Why can"t dataclasses have mutable defaults in their class attributes declaration?
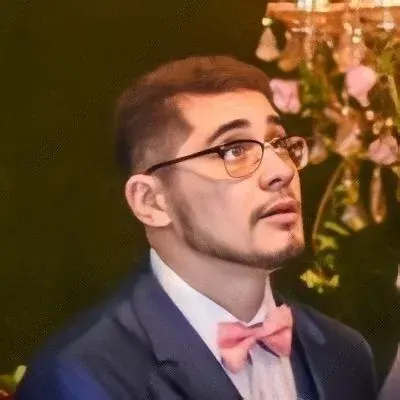
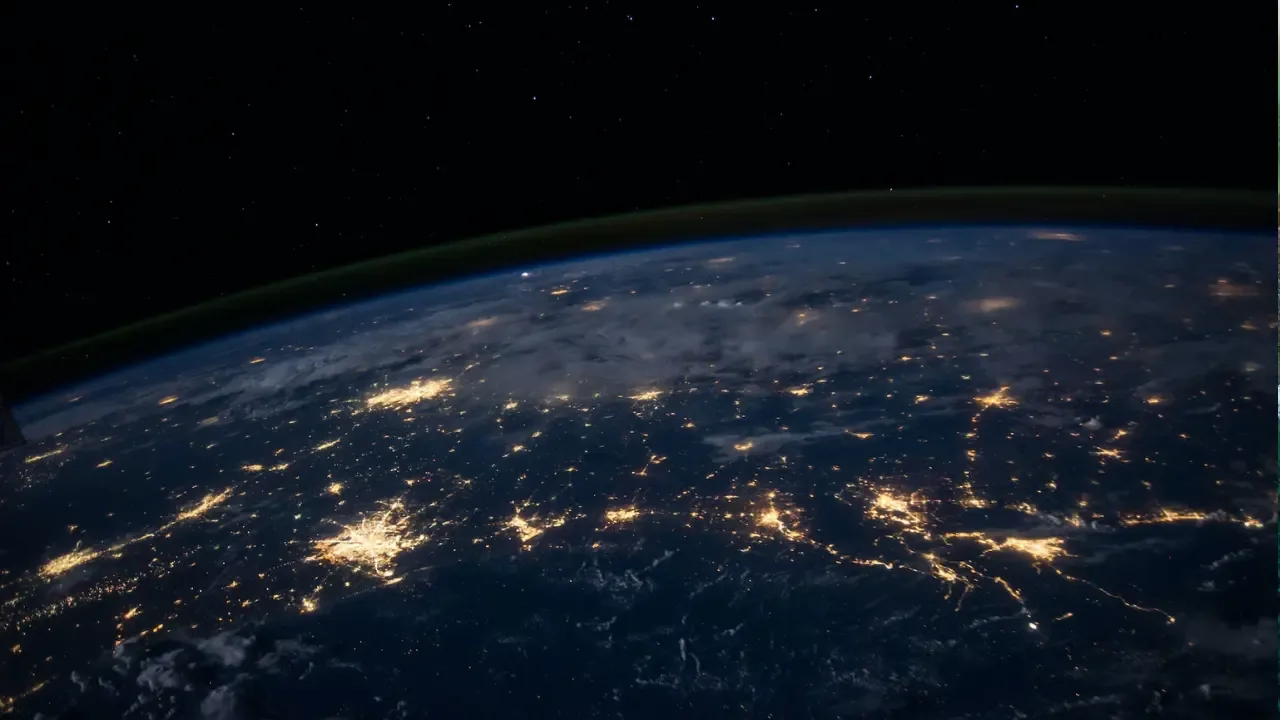
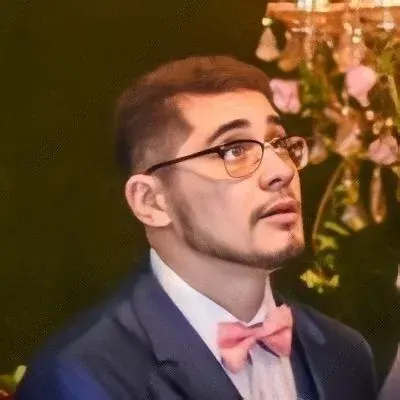
Dataclasses and Mutable Defaults: A Complete Guide 🧬✏️
Are you a Python developer who loves using dataclasses but gets frustrated when trying to assign mutable values as defaults in class attributes? You're not alone! 🙋♂️
In this blog post, we'll dive deep into this common issue and provide easy solutions to help you overcome it. By the end, you'll understand why dataclasses don't allow mutable defaults and how to work around it. Let's get started! 💪
The Problem 🤔❌
So, you've written a dataclass and want to assign a default value to one of its attributes. Easy peasy, right? Not quite! When you try to assign a mutable value, like a list, to a class attribute, you encounter an error message:
@dataclass
class Foo:
bar: list = []
# ValueError: mutable default <class 'list'> for field a is not allowed: use default_factory
Why is this happening? And why do we need to use default_factory
instead? Let's explore the reasons behind this limitation. 💡
Mutable Defaults: The Danger Zone ⚠️😱
To understand why mutable defaults are not allowed in dataclasses, we need to dive into the infamous "mutable default argument problem." This problem arises when using mutable objects, such as lists or dictionaries, as default function arguments. Let's see an example:
def append_to_list(element, my_list=[]):
my_list.append(element)
return my_list
# The expected behavior:
print(append_to_list(1)) # [1]
# But what happens here?
print(append_to_list(2)) # [1, 2] instead of [2]
The unexpected behavior occurs because the default argument (my_list=[]
) is evaluated at function definition time, not each time the function is called. This means that the same list object is used across multiple function calls, leading to unexpected results. 😫
Now imagine if dataclasses allowed mutable object defaults for their attributes. We could face similar issues when creating multiple instances of the same dataclass. This is why Python's dataclasses take precautions to prevent this problem. 🛡️
The Solution: default_factory
🛠️🔧
To overcome the limitation of mutable defaults, Python's dataclasses provide the default_factory
parameter. This parameter allows you to specify a callable object that generates a new default value for each attribute. Here's how to use it:
from dataclasses import field
@dataclass
class Foo:
bar: list = field(default_factory=list)
In this example, we assign list
as the default factory for the bar
attribute. Now, each time a new instance of Foo
is created, a new and empty list will be assigned to bar
, avoiding the mutable default argument problem.
Conclusion and Call-to-Action 🎉🚀
We've explored why dataclasses don't allow mutable defaults and how to work around this limitation using default_factory
. By understanding the dangers of mutable defaults and applying the correct approach, you can ensure predictable behavior in your code.
Next time you encounter the "mutable default" error in your dataclasses, remember the power of default_factory
! 🤩
Now it's your turn to take action! Try implementing default_factory
in your dataclasses and share your experiences in the comments below. I'd love to hear about any challenges you faced and the creative solutions you came up with! Let's keep the conversation going! 💬💡
Happy coding! 🐍💻