What"s the best way to parse a JSON response from the requests library?
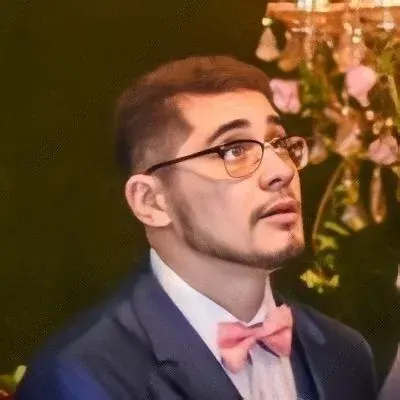
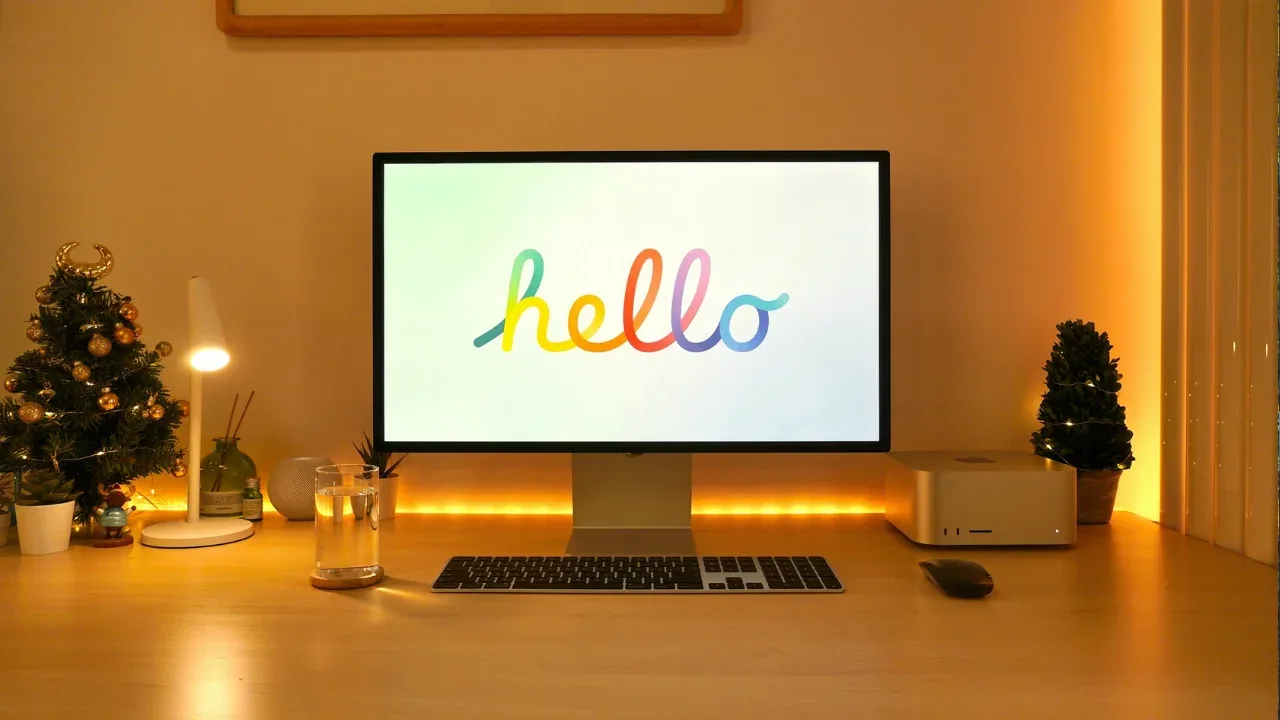
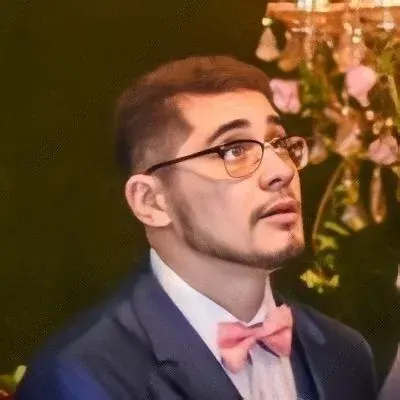
Title: Unleashing the Power of JSON Parsing with the Requests Library 💪🔥
Introduction:
Are you struggling to make sense of the JSON response you received from the requests
library? Fret no more, because we're here to help you unlock the magic of parsing JSON with ease! 🌟
The Challenge:
You've made a RESTful GET request using the fantastic requests
library in Python. The server responds with a JSON document that appears to be just a list of lists. You now face the daunting task of transforming this JSON into a native Python object for further processing. How do you conquer this challenge? 🤔
Introducing the JSON Response Beast 🐲:
To tame this magnificent JSON response beast, we must traverse its structure and transform it into a friendly Python object. Let's dive into some easy solutions that will make you a JSON parsing maestro! 💪🎩
Solution 1: The Mighty .json()
Method 🌟:
The requests
library provides us with a simple solution: the .json()
method. This elegant method automatically decodes the JSON response into a native Python object. Here's how you do it:
import requests
response = requests.get('https://api.example.com/endpoint')
response_json = response.json()
# Voila! You can now iterate, print, or perform any operations on the response_json 🎉
Solution 2: The Powerful json.loads()
Function 🚀:
Perhaps you're looking for an alternative method or need more control over the parsing process. Fear not, for the json
module comes to your rescue with the mighty json.loads()
function. This function takes a JSON string and returns a native Python object. Here's an example:
import json
import requests
response = requests.get('https://api.example.com/endpoint')
json_string = response.text
response_json = json.loads(json_string)
# Huzzah! You're now wielding the power of a Python object 🎩✨
Solution 3: Commanding the JSON Flattening Technique 🌊:
In some cases, the JSON response may contain nested structures that can be challenging to handle. To flatten such complex structures into a simple list, we can utilize the json_normalize()
function from the pandas
library. Let's see it in action:
import pandas as pd
import requests
response = requests.get('https://api.example.com/endpoint')
response_json = response.json()
df = pd.json_normalize(response_json)
# Amazing! You've flattened the JSON response into a tabular structure 📊💥
Conclusion:
Parsing a JSON response doesn't have to be a daunting task. With the right tools and techniques, you can effortlessly transform JSON into a Python object. Whether you prefer the simplicity of .json()
, the full control of json.loads()
, or the JSON flattening technique with json_normalize()
, you now have the power to conquer any JSON parsing obstacle! 🎯💼
🌟🔥 Call-to-Action: Share Your JSON Victories 🔥🌟
Now that you're equipped with new JSON parsing superpowers, put them to use! Share your successful JSON parsing stories, helpful tips, or any other thoughts in the comments section below. Let's build a vibrant community of JSON warriors together! 💪💬
(Optional) You can also subscribe to our newsletter to receive regular updates with more awesome tech tips! 💌
Happy parsing, fellow developers! May the JSON gods smile upon you! 🙌✨🔮