Why am I seeing "TypeError: string indices must be integers"?
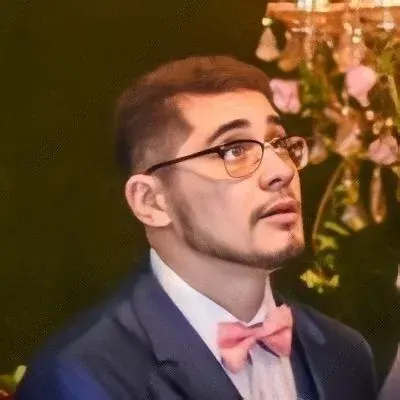
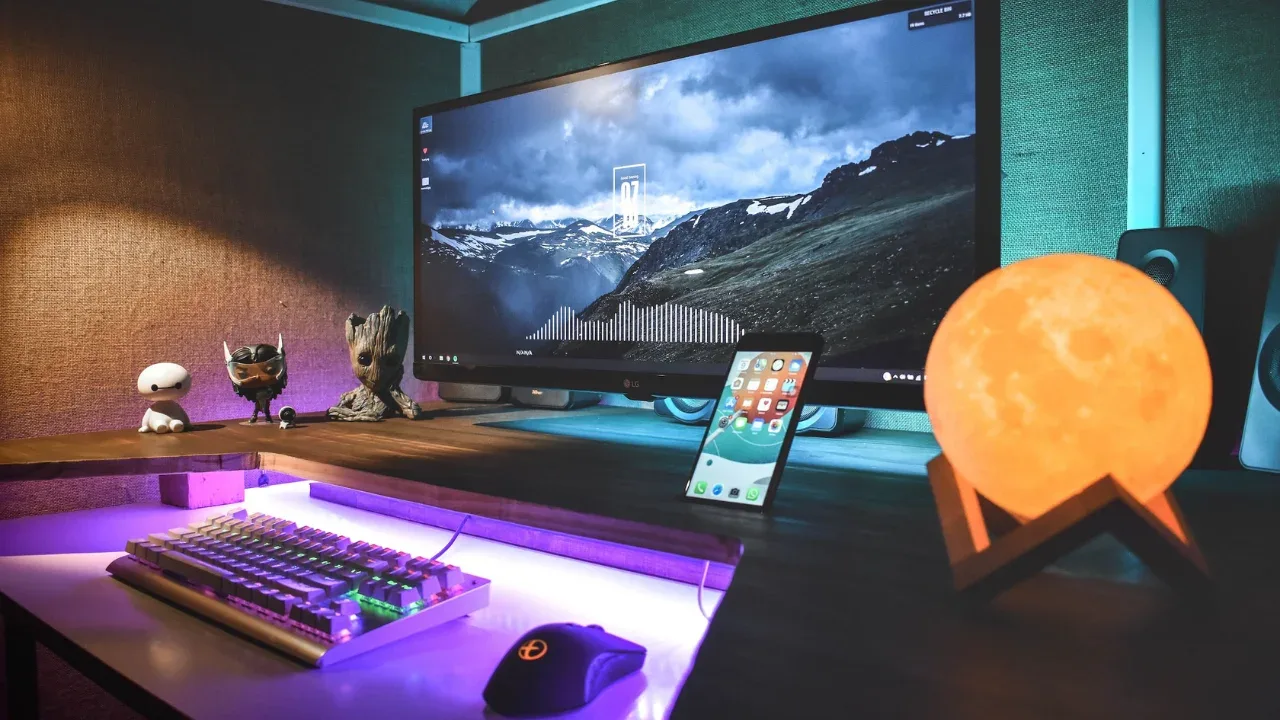
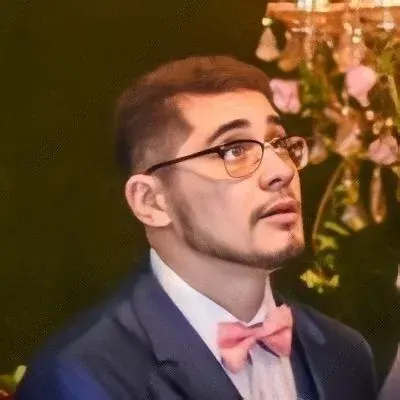
Why am I seeing "TypeError: string indices must be integers"?
Are you getting frustrated with the dreaded "TypeError: string indices must be integers" error in your Python code? Don't worry, you're not alone! This common error occurs when you try to use a string as an index in your code instead of an integer. But hey, we've got your back! In this blog post, we'll explain why this error occurs and provide some easy solutions to fix it. 🐍🔢
Understanding the error
Let's take a closer look at the code snippet that triggered the error:
for item in data:
csv_file.writerow([item["gravatar_id"], item["position"], item["number"], item["votes"], item["created_at"], item["comments"], item["body"], item["title"], item["updated_at"], item["html_url"], item["user"], item["labels"], item["state"]])
In this code, you are trying to access the values of different properties within each item
in the data
object. However, the TypeError is thrown because the item
is treated as a string instead of a dictionary.
Identifying the issue
Based on the provided context, we can see that the data
object is loaded from a JSON file. When you printed the data
object, you noticed that the keys and values were represented differently. For example, instead of "gravatar_id"
, you saw u'gravatar_id'
. This indicates that the JSON file was loaded with Unicode representation.
Fixing the error
To fix the "TypeError: string indices must be integers" error, you need to handle the Unicode representation correctly. You can do this by decoding the loaded data using the json.loads()
function:
data = json.loads(f.read().decode('utf-8'))
By using json.loads()
along with decode('utf-8')
, you decode the data and convert it into a Unicode string, allowing you to access the dictionary keys properly.
The corrected code
Here's an updated version of your code with the fix applied:
import json
import csv
f = open('issues.json')
data = json.loads(f.read().decode('utf-8'))
f.close()
f = open("issues.csv", "wb+")
csv_file = csv.writer(f)
csv_file.writerow(["gravatar_id", "position", "number", "votes", "created_at", "comments", "body", "title", "updated_at", "html_url", "user", "labels", "state"])
for item in data:
csv_file.writerow([item["gravatar_id"], item["position"], item["number"], item["votes"], item["created_at"], item["comments"], item["body"], item["title"], item["updated_at"], item["html_url"], item["user"], item["labels"], item["state"]])
🎉 Hurray! With this fix, you should no longer encounter the "TypeError: string indices must be integers" error. Now your code will run smoothly and populate your CSV file with the desired data from the JSON file.
Conclusion
Dealing with errors can be frustrating, but understanding their causes and finding the right solutions is a valuable skill for any developer. In this blog post, we addressed the "TypeError: string indices must be integers" error, which commonly occurs when using a string index instead of an integer index. By decoding the loaded data properly, we were able to fix the error and provide you with working code.
If you found this blog post helpful, be sure to share it with your fellow coders and spread the knowledge! And don't forget to leave a comment below if you have any questions or other error-related topics you'd like us to cover. Happy coding! 🚀💻