What is the use of "assert" in Python?
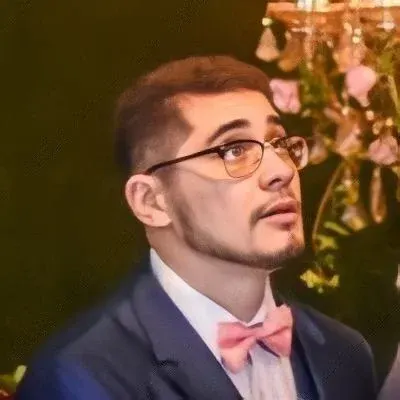
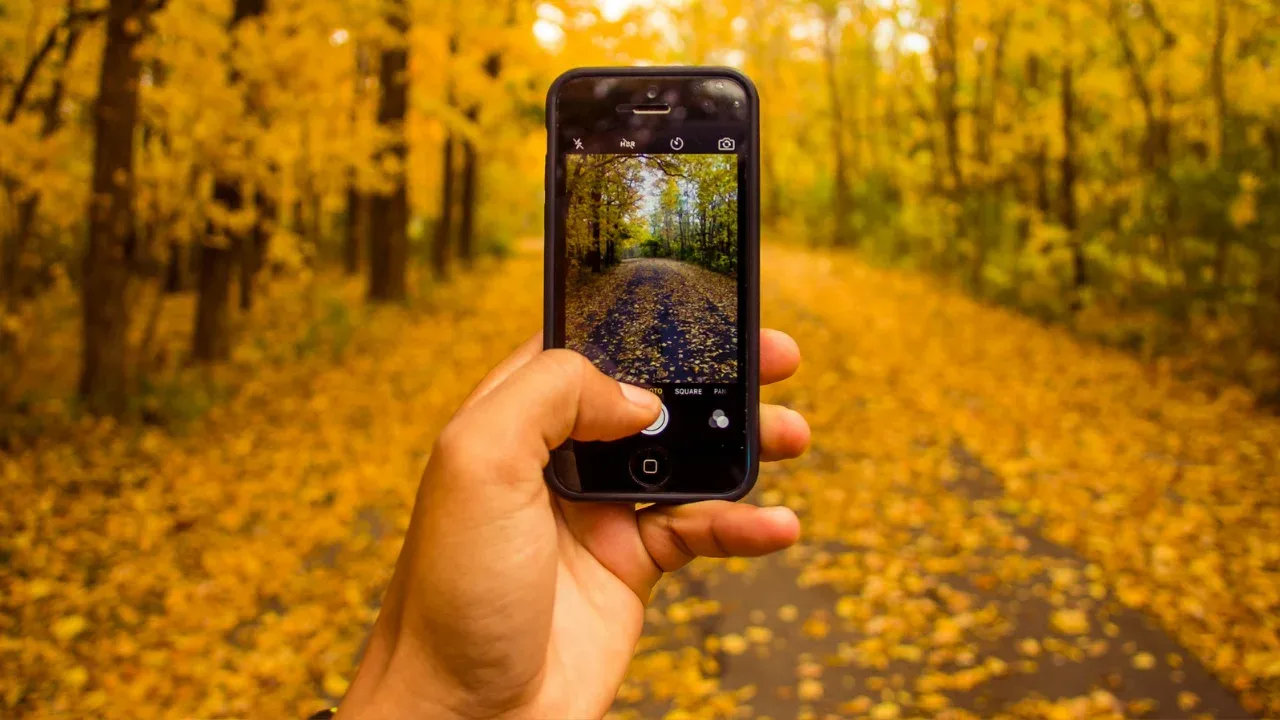
š Title: Demystifying the Power of "assert" in Python š
⨠Introduction āØ
Do you ever find yourself questioning the purpose of the enigmatic "assert" statement in Python? š¤ Fear not! In this comprehensive guide, we'll unravel the mystery surrounding "assert" and explore its multitude of practical applications. Whether you're a beginner or an experienced Pythonista, you're bound to find something valuable in this article. Let's dive into the fascinating world of "assert" together! š
š Understanding the Basics š§©
In its simplest form, the "assert" statement acts as a powerful debugging tool and a runtime assertion. It allows you to check if certain conditions in your code are true and take appropriate actions based on the results. Here's a handy syntax reminder:
assert condition, message
condition: Represents the expression or condition to be evaluated.
message: Provides additional information to be displayed if the condition evaluates to False (optional).
š” Common Use Case Scenarios š»
1. Debugging and Error Detection:
"assert" excels at finding coding errors and ensuring your assumptions hold true during development. Let's consider an example:
def calculate_discount(total_price, discount_percentage):
assert total_price > 0, "Total price should be greater than zero."
assert 0 <= discount_percentage <= 100, "Discount percentage should be between 0 and 100."
discounted_price = total_price * (1 - (discount_percentage / 100))
return discounted_price
If any of the conditions in the "assert" statements fail, Python will raise an AssertionError and display the corresponding message. This helps catch and rectify issues early on, leading to robust code. š
2. Contract Checking:
"assert" can also serve as a runtime contract checker, ensuring the inputs and outputs of functions or methods adhere to predefined constraints. Here's an example:
def divide(a, b):
assert b != 0, "Cannot divide by zero."
return a / b
By asserting that the divisor (b) is not zero, we prevent potential ZeroDivisionErrors. This provides a safety net, preventing unexpected crashes and enhancing overall reliability. ā”ļø
ā Overcoming Challenges & Best Practices āØ
While "assert" is a valuable tool, it's essential to keep a few things in mind to maximize its effectiveness:
Use "assert" for debugging purposes during development, but consider removing or disabling them in production code to improve performance.
Ensure the conditions you specify are testable and valuable for identifying potential bugs.
Avoid complex or time-consuming operations in the "assert" statement, as they may impact the performance of your code. ā ļø
š Conclusion & Call-to-Action šŖ
Congratulations! You've successfully unlocked the secrets of Python's "assert" statement and its versatile applications. Harnessing the power of "assert" will undoubtedly elevate your debugging skills and reinforce the reliability of your code. Start implementing assert statements in your projects today, and witness the tangible benefits it brings! āØš
If you found this article useful or have further questions, let's continue the conversation in the comments below. Share your "assert" success stories or any challenges you've encountered. And don't forget to hit the share button and spread the knowledge with your fellow Python enthusiasts! Let's debug and assert our way to success! šŖš
Disclaimer: "assert" statement usage may vary based on individual coding practices and project requirements. Always consult the official Python documentation and community guidelines for best practices and conventions. šš
ā FAQ ā
Q: Isn't "assert" similar to using regular if-else statements? A: While both serve similar purposes, "assert" focuses on self-checking and raising AssertionError exceptions when a condition fails. In contrast, if-else statements allow you to customize the fault handling and perform more complex operations. "assert" is primarily used for debugging and contract checking.
Q: Can I disable "assert" statements if necessary? A: Yes! You can pass the -O (optimize) flag or set the debug variable to False when running your Python program. This will disable all "assert" statements, potentially improving performance.
Q: How can I use "assert" alongside unit testing frameworks? A: Excellent question! Most unit testing frameworks have built-in assertion mechanisms. Instead of using "assert", it's better to leverage the functionalities provided by testing frameworks like unittest, pytest, or doctest. They offer more features and detailed test outputs.
That's all, folks! Thank you for joining us on this "assert" adventure. We hope you found this guide helpful and enjoyable. Keep coding, exploring, and asserting with confidence! šš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
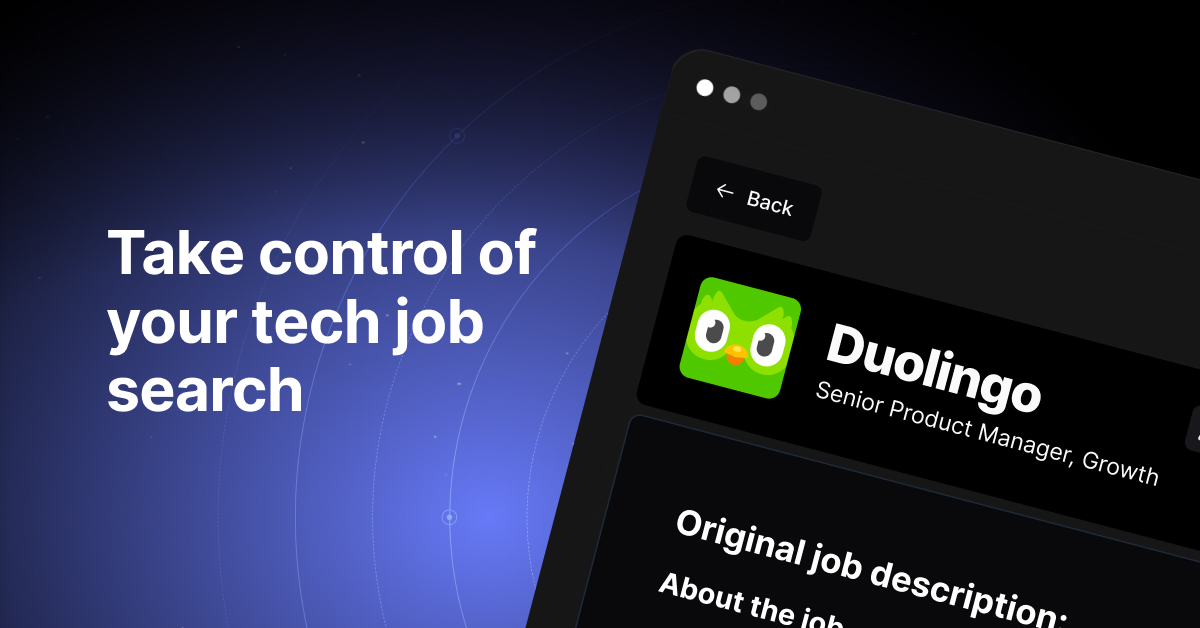