Append value to empty vector in R?
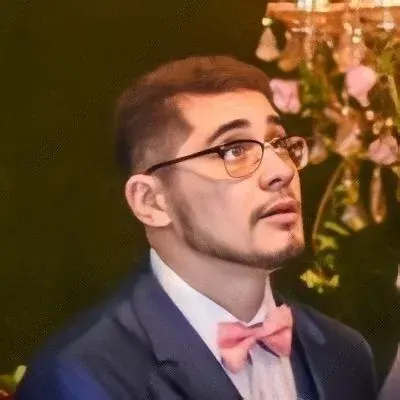
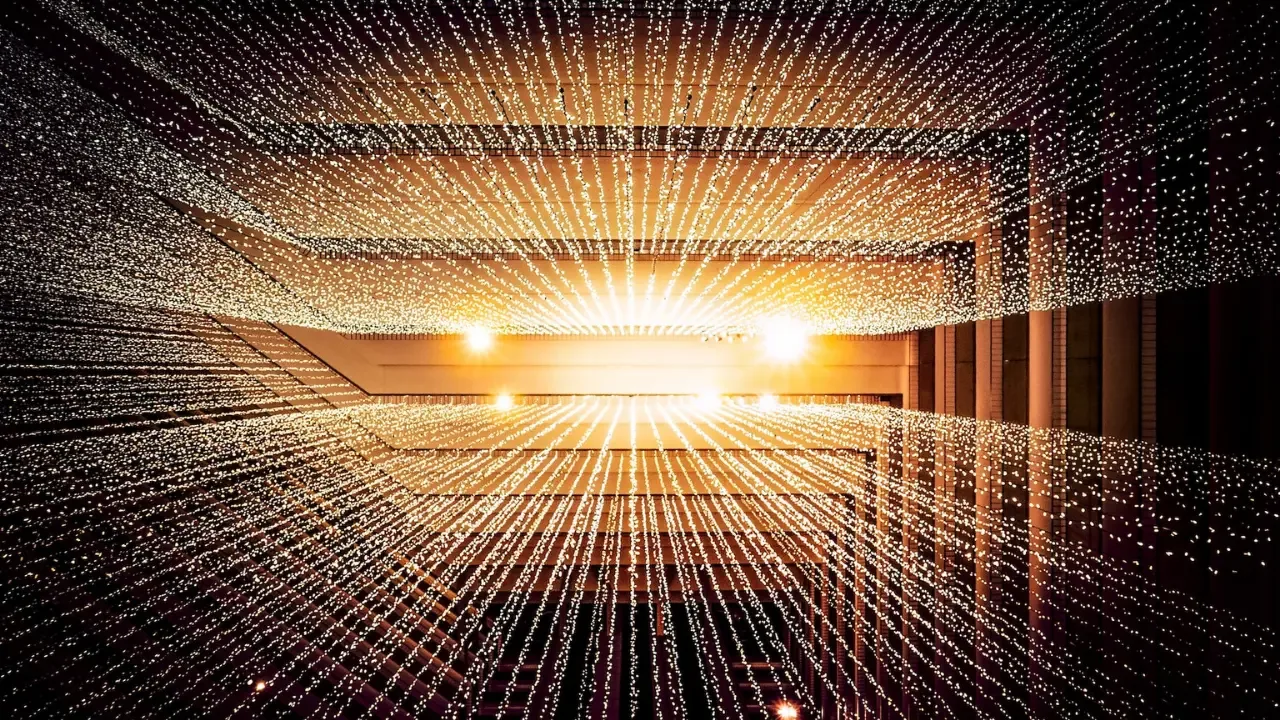
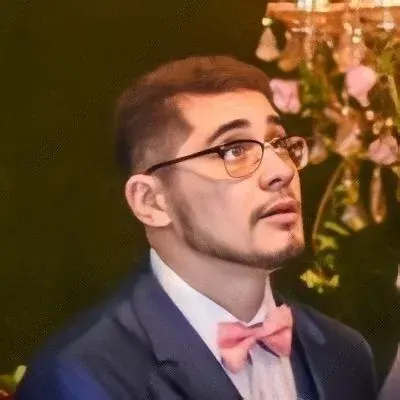
📚 A Complete Guide on Appending Values to an Empty Vector in R
Are you new to R programming and struggling to figure out how to append values to an empty vector? Don't worry, we've got you covered! In this blog post, we will walk you through common issues and provide easy solutions to help you append values to an empty vector in R. 🙌
Understanding the Problem
The problem at hand is appending values to an empty vector in R. In other programming languages like Python, appending values to a list is straightforward. However, the syntax and approach in R differ slightly. So, let's dive into the solution!
Solution Approach
To append values to an empty vector in R, you can use a for loop and the length()
function to iterate over the values and add them to the vector.
Here's an example code snippet to illustrate this approach: 🖥️
vector <- c()
values <- c('a', 'b', 'c', 'd', 'e', 'f', 'g')
for (i in 1:length(values)) {
vector <- c(vector, values[i])
}
In the above code, we:
Initialize an empty vector using
c()
and assign it to the variablevector
.Define the values that we want to append to the vector, in this case,
values
with elements 'a' to 'g'.Use a for loop to iterate over each element in
values
.Inside the loop, we append each element (
values[i]
) to the empty vectorvector
by using the concatenation operatorc()
.Finally, we have our desired vector with appended values.
That's it! You have successfully appended values to an empty vector in R. 🎉
A More Efficient Alternative 🔍
While the above approach works perfectly fine, there is a more efficient way to achieve the same result in R, using the append()
function.
Here's an example of how to use the append()
function to append values to an empty vector: 🚀
vector <- c()
values <- c('a', 'b', 'c', 'd', 'e', 'f', 'g')
vector <- append(vector, values)
In the code above, we:
Initialize an empty vector using
c()
and assign it to the variablevector
.Define the values that we want to append to the vector as before, in this case,
values
with elements 'a' to 'g'.Use the
append()
function, which takes the empty vector (vector
) and the values you want to append (values
) as arguments.Assign the result back to
vector
, which now contains the appended values.
This alternative method using append()
is both concise and efficient, making your code more readable and maintainable. 🎯
Conclusion ✨
Appending values to an empty vector in R may initially seem confusing, but with the solutions provided above, you're well-equipped to handle this task. Remember to choose the approach that suits your needs best: the traditional for loop or the more efficient append()
function.
Now it's your turn to give it a try! 🤓
If you have any questions or face any issues, feel free to reach out in the comments below. We'd love to hear from you and help you out. Happy coding! 💻
Like this article? Share it with your fellow R enthusiasts! 💬