Why does comparing strings using either "==" or "is" sometimes produce a different result?
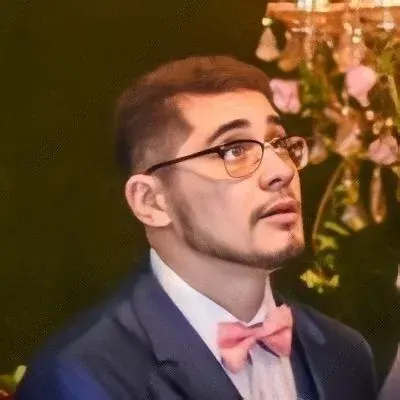
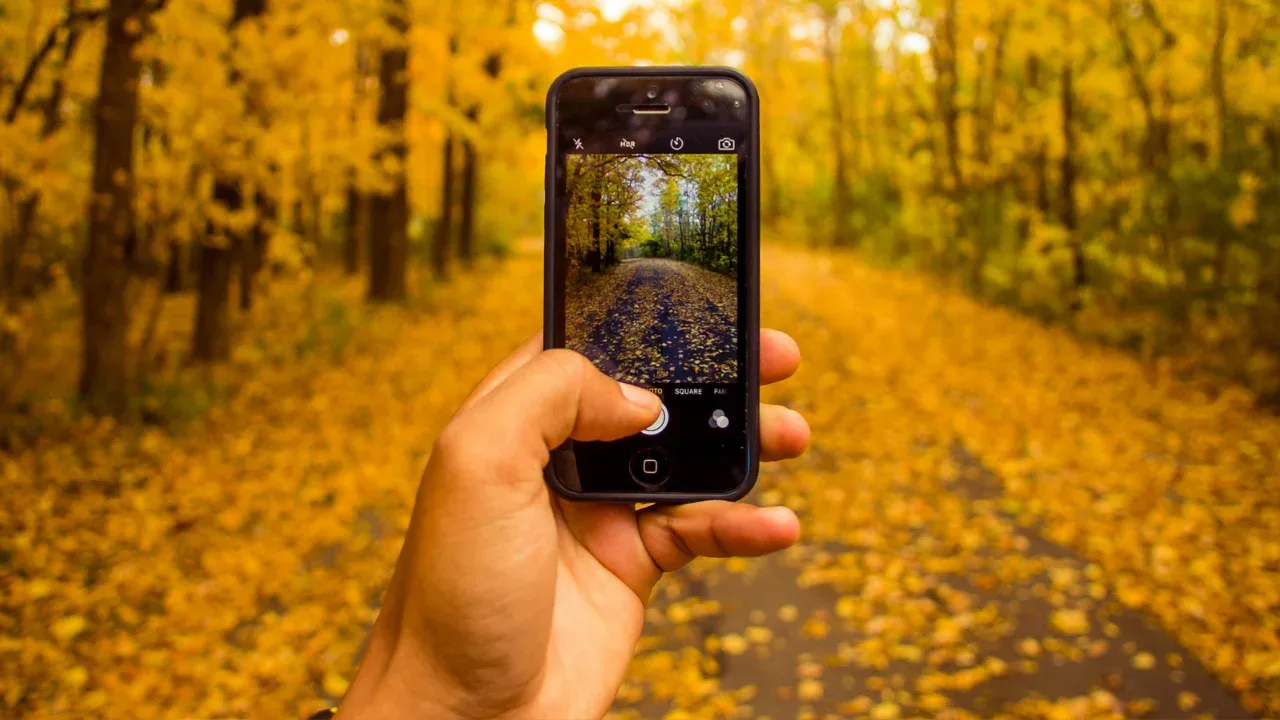
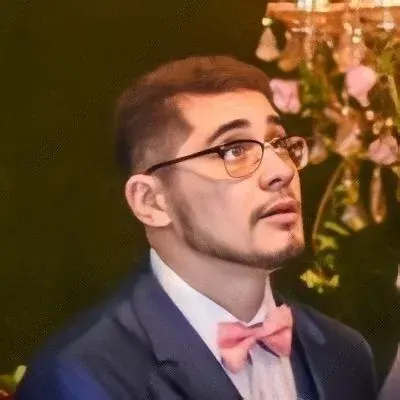
🔍Why does comparing strings using either '==' or 'is' sometimes produce a different result? 🤔
Have you ever encountered a situation where two string variables are set to the same value, but when you compare them using '==' and 'is', you get different results? It can be quite puzzling, and you may wonder why this happens. 🤷♀️
Let's dive into this common issue in Python and explore why comparing strings using '==' or 'is' can sometimes yield different outcomes. 💻
The Difference between '==' and 'is'
Before we delve into the specifics of the problem, let's clarify the difference between '==' and 'is' in Python.
'==' compares the values of two objects, and returns True if they are equal.
'is' compares the identities of two objects, and returns True if they refer to the same object in memory.
The Context: Comparing Strings
Now, let's put this into context using string variables. Consider the following code:
s1 = 'text'
s2 = 'text'
print(s1 == s2) # True
print(s1 is s2) # It depends!
Surprisingly, the result of the '==' comparison is always True. However, when we use 'is' for comparison, things become inconsistent. Sometimes it returns True, while other times it returns False. 😲
The Explanation: String Interning
The reason behind this unexpected behavior lies in a concept called "string interning". In Python, string interning is an optimization technique to store only one instance of a particular string value in memory. This optimization aims to save memory consumption.
When you create a string in Python (e.g., 'text'), the interpreter checks if an identical string already exists in memory. If it does, the interpreter refers to the existing instance instead of creating a new one.
For strings that are created explicitly (like in our example above), the interpreter optimizes memory usage and ensures that the 'is' comparison returns True. However, for strings created implicitly or through runtime computations, the optimization may not occur, leading to the 'is' comparison returning False.
Solutions: What You Can Do
If you encounter this issue and need to compare the values of strings, it is recommended to use the '==' operator. Since '==' compares the values and not the identities, it will consistently return the expected result.
If you specifically need to check if two strings refer to the same object in memory (same identity), you can use the 'is' operator. However, be cautious when using 'is' with implicitly created strings or dynamically computed string values.
Call-to-Action: Share Your Experience!
Have you ever encountered this issue while comparing strings? Share your experience and let us know in the comments below! 👇
Understanding the distinction between '==' and 'is' when comparing strings can help you avoid unexpected pitfalls in your Python code. Remember to choose the appropriate operator based on your specific requirements.
If you found this post helpful, share it with your fellow Pythonistas! 🐍💻
Happy Coding! 🎉✨