Why is there no xrange function in Python3?
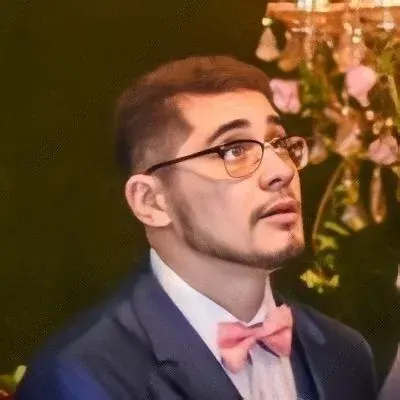
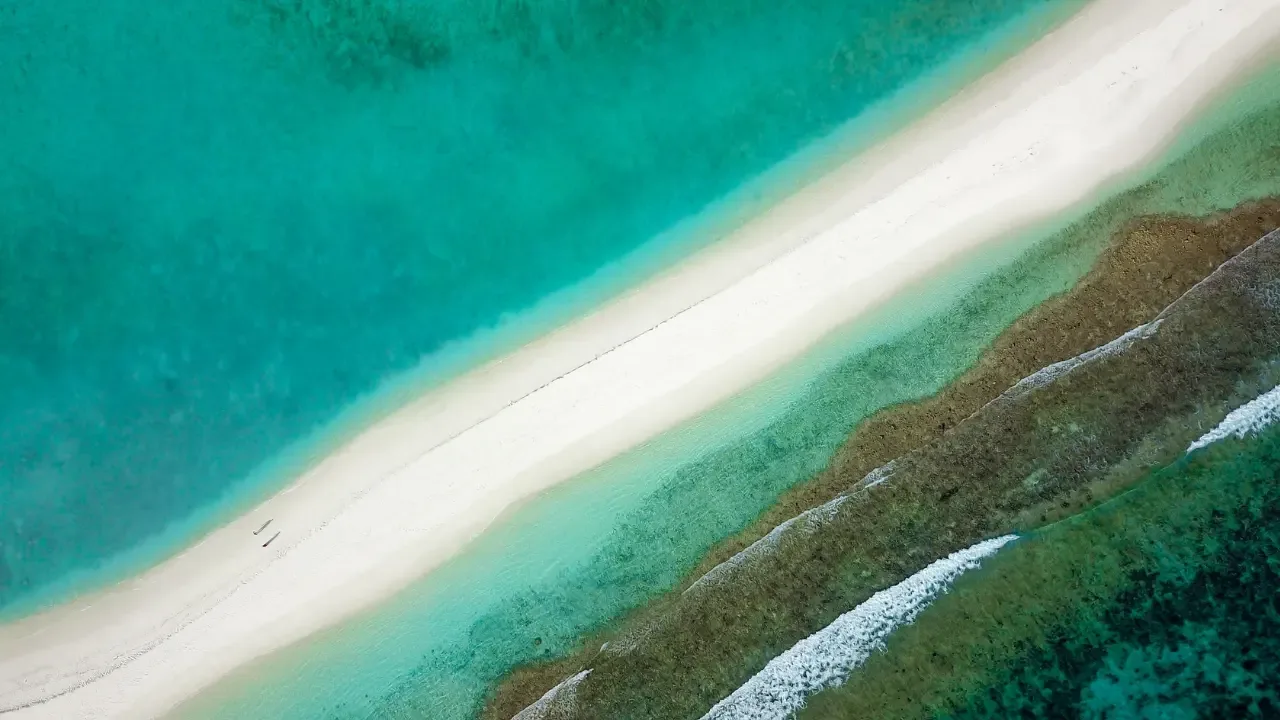
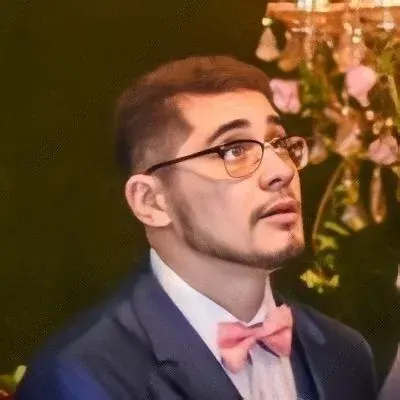
📢 Why is there no xrange function in Python3? Let's unravel this mystery and find easy solutions, so you can code like a pro! 🐍
Recently, I dived into Python3 and discovered a missing piece in the puzzle - the beloved xrange
function. 😢 It used to be a handy tool in Python2, but it has vanished in Python3. So, what's the deal?
To shed some light on this matter, let's examine a simple example comparing Python2 and Python3:
Python2:
from time import time as t
def count():
st = t()
[x for x in xrange(10000000) if x%4 == 0]
et = t()
print et-st
count()
Python3:
from time import time as t
def xrange(x):
return iter(range(x))
def count():
st = t()
[x for x in xrange(10000000) if x%4 == 0]
et = t()
print (et-st)
count()
Running these code snippets will yield the following results:
Python2:
1.53888392448
Python3:
3.215819835662842
Wow, that's quite a performance difference! But why? Why was xrange
removed? And where can we find more information? Let's dive in! 💡
🔎 The reason behind the removal of xrange
lies in the Python3 philosophy of optimizing memory usage. In Python2, xrange
returned a generator that produced values on the fly, optimizing both time and space. However, in Python3, the range
function was enhanced to behave like xrange
- returning an iterator instead of a list.
To bridge the gap, you can create your own equivalent xrange
function in Python3 using the iter
and range
functions - like the example provided. This way, you can still enjoy the memory efficiency of generators while coding in Python3. 🎉
But what if you miss the concise and elegant syntax of xrange
in Python2? Fear not! You can simulate it using a list comprehension or a for loop:
Using list comprehension:
[x for x in range(10000000) if x%4 == 0]
Using a for loop:
result = []
for x in range(10000000):
if x%4 == 0:
result.append(x)
Both of these alternatives achieve the same result as the original xrange
function, ensuring you don't miss out on any Pythonic goodness. 😄
📚 If you want to dive deeper into the reasons behind the removal of xrange
and other changes in Python3, you can refer to the Python Enhancement Proposal (PEP) 3100. It explains the decision-making process and the motivation behind various modifications in Python3.
Now that you understand the absence of xrange
in Python3 and have easy alternatives, it's time to embrace the change and level up your Python skills! ⚡️
Did you find this post helpful? Share your thoughts in the comments below and let's keep the conversation going. 💬 And don't forget to spread the Pythonic love by sharing this post with your fellow developers! 🚀