Why is it string.join(list) instead of list.join(string)?
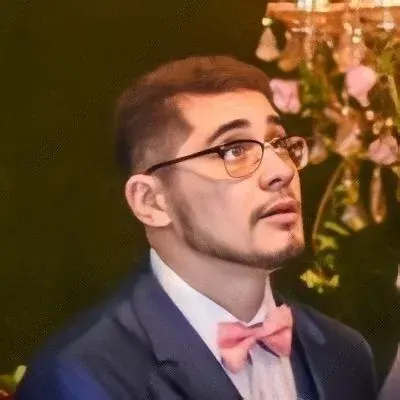
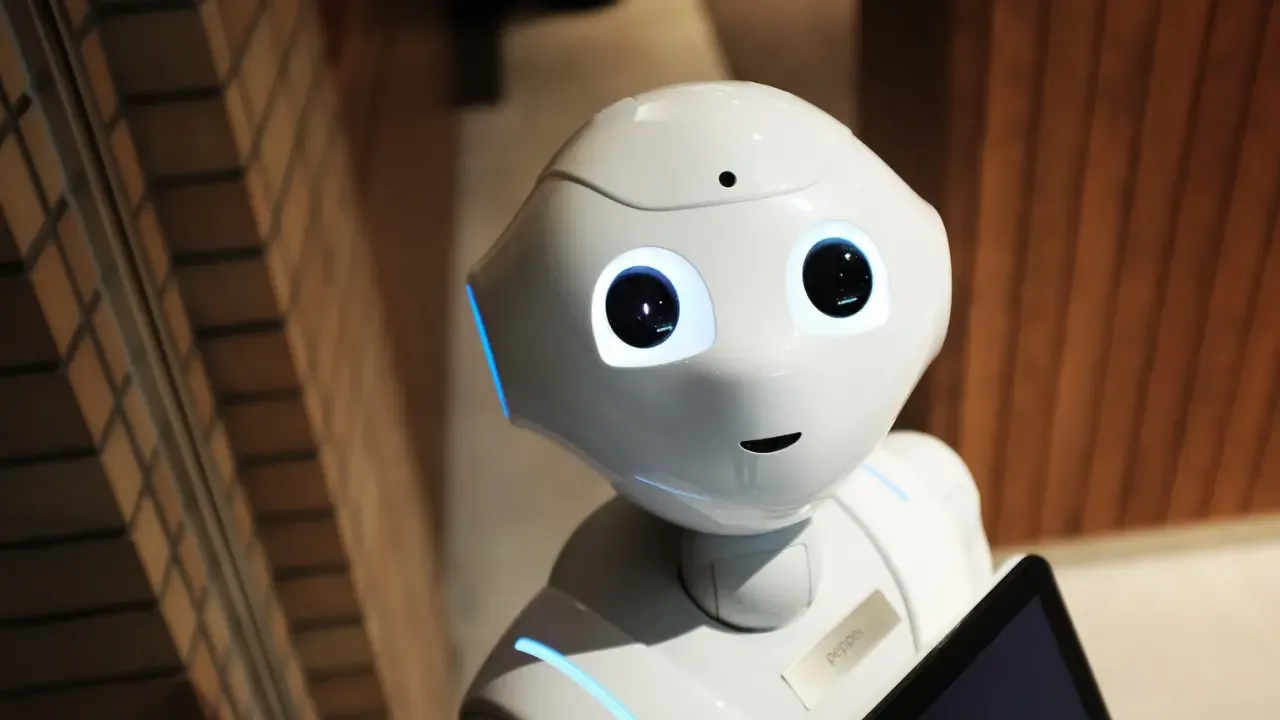
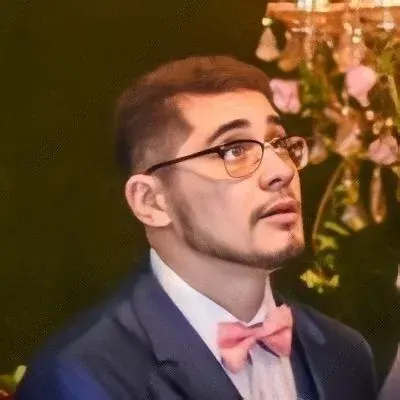
String.Join(List) vs. List.Join(String): The Battle of Word Concatenation
š Welcome back to our tech blog! Today we are going to dive deeper into a question that has puzzled many: why is it string.join(list)
instead of list.join(string)
? š¤ This seemingly innocent query has sparked confusion and frustration among programmers, but fear not! We have the answers you seek.
The Confusion Unveiled
Before we delve into the depths of this conundrum, let's take a quick look at the provided code snippets to understand the heart of the issue.
["Hello", "world"].join("-")
Versus
"-".join(["Hello", "world"])
At first glance, one might argue that list.join(string)
reads more naturally and feels intuitive. So why, oh why, does the opposite prevail? š¤
Understanding the Syntax
To decipher this puzzle, we need to understand how these methods are structured. In Python, string.join(list)
is the correct syntax for word concatenation. Here's why:
Method Invocation
In Python, methods are invoked on objects. In the case of string.join(list)
, the join
method is invoked on the string object. On the other hand, list.join(string)
would imply invoking the join
method on the list object. But does the list have a join
method? š¤ Unfortunately, it does not.
Consistency is Key
Programming languages strive for a consistent and uniform design across various methods and functionalities. Python's choice of string.join(list)
aligns with this principle.
By placing the join
method within the string
object, it establishes a logical and predictable pattern. Similar methods like split
, replace
, and format
also follow this paradigm. It ensures that regardless of the task at hand, developers can anticipate where to find these methods with ease. šÆ
š ļø A Solution at Hand
Now that we've shed light on the "why" behind this syntax, it's time to address the problem at hand: how to concatenate words effectively using a delimiter. Fear not, for we have some practical solutions for you!
Solution 1: The Traditional Approach
The first solution embraces the Pythonic way by using the familiar string.join(list)
syntax. Let's see it in action:
delimiter = "-"
words = ["Hello", "world"]
result = delimiter.join(words)
print(result) # Output: Hello-world
By invoking the join
method on the delimiter string and passing the list of words as the argument, we achieve the desired concatenation with the specified delimiter. š
Solution 2: Embrace the List Comprehension
For those with a penchant for brevity and elegance, fear not! Python offers an alternative solution using list comprehension:
delimiter = "-"
words = ["Hello", "world"]
result = delimiter.join([f"{word}" for word in words])
print(result) # Output: Hello-world
By employing a list comprehension, we iterate through the words in the list, encapsulating each word with the desired delimiter. The join
method then combines these elements into a single string. Voila! š
š” Shine Bright and Share!
We hope this enlightening exploration has quelled your confusion surrounding string.join(list)
versus list.join(string)
. Now it's time for you to shine! Share this blog post with fellow programmers and expand their knowledge too. š„
Remember, understanding the reasoning behind seemingly odd syntax choices can often lead to a profound appreciation for a language's design. So go forth, concatenate with confidence, and embrace the elegance of Python! š
If you've encountered any other perplexing coding questions or have comments to add, drop them in the comments section below. Let's keep the discussion going! š