Why is "x" in ("x",) faster than "x" == "x"?
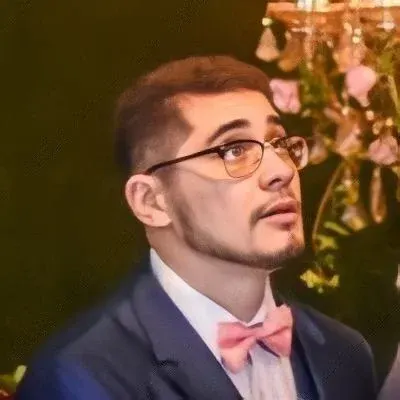
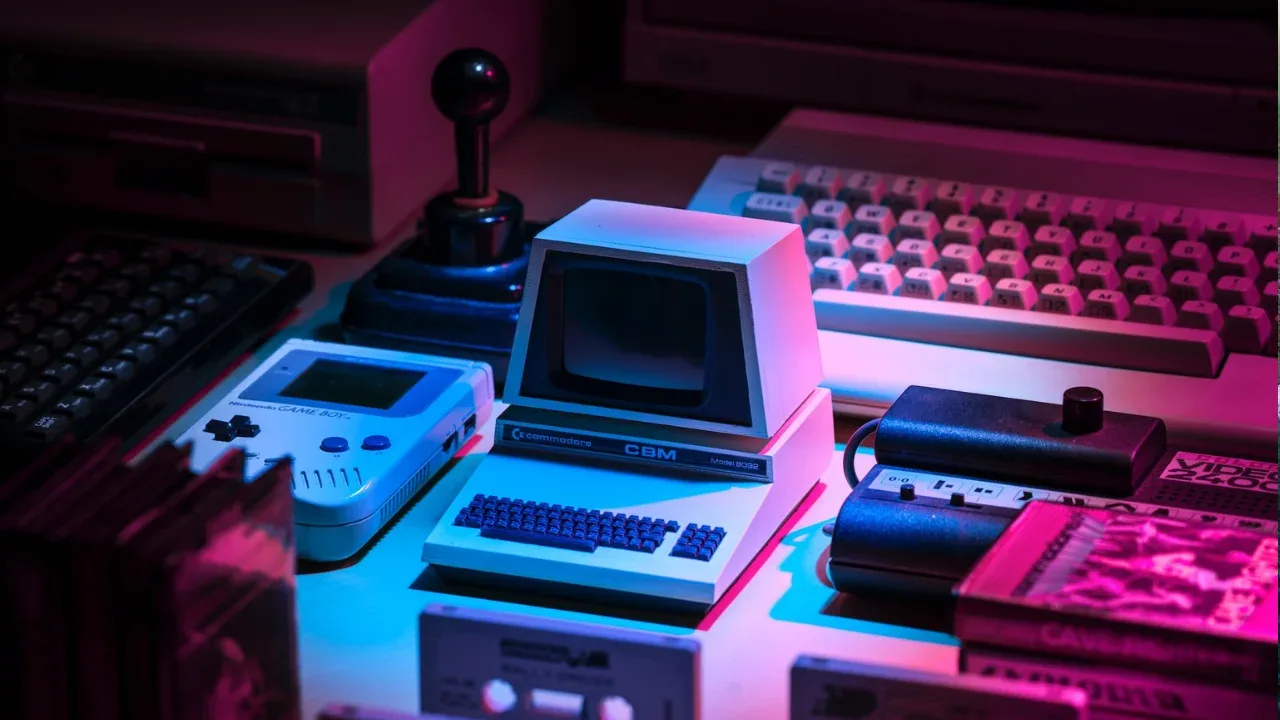
Why is 'x' in ('x',) faster than 'x' == 'x'?
Hey there! 👋 Have you ever wondered why using the in
operator seems to be faster than the ==
operator in certain cases? Well, today we're going to dig deep into this question and unravel the mystery for you! 🕵️♂️
Let's start by looking at some code examples:
>>> timeit.timeit("'x' in ('x',)")
0.04869917374131205
>>> timeit.timeit("'x' == 'x'")
0.06144205736110564
Here, we can see that using the in
operator to check if the string 'x'
is present in the tuple ('x',)
is faster than using the ==
operator to compare the string 'x'
with itself.
But why does this happen? 🤔
To understand the reason behind this performance difference, we need to dive into how the two operators work under the hood.
The in
operator checks if an element exists in an iterable (in this case, a tuple), while the ==
operator compares two objects for equality.
When using the in
operator, Python performs a membership test by iterating over the elements in the specified iterable until a match is found. If the element is found early on, the process stops, resulting in faster performance.
On the other hand, the ==
operator compares both sides of the expression character by character, making it slightly slower for simple comparisons like the one mentioned above.
Let's explore this further with additional examples:
>>> timeit.timeit("'x' in ('x', 'y')")
0.04866674801541748
>>> timeit.timeit("'x' == 'x' or 'x' == 'y'")
0.06565782838087131
>>> timeit.timeit("'x' in ('y', 'x')")
0.08975995576448526
>>> timeit.timeit("'x' == 'y' or 'x' == 'y'")
0.12992391047427532
As you can see, even when dealing with tuples containing multiple elements, the in
operator consistently outperforms the ==
operator. The more elements you have, the greater the performance gap becomes.
So, what should we take away from this? 🤷♀️
Based on these observations, it's clear that using the in
operator is generally faster and more efficient when checking for membership. Therefore, if you're using Python and need to determine whether an element exists in an iterable, go ahead and use the in
operator with confidence! 😎
Now that you're armed with this knowledge, go ahead and optimize your code by replacing unnecessary ==
operations with the more efficient in
operator. Your code will not only run faster but also be more readable and concise!
If you found this blog post helpful, don't forget to share it with your fellow Pythonistas! 🚀
Got any other questions or insights? Leave a comment below and let's keep the conversation going! 💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
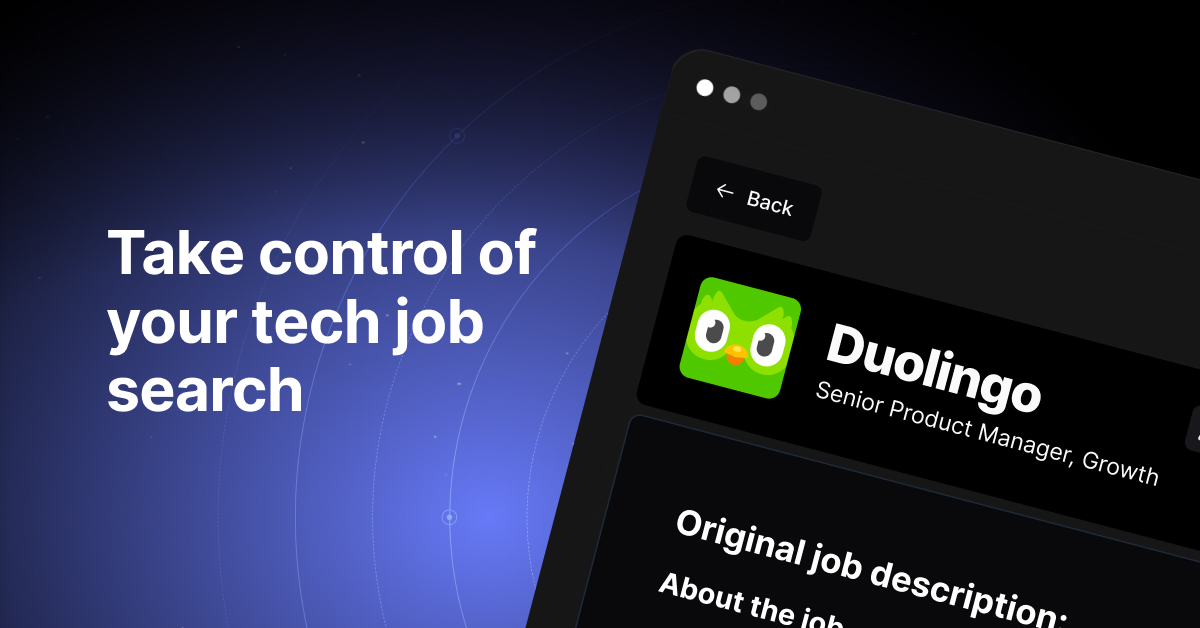