What"s the canonical way to check for type in Python?
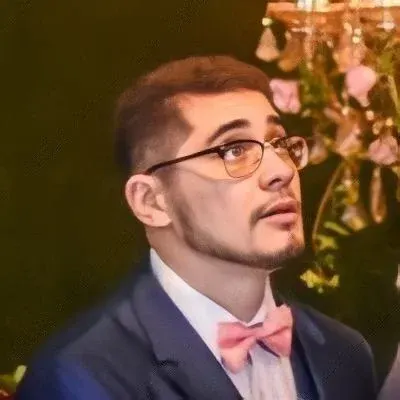
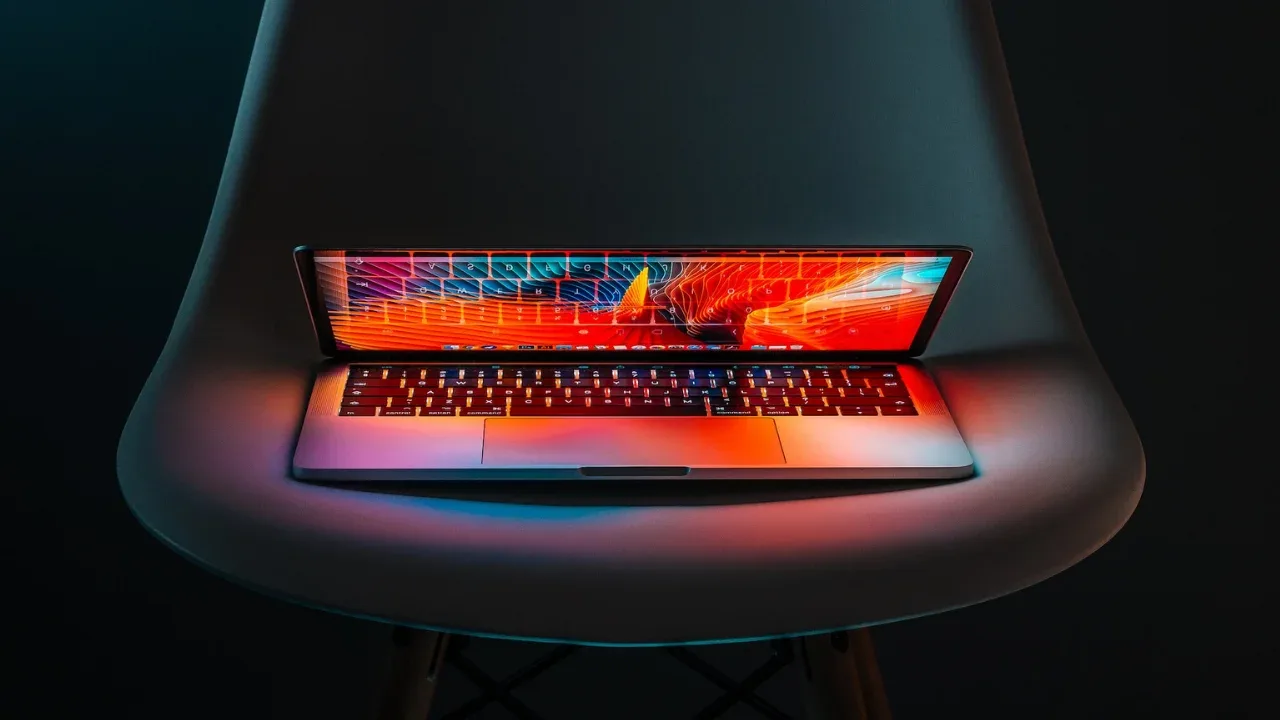
What's the Canonical Way to Check for Type in Python? ππ§ͺ
So, you're writing some Python code and you want to check if an object is of a specific type or if it inherits from a certain type. You might be wondering, what's the best way to do this? π€
Let's dive right in and address this common issue with some easy solutions! π‘
Checking if an object is of a given type π΅οΈββοΈ
To check if an object o
is of type str
, you can use the isinstance()
function like this:
o = "Hello, World!"
if isinstance(o, str):
print("It's a string!")
else:
print("It's not a string.")
So, if o
is indeed a string, it will print "It's a string!" π€. Otherwise, it will print "It's not a string." β
Checking if an object inherits from a given type π¨βπ§βπ¦
If you want to check if an object o
inherits from a specific type, you can use the issubclass()
function:
class Parent:
pass
class Child(Parent):
pass
o = Child()
if issubclass(type(o), Parent):
print("It's a child of Parent!")
else:
print("It's not a child of Parent.")
In this example, Child
is a subclass of Parent
. Therefore, the code will print "It's a child of Parent!" πΆπ¨βπ§βπ¦. If o
was not a subclass of Parent
, it would print "It's not a child of Parent." β
Common Pitfalls and Useful Resources π§π
Beginners often fall into the trap of assuming that a string can automatically be treated as a number or any other specific type. Remember, Python doesn't perform these automatic conversions.
If you're looking to check if a string represents a number, you can refer to the following Stack Overflow questions for the right approach:
These resources will provide you with valuable insights and help you handle different scenarios.
Take Action! π
Now that you know the canonical ways to check for type in Python, go ahead and apply this knowledge to your code. Be proactive and make your code more robust by validating the type of your objects when necessary.
If you found this blog post helpful, feel free to share it with your fellow Pythonistas! And don't hesitate to leave a comment below, sharing your experience or any other tips you might have. Let's keep the Python community thriving! ππ»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
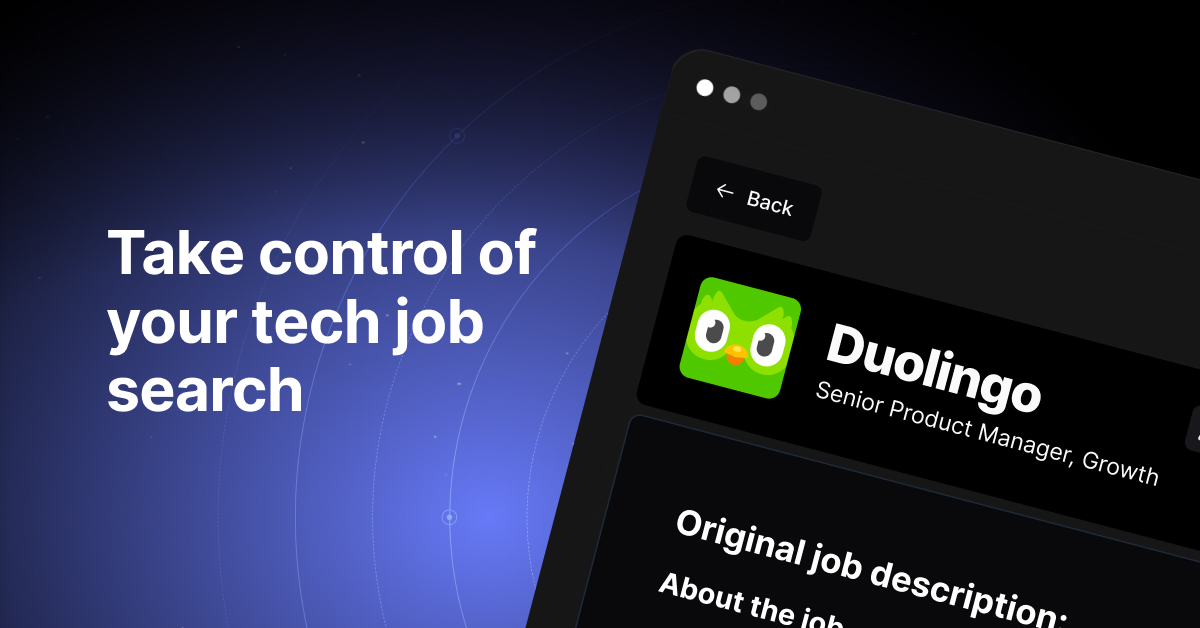