What is the "@=" symbol for in Python?
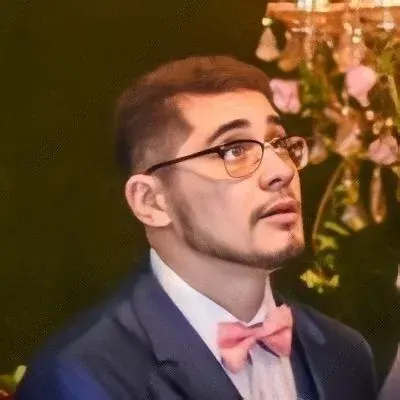
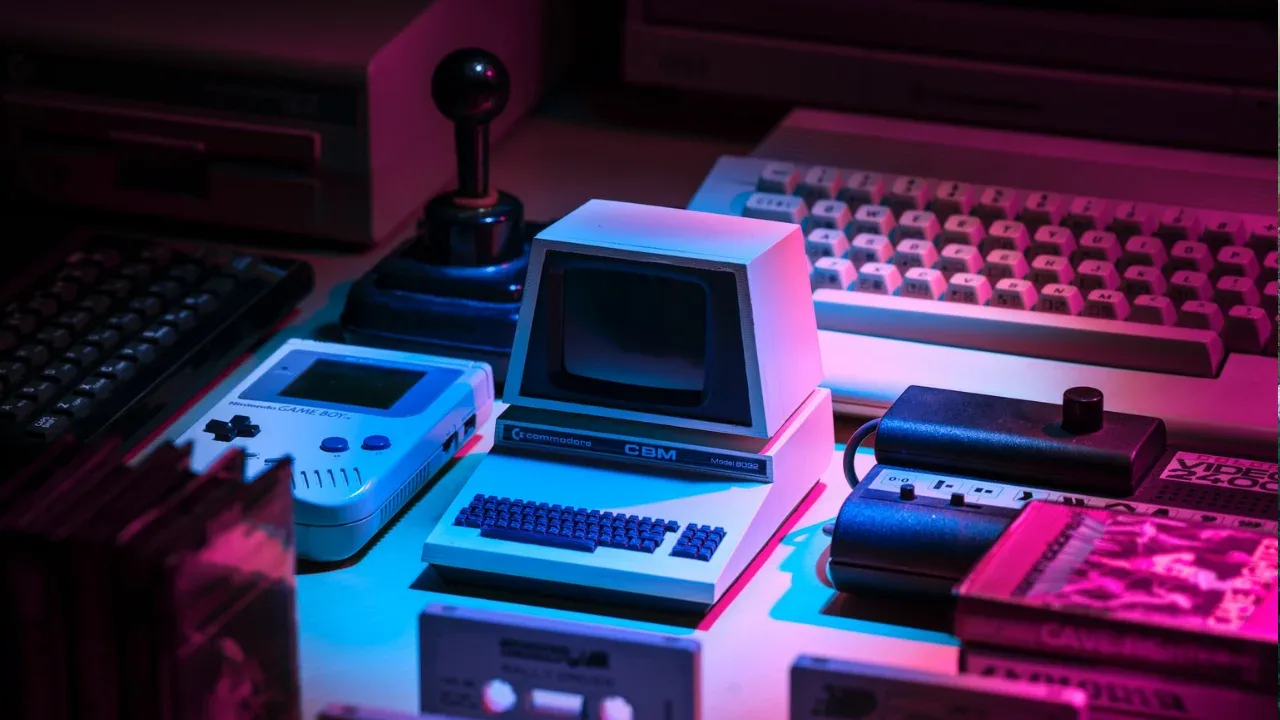
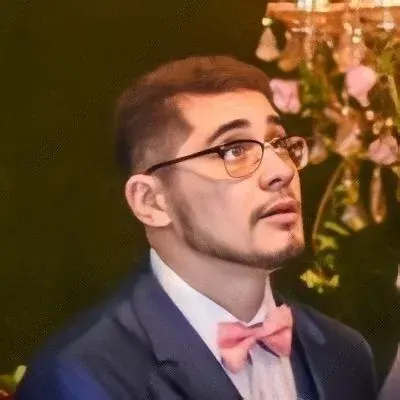
What's the Deal with the '@=' Symbol in Python? 🤔
When exploring the depths of Python programming, we often come across symbols and syntax that leave us scratching our heads. One such symbol is the '@=' symbol, which can be quite puzzling if you haven't encountered it before.
In Python, we are familiar with the '@' symbol as it is commonly used for decorators. Decorators allow us to modify the behavior of functions, classes, or methods without directly modifying their source code. But what about the '@=' symbol? Is it just a placeholder for some mystical feature or a syntax error waiting to happen? Let's dive in and uncover the truth!
The '@=' Symbol... Is It a Thing? 🤔
The truth is, the '@=' symbol is not a valid Python syntax. As of the current version of Python (3.9), there is no official use for the '@=' symbol in the language. So if you stumbled upon this symbol while reading the tokenizer.py
file or elsewhere, it is most likely a typo, an overlooked symbol, or an experimental feature that never made it into the language.
Common Mistakes: Similar-Looking Symbols
It's quite easy to get confused with symbols that look similar to the '@=' symbol. Let's address two such symbols that can be easily mistaken for the elusive '@=' symbol.
1. '@=' vs. '@' - The Decorator Symbol
As mentioned earlier, Python uses the '@' symbol for decorators. Decorators are a powerful way to modify the behavior of functions, classes, or methods. Here's an example of how decorators are commonly used in Python:
def uppercase_decorator(func):
def wrapper():
result = func()
return result.upper()
return wrapper
@uppercase_decorator
def greet():
return "hello, world!"
print(greet()) # Output: HELLO, WORLD!
In the example above, we define a decorator function called uppercase_decorator
that takes a function as an argument and returns a modified version of it. By using the '@' symbol before the function definition, we apply the decorator to the greet
function, altering its behavior. Note that this usage is correct and different from the elusive '@=' symbol.
2. '@=' vs. '==' - Equality Comparison Operator
The '==' symbol is the equality comparison operator in Python. We use it to compare two values and determine if they are equal or not. Here's a simple example:
x = 5
y = 7
if x == y:
print("x is equal to y")
else:
print("x is not equal to y")
In this example, the '==' symbol is used to compare the values of x
and y
. If they are equal, the first block of code executes; otherwise, the second block executes. Remember that '==' checks for equality, whereas '=' is used for variable assignment.
The Quest for '@=' Ends Here
Now that we have explored the possibilities and misconceptions surrounding the '@=' symbol in Python, it's time to put this mystery to rest. Remember, the '@=' symbol is not a valid Python syntax and does not have any standard use within the language.
If you encounter the '@=' symbol, it is advisable to thoroughly review the context and seek clarification from the code's author. Chances are it's a mistake that can be easily corrected.
Join the Conversation! 🎉
Have you ever encountered mysterious symbols or syntax in Python that made you do a double-take? Share your experiences and insights in the comments below! Let's unravel the perplexing world of Python symbols together.
Make sure to follow us on Twitter (@exampleblog) for more Python tips, tricks, and discussions. Happy coding! 😄🐍✨