Why do I get "TypeError: not all arguments converted during string formatting" trying to substitute a placeholder like {0} using %?
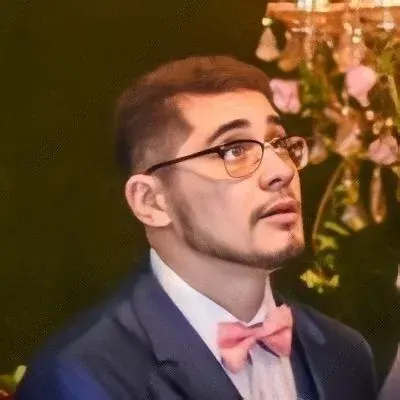
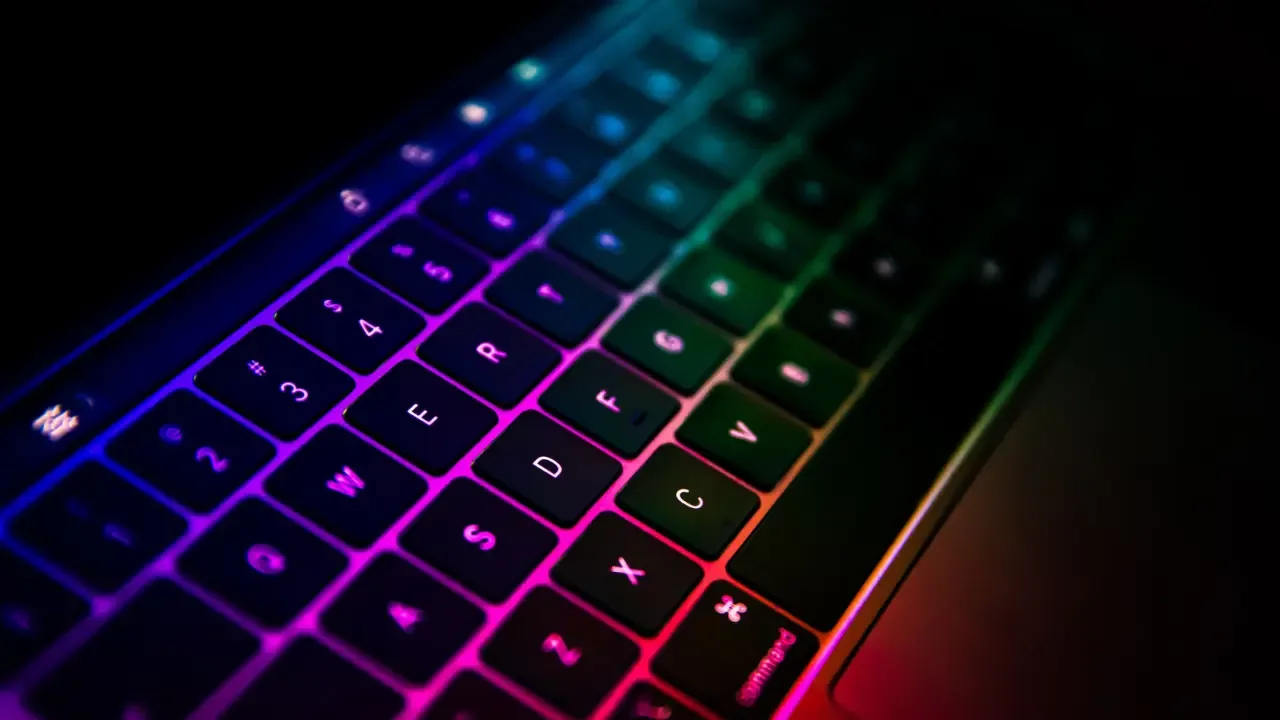
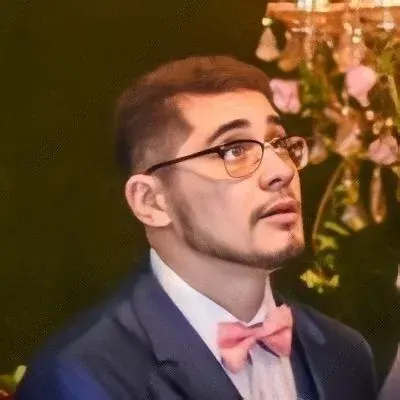
π Emoji-Tech Blog Post: Why do I get "TypeError: not all arguments converted during string formatting" trying to substitute a placeholder like {0} using %?
π Hey there, code enthusiasts! π©βπ»π¨βπ»
Have you ever encountered the infamous "TypeError: not all arguments converted during string formatting" error message in Python? π It can be quite frustrating, especially when you're trying to substitute a placeholder like {0} using the % operator. But fear not! π‘οΈ We're here to demystify this error and provide you with easy solutions. Let's dive in! πͺ
So, let's take a look at a code snippet that might trigger this error:
name1 = input("Enter name 1: ")
name2 = input("Enter name 2: ")
if len(name1) > len(name2):
print("'{0}' is longer than '{1}'" % name1, name2)
And boom, we witness the dreaded "TypeError: not all arguments converted during string formatting" error. What's going wrong here? π€
The issue lies in the way the string formatting is being done. In this case, the % operator is trying to use two separate arguments for string substitution. However, it mistakenly interprets name2
as a separate argument instead of being part of the string formatting operation, resulting in the error.
Fear not, fellow coder! We have the solution. π‘
To fix this error and format the string properly, you can enclose the two variables within a tuple:
if len(name1) > len(name2):
print("'{0}' is longer than '{1}'".format(name1, name2))
Using .format()
instead of the % operator allows for clearer separation between the string and the variables being substituted. By passing the variables as arguments within the .format()
method, you ensure that they are correctly substituted in the string.
Easy as pie, right? π°
To sum it up, here's a breakdown of the steps to fix the "TypeError: not all arguments converted during string formatting" error:
Replace the string formatting with
.format()
instead of %.Enclose the variables within a tuple inside the string.
Remember, it's always better to use the more modern .format()
method for string formatting in Python. It allows for more flexibility and readability in your code. π
Now that you've successfully fixed this pesky error, go ahead and implement the changes in your code. β¨
Have you ever encountered this error or any other Python coding challenges? Share your experience in the comments below! Let's learn and grow together. π
β‘οΈ Need more guidance on Python string formatting? Check out these helpful resources to level up your skills:
π String formatting: % vs. .format vs. f-string literal: Explore the pros and cons of the most common ways to format strings in Python.
π How do I put a variableβs value inside a string (interpolate it into the string)?: Get a general how-to guide for this kind of string construction.
π Printing tuple with string formatting in Python: Dive into handling tuple printing with string formatting and address potential pitfalls.
That's all for now, folks! Happy coding! π»π