When should iteritems() be used instead of items()?
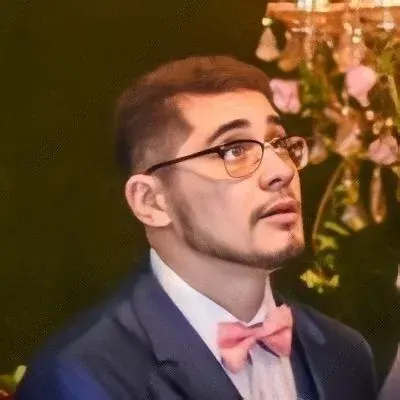
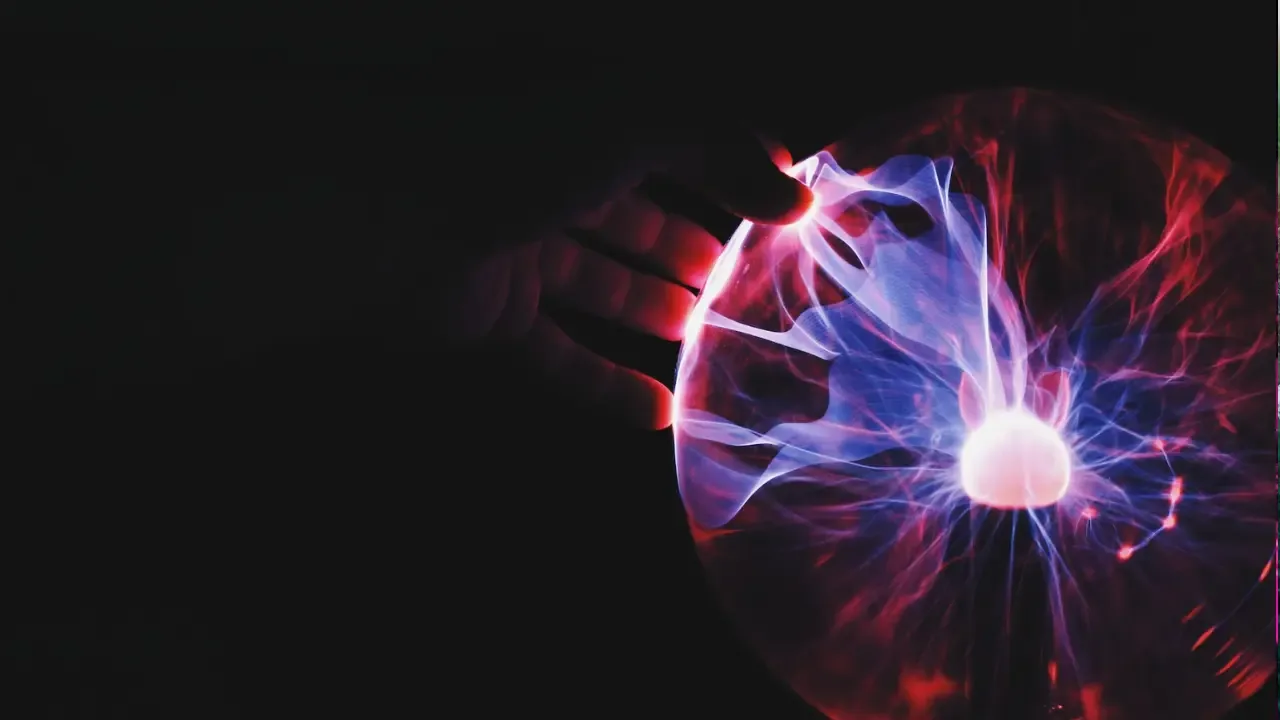
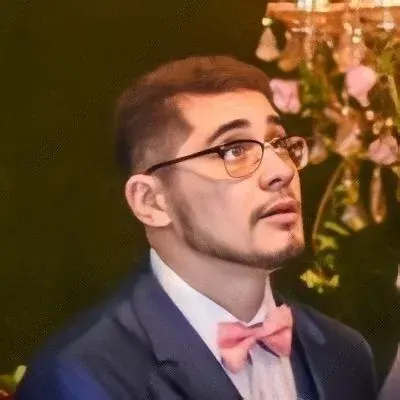
📝When to Use iteritems() Instead of items() in Python
Are you confused about when to use iteritems()
instead of items()
in Python? 🐍 Don't worry, you're not alone! Many developers wonder about the difference between these two methods and why iteritems()
was removed in Python 3. In this blog post, we'll address these common questions and provide easy solutions for your Python programming needs.
🧐 The Dilemma: items() vs. iteritems()
In Python, both items()
and iteritems()
are dictionary methods used to iterate over key-value pairs. 🗝️🔑 The main difference is their behavior and compatibility with different Python versions. Let's dive deeper into each method to understand their nuances.
📚 items() Method
The items()
method returns a list-like object containing all the key-value pairs of a dictionary. This means that all the pairs are loaded into memory at once, which can be inefficient if you're working with a large dictionary. However, it's important to note that this method is available in both Python 2 and Python 3. Here's an example of using items()
:
my_dict = {'apple': 5, 'banana': 3, 'orange': 7}
for key, value in my_dict.items():
print(f"The count of {key} is {value}.")
🎩 iteritems() Method
The iteritems()
method, on the other hand, returns an iterator object that yields one key-value pair at a time. It doesn't load all the pairs into memory at once, making it more memory-efficient, especially when dealing with large dictionaries. However, this method is only available in Python 2 and has been removed in Python 3. Here's an example of using iteritems()
:
my_dict = {'apple': 5, 'banana': 3, 'orange': 7}
for key, value in my_dict.iteritems():
print(f"The count of {key} is {value}.")
💡 Solutions for Compatibility and Efficiency
Now that we understand the differences between items()
and iteritems()
, let's address the main concern: iterating over a dictionary in a generator-like way that is compatible with both Python 2 and Python 3. 🔄
The best way to achieve this is to use the items()
method in Python 3 and wrap it with the list()
function in Python 2. This ensures compatibility while maintaining efficiency for large dictionaries. Here's an example of implementing this solution:
my_dict = {'apple': 5, 'banana': 3, 'orange': 7}
# For Python 2 and Python 3 compatibility
if sys.version_info.major < 3:
dict_items = my_dict.iteritems()
else:
dict_items = my_dict.items()
for key, value in dict_items:
print(f"The count of {key} is {value}.")
💬 Join the Discussion!
Now that you have a clearer understanding of when to use iteritems()
instead of items()
, it's time to put your knowledge into practice. Share your thoughts, experiences, or any other insights related to this topic in the comments section below. Let's learn from each other and keep the conversation going! 💬🚀
So, the next time you find yourself in a dilemma between items()
and iteritems()
, remember the Pythonic way of iterating over dictionaries 🐍 and choose wisely based on compatibility and efficiency considerations. Happy coding! 😄✨