Append an object to a list in R in amortized constant time, O(1)?
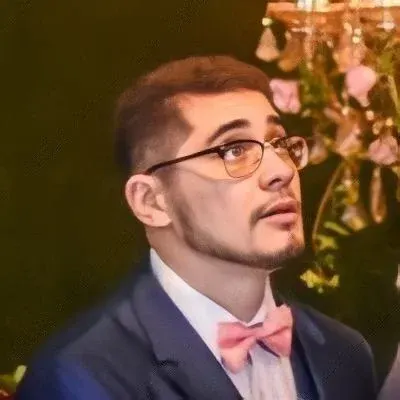
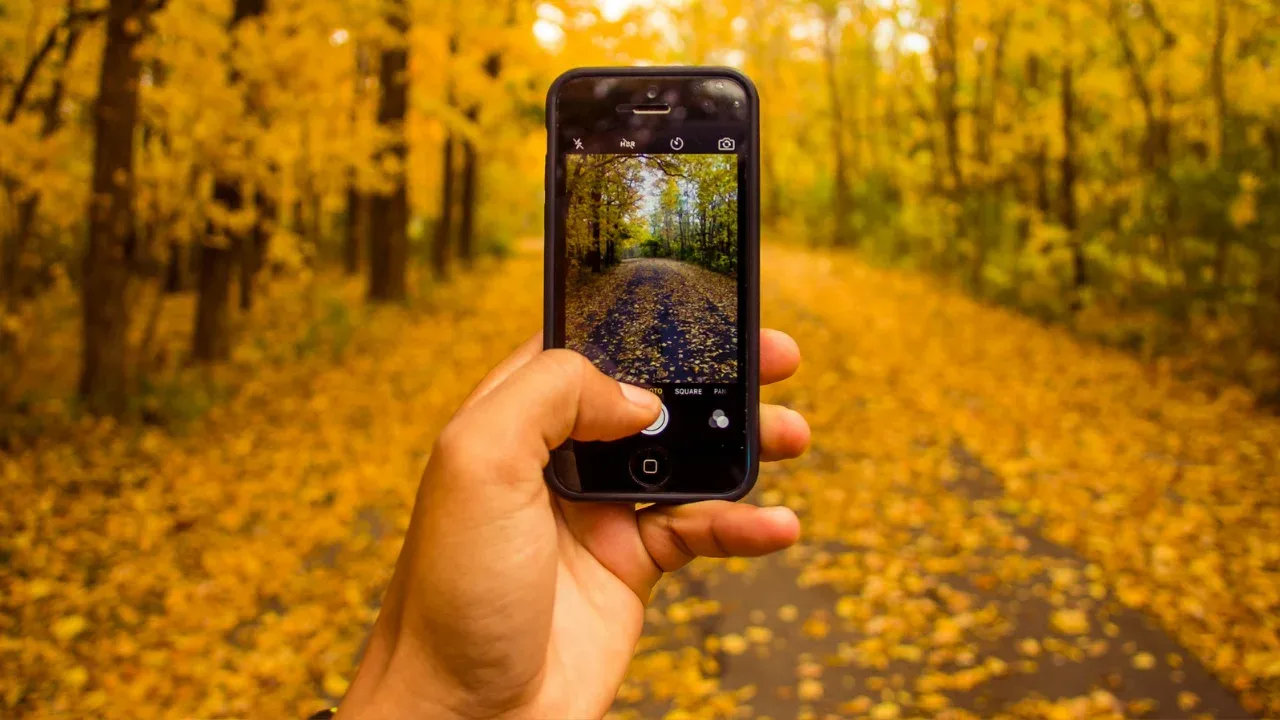
📝 Amortized Constant Time Appending to a List in R
Are you tired of using complex methods to append an object to a list in R? 🤔 Don't worry, I've got you covered! In this blog post, I'll guide you through the common issues and provide easy solutions to help you append an object to a list in amortized constant time, O(1). Let's dive in! 💪
The Usual Approach
So, the regular way to append an object to a list in R is by using the following code:
mylist[[length(mylist)+1]] <- obj
While this method works perfectly fine, it doesn't provide the elegance and simplicity we desire. We yearn for a more compact and beautiful solution. 🌟
The Failed Attempt with lappend()
When trying to enhance the append functionality, you might have come across the lappend()
function. It goes something like this:
lappend <- function(lst, obj) {
lst[[length(lst)+1]] <- obj
return(lst)
}
But unfortunately, due to R's call-by-name semantics, this approach doesn't work as expected. Changes made to lst
inside lappend()
are not visible outside the function scope. 😞
A More Elegant Solution
But fear not! There's a more beautiful way to append an object to a list in R. 🎉 This approach not only solves the previous issue but also works for both vectors and lists. Here's the magic code:
mylist <- c(mylist, obj)
By using the c()
function, we can concatenate the existing list mylist
with the new object obj
. This creates a new list that includes the appended item. It's as simple as that! 😎
Your Turn to Shine!
Now that you know the secret, it's time for you to give it a try. Experiment with different lists and objects. Play around and see how effortlessly you can append items in R! ✨
I encourage you to share your experiences and thoughts in the comments section below. If you have any questions or other cool tricks to share, feel free to join the conversation. Let's make R programming even more exciting and efficient together! 🚀
Happy coding! 💻
What other R-related topics would you like me to cover in future blog posts? Let me know in the comments!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
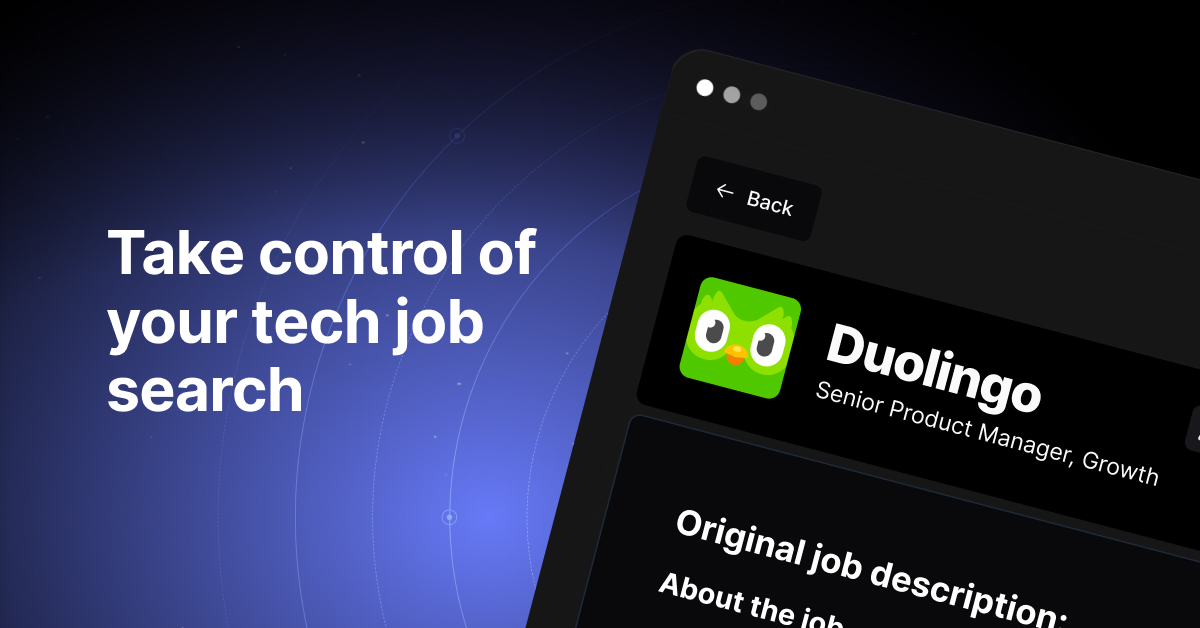