Determine the data types of a data frame"s columns
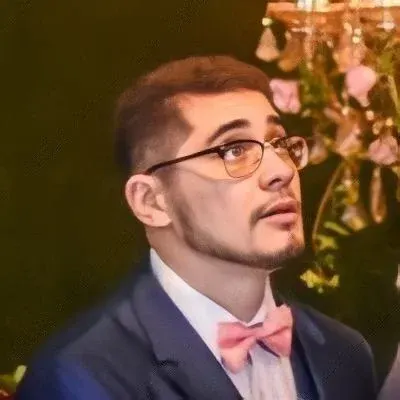
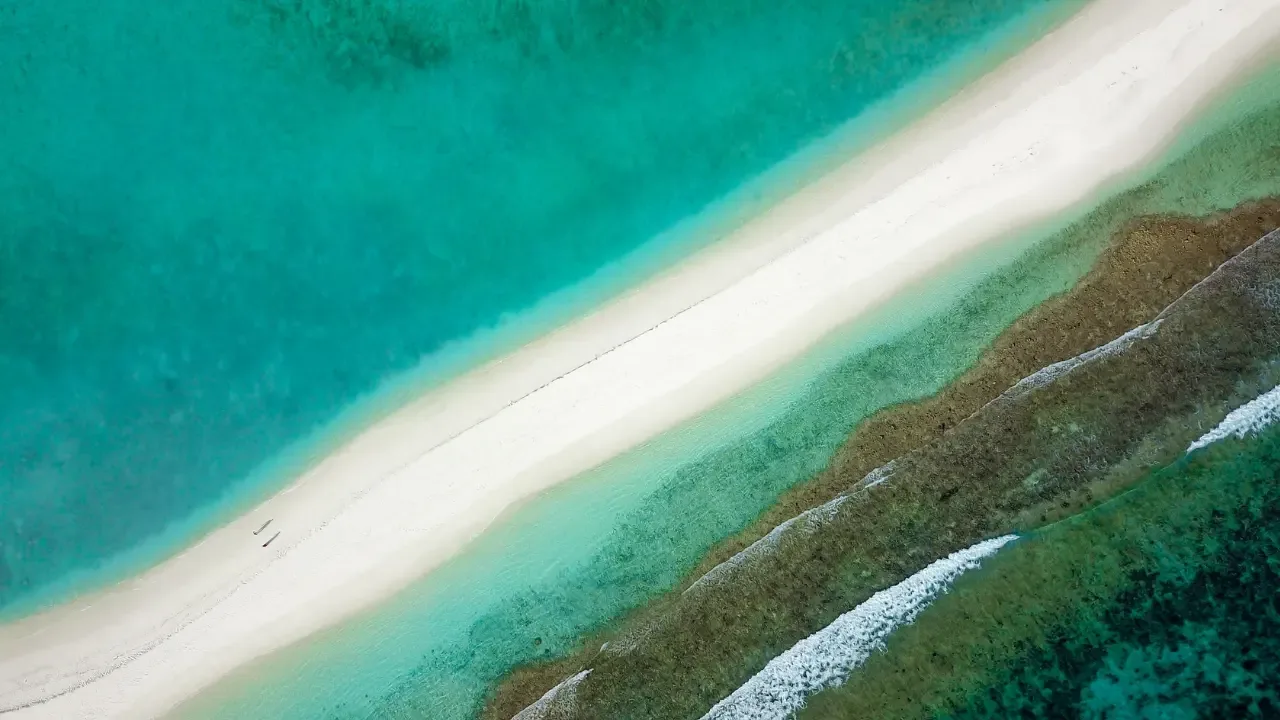
📊 Determining the Data Types of a Data Frame's Columns in R
So, you've loaded your data into a dataframe in R using the read.csv()
function. Now you want to know how to determine the data type of each column in that data frame. Don't worry, I'm here to help you unravel this mystery! 😄
The Problem: Understanding Data Types in a Data Frame
Data frames in R are incredibly useful for organizing and analyzing data. However, it's essential to know the data types of your columns because it affects how you can manipulate and work with your data. The wrong data types can lead to unexpected results and errors in your analyses. 😱
The Solution: Inspecting Data Types
Lucky for us, R provides a straightforward method for determining the data types of a data frame's columns. We can use the str()
function to gain insights into the structure of our data frame. Let's take a look at an example:
# Load the data frame
my_data <- read.csv("your_data_file.csv")
# Inspect the data types
str(my_data)
Running the str()
function on your data frame will display a summary of its structure. It includes information such as the variable names, data types, and the first few values in each column. 🧐
Example: Determining Data Types
To make things clearer, let's consider a scenario where we have a data frame called "my_data" with three columns:
# Sample data frame
my_data <- data.frame(
name = c("John", "Jane", "Alice"),
age = c(25, 32, 28),
is_employed = c(TRUE, FALSE, TRUE)
)
If we run str(my_data)
on our sample data frame, we would get the following output:
'data.frame': 3 obs. of 3 variables:
$ name : Factor w/ 3 levels "Alice","Jane",..: 3 2 1
$ age : num 25 32 28
$ is_employed: logi TRUE FALSE TRUE
From the output, we can deduce that:
The "name" column is a factor (a categorical variable) with three levels: "Alice", "Jane", and "John".
The "age" column is a numeric variable, indicated by "num".
The "is_employed" column is a logical variable (boolean), labeled as "logi" and representing TRUE or FALSE values.
Your Turn: Discover the Data Types!
Now it's your turn to put this knowledge into practice! Load your own data frame or use the provided example data frame to determine the data types of your columns. Run the str()
function on your data frame and take a look at the output. 🕵️
If any of the data types are unexpected or seem incorrect, it may be due to the way the data was read and imported. Ensure that you're using the correct functions, such as read.csv()
for CSV files or other appropriate functions for different file formats.
Conclusion
Understanding the data types of each column in your data frame is crucial for effective data analysis in R. By using the str()
function, you can quickly inspect the structure of your data frame and make informed decisions about how to manipulate your data.
So, don't hesitate to take a peek at the data types – it's an essential step towards mastering R and extracting valuable insights from your data! 📚🔍
👉 What are the most surprising or unexpected data types you've encountered in your data frames? Share your thoughts and experiences in the comments below! Let's explore this together!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
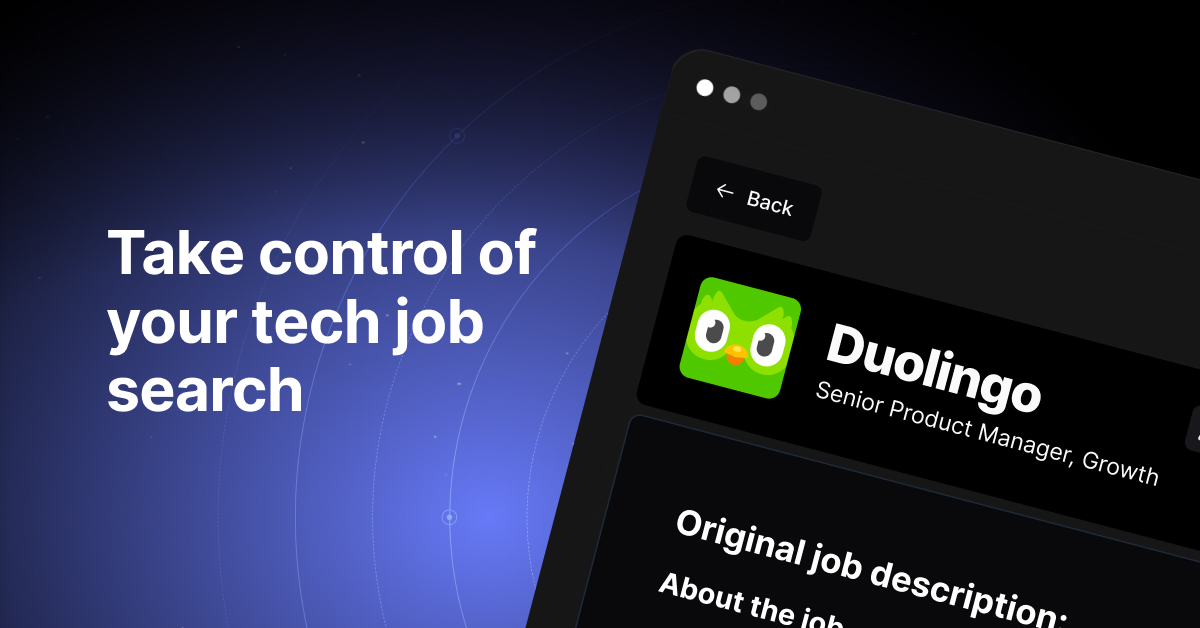