Why do Python classes inherit object?
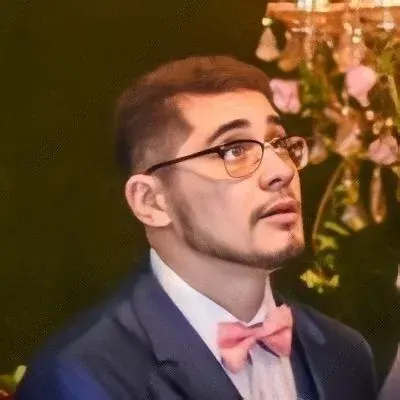
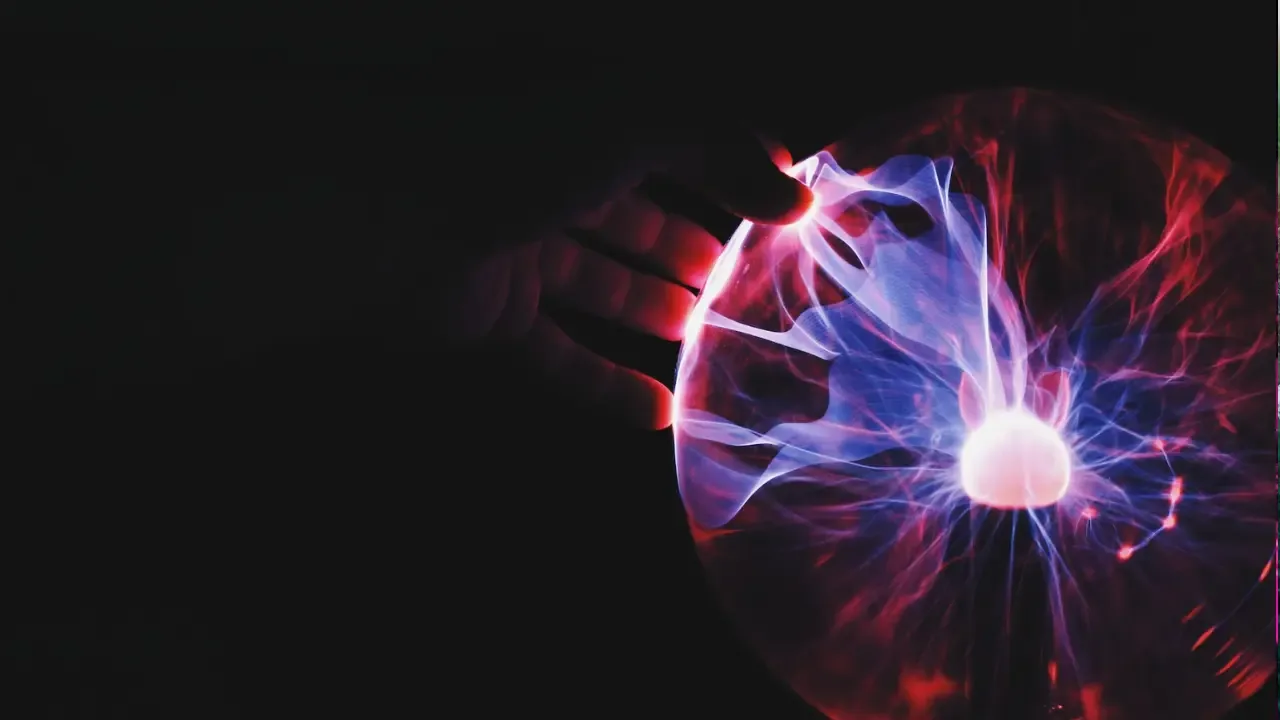
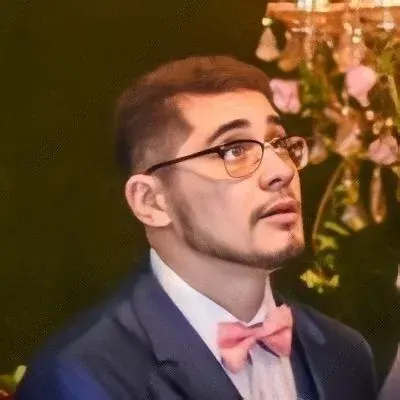
💡Why do Python classes inherit object
?
If you've ever come across the following class declaration in Python:
class MyClass(object):
...
You might have wondered, why does the class inherit from object
? After all, isn't it enough to simply declare a class without any explicit inheritance?
In this article, we'll delve into the rationale behind inheriting from object
and address common issues that arise from omitting or misunderstanding this inheritance. By the end, you'll have a solid understanding of why it is important and how it can impact your code.
🤷♂️ Why is object
inheritance necessary?
In Python, every class implicitly inherits from the built-in object
class. This inheritance provides the class with some fundamental features and methods that are essential for proper functionality, such as:
The ability to create an instance of the class.
Support for basic object operations such as comparison and copying.
The capability to define and access object attributes.
By explicitly inheriting from object
, we ensure that our class has access to these core functionalities. It acts as a base class from which all other classes are derived.
🐍 Common issues without object
inheritance
1. Missing essential methods
If you omit the object
inheritance and define a class like this:
class MyClass:
...
Your class will still work fine in most cases. However, it will lack some crucial methods that a class with object
inheritance automatically gets.
For example, without inheriting from object
, your class won't have the __init__()
, __del__()
, or __str__()
methods. These methods are essential for proper object initialization, cleanup, and human-readable string representation, respectively.
2. Compatibility issues with certain libraries and frameworks
Some third-party libraries and frameworks rely on the fact that classes inherit from object
. They may expect certain behaviors or use special methods that are provided by the object
class. If you omit this inheritance, you might encounter compatibility issues with such libraries.
To ensure seamless integration and compatibility, it's best practice to always inherit from object
.
✅ Easy solutions
In Python 3.x, explicitly inheriting from object
is no longer required, as all classes implicitly inherit from it. However, for backward compatibility with older versions of Python, it's still recommended to include it.
So, to future-proof your code and avoid potential issues, simply include object
as the base class:
class MyClass(object):
...
By doing so, you ensure that your class has access to all the core functionalities and maintain compatibility across different Python versions.
📣 Share your thoughts
Do you always remember to inherit from object
when crafting your Python classes? Share your experiences in the comments below!
Remember, by including object
as the base class, you guarantee compatibility and avoid potential pitfalls. So, don't forget to embrace this Pythonic convention in your code!
Happy coding! 🚀