Extract a dplyr tbl column as a vector
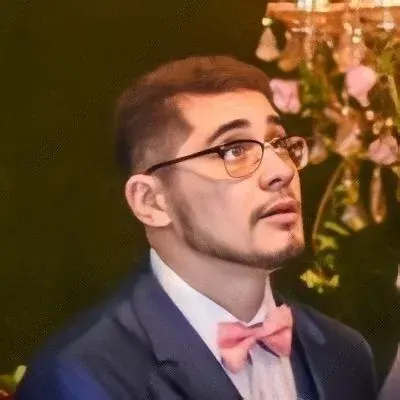
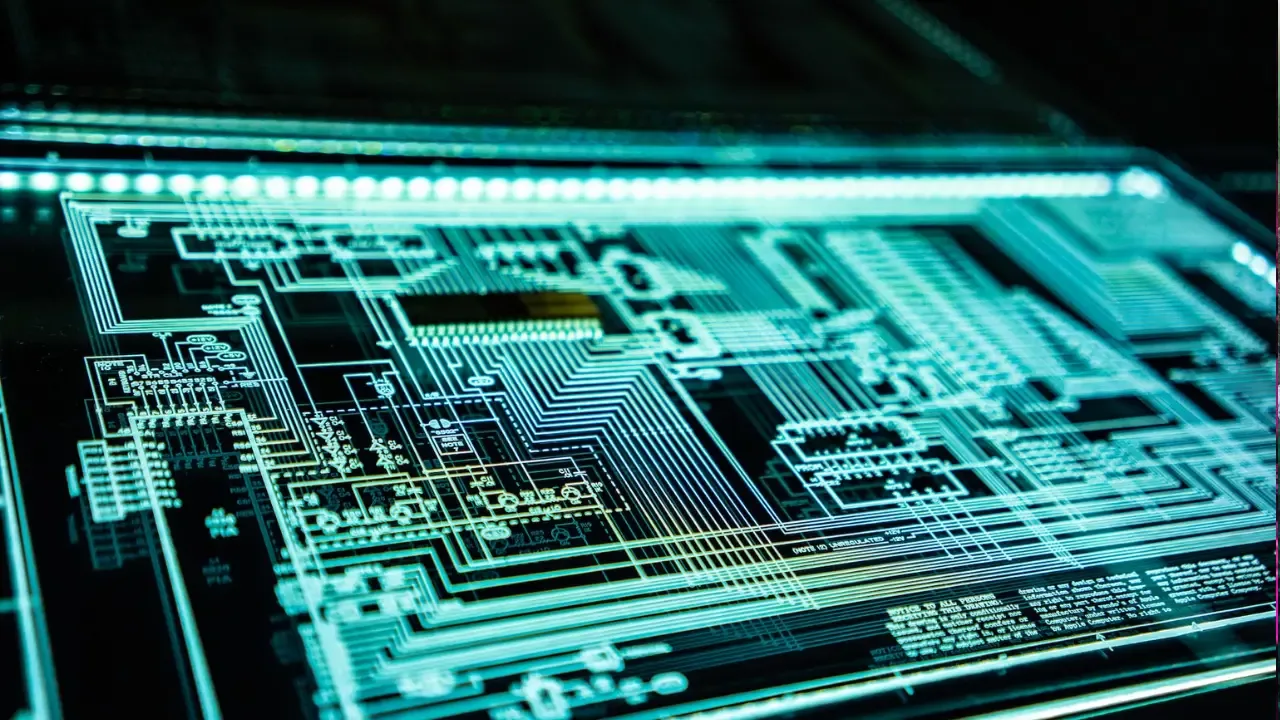
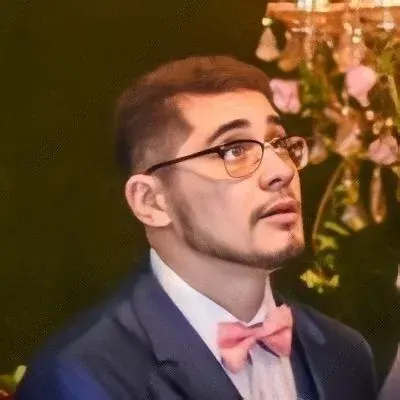
📝 Extract a dplyr tbl column as a vector: Easy solutions for a common problem
Are you frustrated with extracting a specific column as a vector from a dplyr tbl with a database backend? 🤔 Don't worry, we've got you covered! In this blog post, we'll walk you through the common issues surrounding this problem and provide easy solutions to make your life easier. 💪
The Background Story
Let's start with a scenario. You have a dplyr tbl with a database backend, and you want to extract just one column as a vector. Seems like a simple task, right? 🤷♂️ Sadly, it's not that straightforward!
Let's take a look at a piece of code that demonstrates the problem:
require(dplyr)
db <- src_sqlite(tempfile(), create = TRUE)
iris2 <- copy_to(db, iris)
iris2$Species
# NULL
In this case, you might expect to see the Species
column, but it returns NULL
. So, what went wrong? Let's find out!
The Clumsy Solution
One way to extract the column is to use the select()
and collect()
functions, like this:
collect(select(iris2, Species))[, 1]
# [1] "setosa" "setosa" "setosa" "setosa" etc.
This solution works, but the syntax may seem a bit clumsy and convoluted. 😕
The Easy Solutions
Fortunately, there are more succinct ways to achieve the same result. Let's explore two easy and efficient solutions for extracting a dplyr tbl column as a vector.
Solution 1: Use pull()
The pull()
function from the dplyr package is designed specifically for extracting a single column as a vector. Here's how you can use it:
pull(iris2, Species)
# [1] "setosa" "setosa" "setosa" "setosa" etc.
That's it! 🎉 With just one line of code, you can extract the desired column as a vector using pull()
.
Solution 2: Convert to a data frame and extract the column
Another simple solution is to convert the tbl to a data frame and then extract the column as you would normally do. Here's how you can do it:
as.data.frame(iris2)$Species
# [1] "setosa" "setosa" "setosa" "setosa" etc.
By converting the tbl to a data frame, you can access the column using the familiar $
operator effortlessly. ✨
The Call-to-Action
Now that you know the easy solutions to extract a dplyr tbl column as a vector, it's time to put your knowledge into practice and simplify your data extraction tasks! 🚀
Try out these solutions in your own code and share your experience in the comments below. We would love to hear from you! 💬
The Conclusion
Extracting a single column as a vector from a dplyr tbl with a database backend can be a bit tricky, but it doesn't have to be a headache. By using the pull()
function or converting the tbl to a data frame, you can achieve your goal easily and efficiently.
Remember, ease of use and simplicity are key when working with data. So, next time you encounter this problem, don't stress! Just refer back to this guide for quick and simple solutions. 😎
Happy coding! 💻✨