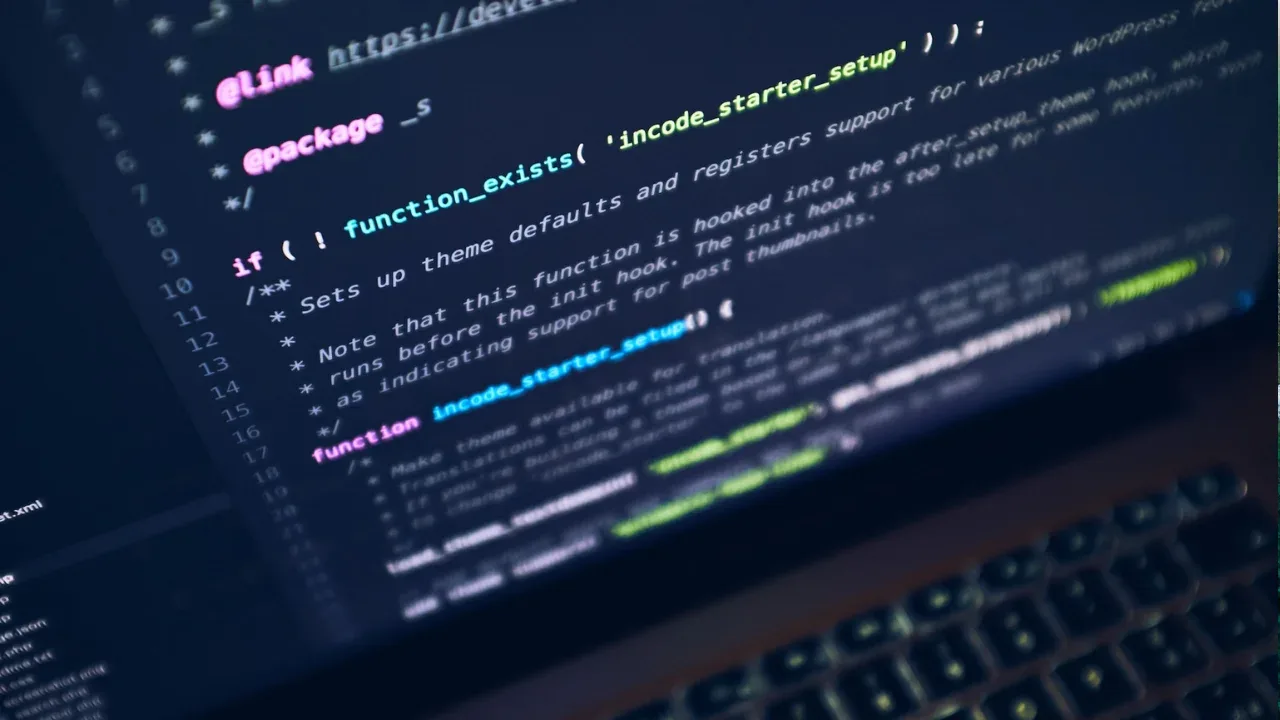
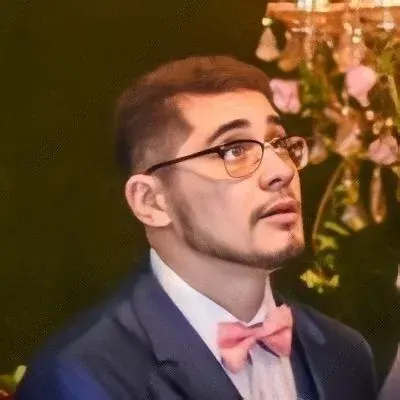
📚 Unlocking the "R Way" to Work with Each Row in a DataFrame 📚
Are you struggling to iterate over each row in an R DataFrame to perform complex lookups and append data to a file? Fear not! In this blog post, we'll explore the "R way" to tackle this problem and provide you with easy solutions to make your life easier. 🚀
🎯 Understanding the Problem
Let's dig into the context: you have a DataFrame that contains scientific results for selected wells from 96 well plates used in biological research. Your goal is to perform some intricate lookups and append specific data to a file for each row in the DataFrame. Sounds a bit tricky, right? But worry not, we will guide you through the process! 💪
💡 The Procedural Approach
Before we immerse ourselves in the "R way," let's take a step back and understand the procedural approach presented in the code snippet. The code showcases a traditional for loop iterating over each row in the DataFrame. Within the loop, variables like wellName
and plateName
are assigned values from the current row, and a lookup operation is performed using these values. Finally, the relevant data is appended to an outputFile
using the cat()
function. This approach might seem familiar to those coming from a procedural programming background, but let's discover a more elegant solution in the world of R! 🌍
🔑 The "R Way" - Apply Functions
In R, we strive to write vectorized and efficient code. Instead of explicitly looping over each row, we can utilize the power of apply functions, such as apply()
or lapply()
. These functions allow us to apply a custom function to each row (or column) of a DataFrame, without the need for explicit iteration. 🌟
To illustrate this, let's transform the procedural code snippet to the "R way" by leveraging the apply()
function:
df <- data.frame(name = c("H1", "H2", "H3"), plate = c("plate67", "plate68", "plate69"), value1 = c(10, 20, 30), value2 = c(40, 50, 60))
getWellID <- function(name, plate) {
# Perform the complex lookup operation here
return(paste(name, plate, sep = "_"))
}
outputFile <- "output.txt"
processRow <- function(row) {
wellName <- row["name"]
plateName <- row["plate"]
wellID <- getWellID(wellName, plateName)
dataToAppend <- c(wellID, row["value1"], row["value2"])
cat(paste(dataToAppend, collapse = ","), file = outputFile, append = TRUE, sep = "\n")
}
apply(df, 1, processRow) # Apply processRow() function to each row (1 = rows, 2 = columns)
In this revised version, we create a custom processRow()
function that takes a row as an argument. Inside the function, we perform the complex lookup using the row's data and generate the required data for appending. Finally, we use the cat()
function to append the processed data to the outputFile
.
By calling apply(df, 1, processRow)
, we achieve the desired outcome without explicit looping. The 1
indicates that we want to apply the function to each row of the DataFrame df
. 🎉
📢 Time for Engagement - Your Turn!
Now that you have learned the "R way" to iterate over rows in a DataFrame, it's your turn to try it out! Think about your specific use case and unleash the power of apply functions in R. Share your experience in the comments below, and if you face any challenges or have questions, don't hesitate to reach out. Let's grow together as an R community! 🌱
🤖 Conclusion
In this blog post, we dived deep into iterating over each row in an R DataFrame, transforming a procedural approach to the elegant "R way." By using apply functions, we unleashed the power of vectorized operations, making our code more efficient and concise. Remember, when working in R, it's essential to embrace the language's unique features to maximize productivity and maintainability. So go ahead, refactor those loops, and join the ranks of the R aficionados!
🚀📝👩💻 Keep coding, keep exploring, and keep embracing the beauty of R! Cheers! 🥂🎉