Which is the preferred way to concatenate a string in Python?
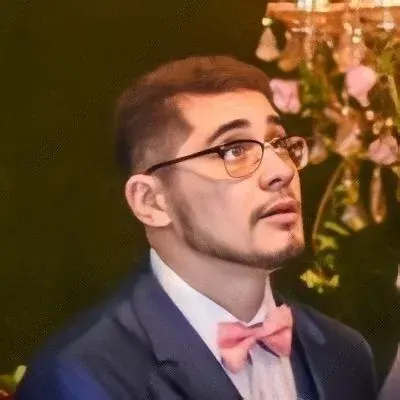
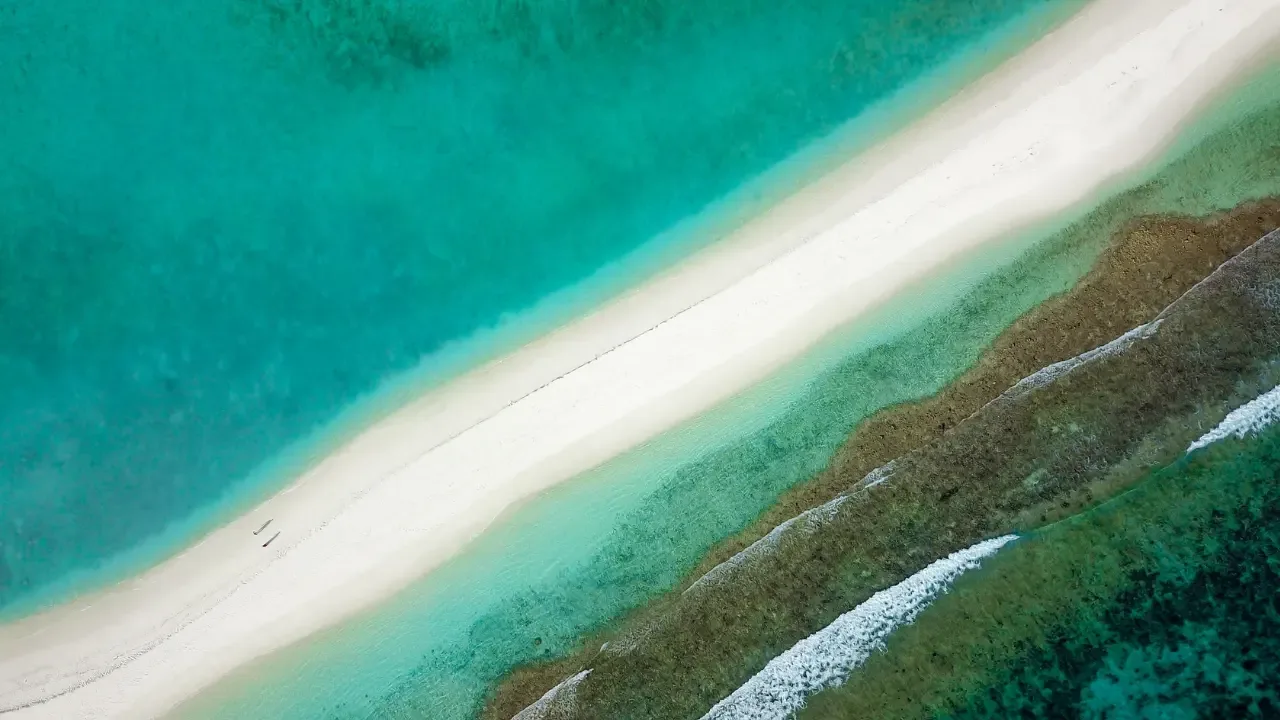
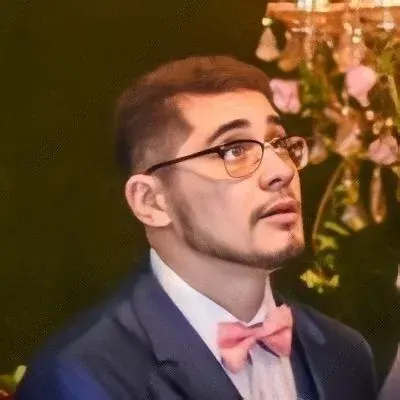
The Preferred Way to Concatenate a String in Python
š¤ Are you wondering how to efficiently concatenate a string in Python? You've come to the right place! In this article, we'll explore the different methods of string concatenation and determine the preferred way in Python, specifically in Python 3.
The Challenge of Concatenating Strings
Python's string
data type is immutable, meaning you can't modify it directly. So, how can we efficiently concatenate strings without creating unnecessary copies of the original string? Let's examine the two common approaches.
Approach 1: Using the +=
Operator
One way to concatenate strings in Python is by using the +=
operator. This approach is simple and straightforward:
s += stringfromelsewhere
This code appends the value of stringfromelsewhere
to the existing string s
. However, it's important to note that this method creates a new string object every time you concatenate, resulting in unnecessary memory usage. š§ š„
Approach 2: Using a List and join()
To tackle the memory issue, another approach is to use a list to store the string fragments and then join them together using Python's built-in join()
method. Here's how it works:
s = []
s.append(somestring)
# later
s = ''.join(s)
In this approach, we create an empty list s
and append each string fragment using the append()
method. Then, when we're ready to finalize the concatenation, we use join()
to efficiently combine all the elements of the list into a single string.
Using this method, we only create one new string object instead of multiple intermediate copies, resulting in improved memory efficiency. š”š
Python 3 vs. Python 2
While doing research on this topic, you may come across articles discussing Python 2.x. But don't worry ā the preferred way to concatenate strings remains the same in Python 3!
The +=
operator and the list with join()
approach are both valid in Python 3. However, it's worth noting that the list and join()
method approach offers better performance when dealing with a large number of string concatenations. š
Conclusion and Your Next Steps
Now that you know the preferred way to concatenate strings in Python, it's time to put your newfound knowledge into practice. Experiment with different methods and determine which approach works best for your specific use cases.
Remember, choosing the right method can significantly impact your code's efficiency and overall performance. So choose wisely! šŖš
š To dive deeper into this topic, you can check out this helpful article: Efficiency of String Concatenation in Python.
š£ Now it's your turn! Share your experience with string concatenation in Python in the comments below. What challenges did you face, and how did you overcome them? Let's learn together! š¬š”