"Correct" way to specifiy optional arguments in R functions
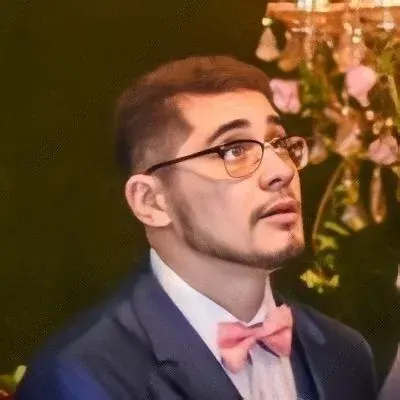
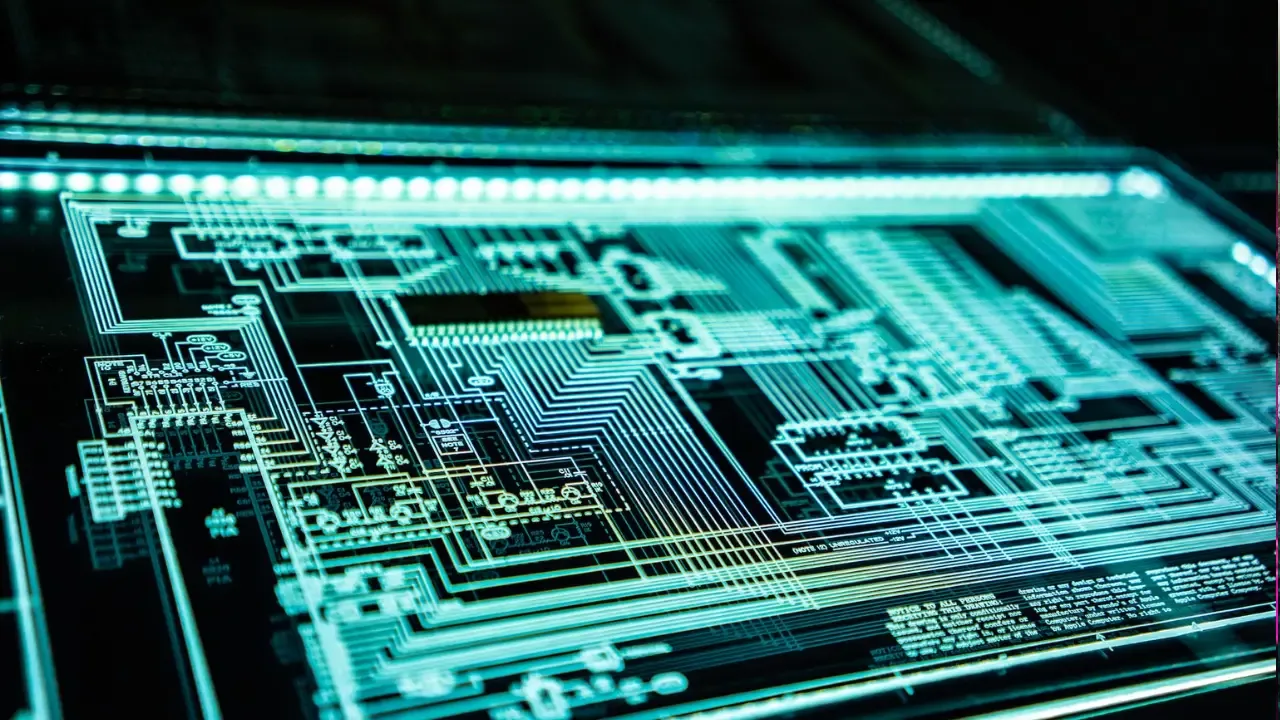
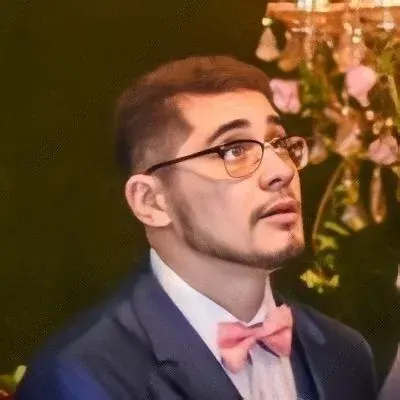
📝 Title: The "Correct" Way to Specify Optional Arguments in R Functions: A Guide
Are you confused about the "correct" way to write functions with optional arguments in R? You're not alone! In this blog post, we'll dive into the common issues and provide easy solutions to help you write clean and error-free code. Buckle up, and let's uncover the secrets of specifying optional arguments in R functions! 😎
💡 Introduction: The Dilemma
Sometimes, when writing R functions, we need to make certain arguments optional. However, there's no official consensus on the best approach. In this guide, we'll explore two common methods and discuss their pros and cons. By the end, you'll have a clear understanding of how to choose the most suitable option for your coding needs.
🙋♀️ Method 1: The "is.null" Approach
The first approach involves using a default value, usually NULL
, for the optional argument. Let's take a look at an example:
fooBar <- function(x, y = NULL) {
if (!is.null(y))
x <- x + y
return(x)
}
In this snippet, x
is always required, while y
is optional and defaults to NULL
. We can invoke the function in two ways:
fooBar(3) # Returns 3
fooBar(3, 1.5) # Returns 4.5
🔍 Explanation: Method 1
When y
is not NULL
, the function adds x
and y
. This method is straightforward and less error-prone. It's a great choice when dealing with a small number of optional arguments. However, what if you want to handle an arbitrary number of optionals? Fear not—we have another method to explore!
🚀 Method 2: The "..." Approach
The second option lets you pass an arbitrary number of optional arguments using the ...
syntax. Let's see it in action:
fooBar <- function(x, ...) {
z <- list(...)
if (!is.null(z$y))
x <- x + z$y
return(x)
}
In this variation, we define a list z
to capture the optional arguments. The y
argument can be specified by name, such as fooBar(3, y = 1.5)
. However, if you prefer, you can use unlist(z)
or z <- sum(...)
instead.
🔍 Explanation: Method 2
This method allows for greater flexibility by incorporating an arbitrary number of optional arguments. If you anticipate a scenario where you need to handle multiple optionals, this method might be the better choice. However, remember that it's slightly more error-prone than Method 1, as the arguments are passed using names or positional ordering.
🛠️ Choosing the "Correct" Way: Personal Preference and Beyond
Selecting the "correct" method boils down to personal preference and the needs of your specific use case. Both methods have their pros and cons. Method 1 is cleaner and less prone to errors, making it suitable for a small number of optionals. On the other hand, Method 2 is more flexible, allowing for an arbitrary number of optionals. Consider your requirements, the complexity of your code, and the potential for future scalability.
💬 Call-to-Action: Share Your Approach!
What's your preferred method for specifying optional arguments in R functions? Are you Team Method 1 or Team Method 2? We'd love to hear your thoughts and experiences in the comments below. Let's spark a discussion and learn from each other!
So, whether you're a beginner or an experienced R coder, don't let the uncertainty of optional arguments hold you back. Embrace these methods, experiment, and find what works best for you. Happy coding! 🚀💻