What"s the best way to store a phone number in Django models?
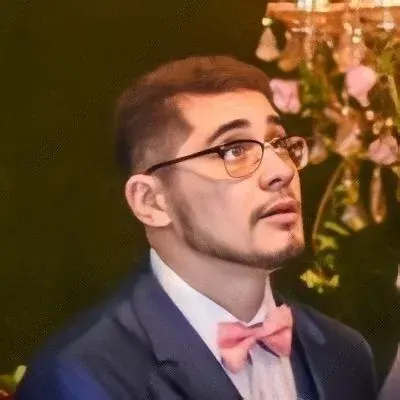
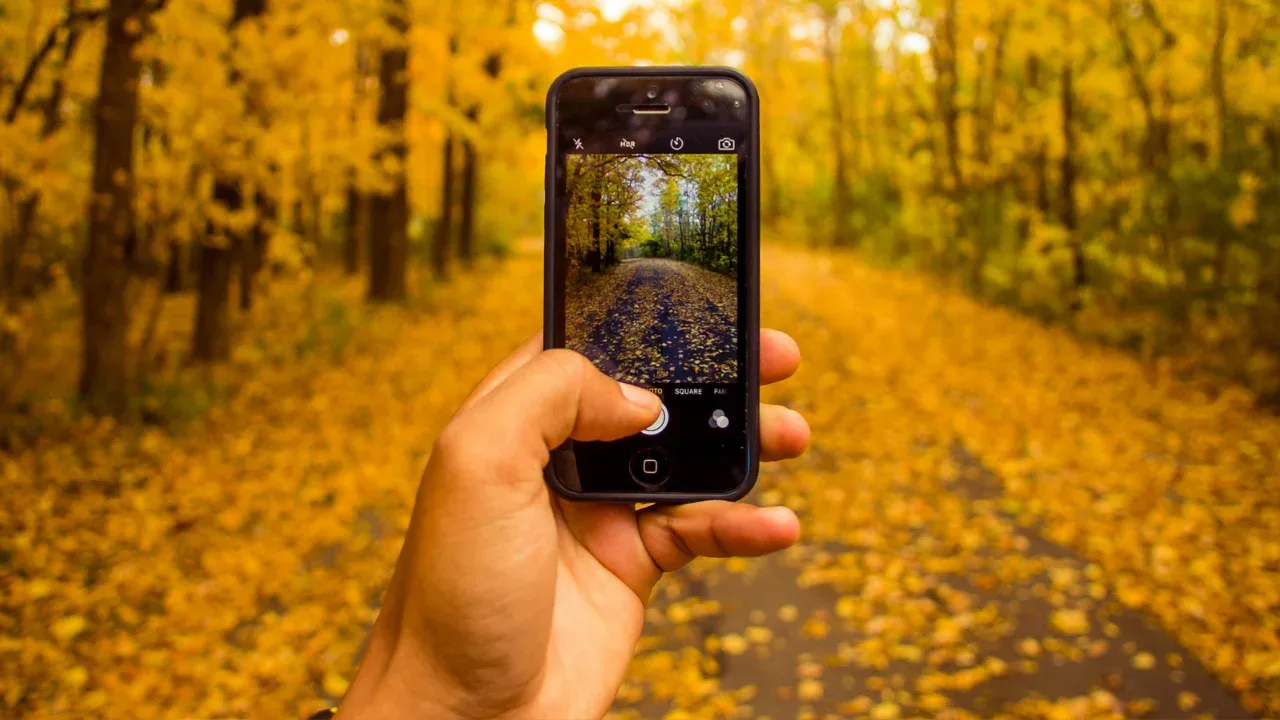
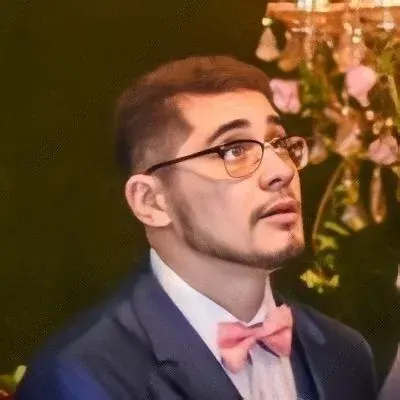
📱 What's the Best Way to Store a Phone Number in Django Models? 📱
Are you building a Django application that requires storing phone numbers and need guidance on the best approach? Look no further! In this blog post, we will address the common issues and provide easy solutions to efficiently store phone numbers in Django models. Let's dive in!
💡 The Challenge: Storing Phone Numbers with Country Codes 💡
The user in our example is storing a phone number using a CharField
with a maximum length of 12 characters. While this might seem like a straightforward solution initially, we need to consider a few factors before proceeding.
🌍 Going Global: Considering Country Codes 🌍
Since the application will be used globally, it's crucial to include country codes for phone numbers. Without the country code, it becomes challenging to identify the correct region for SMS authentication. So how do we incorporate country codes into our phone number storage?
🚀 The Solution: Using a Custom Phone Number Field 🚀
To handle phone numbers with country codes effectively, we recommend creating a custom model field using Django's built-in PhoneNumberField
. This field provided by the phonenumbers
library will ensure the proper format and validation of phone numbers.
First, make sure you have the phonenumbers
library installed:
pip install phonenumbers
Next, import the necessary modules in your Django model:
import phonenumbers
from phonenumbers import PhoneNumber
from django.db import models
from django.core.exceptions import ValidationError
Now, let's create our custom phone number field:
class CustomPhoneNumberField(models.CharField):
def __init__(self, *args, **kwargs):
kwargs['max_length'] = kwargs.get('max_length', 20)
super().__init__(*args, **kwargs)
def from_db_value(self, value, expression, connection):
if value is None:
return value
try:
return phonenumbers.parse(value, None)
except phonenumbers.phonenumberutil.NumberParseException:
raise ValidationError("Invalid phone number format.")
def to_python(self, value):
if isinstance(value, PhoneNumber):
return value
try:
return phonenumbers.parse(value, None)
except phonenumbers.phonenumberutil.NumberParseException:
raise ValidationError("Invalid phone number format.")
def get_prep_value(self, value):
if not value:
return None
return str(value)
def value_to_string(self, obj):
value = self.value_from_object(obj)
return self.get_prep_value(value)
🎉 How to Use the Custom Phone Number Field 🎉
Now that we have our custom field set up, we can use it in our models. Let's update the phone_number
field in your example model:
phone_number = CustomPhoneNumberField(max_length=20)
With this setup, any phone number entered by the user will be validated and stored with its corresponding country code. Django's PhoneNumberField
in combination with the phonenumbers
library takes care of the heavy lifting, ensuring proper phone number formats.
👏 Encourage Reader Engagement and Feedback 👏
We hope this guide has helped you find the best way to store phone numbers in Django models while incorporating country codes. If you have any questions or suggestions, feel free to leave a comment below. We'd love to hear your thoughts! 🗣️
Happy coding! 💻✨