What is the most efficient way to loop through dataframes with pandas?
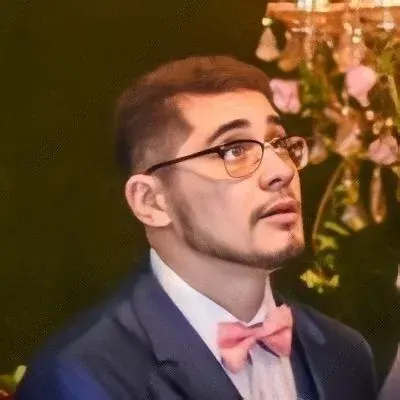
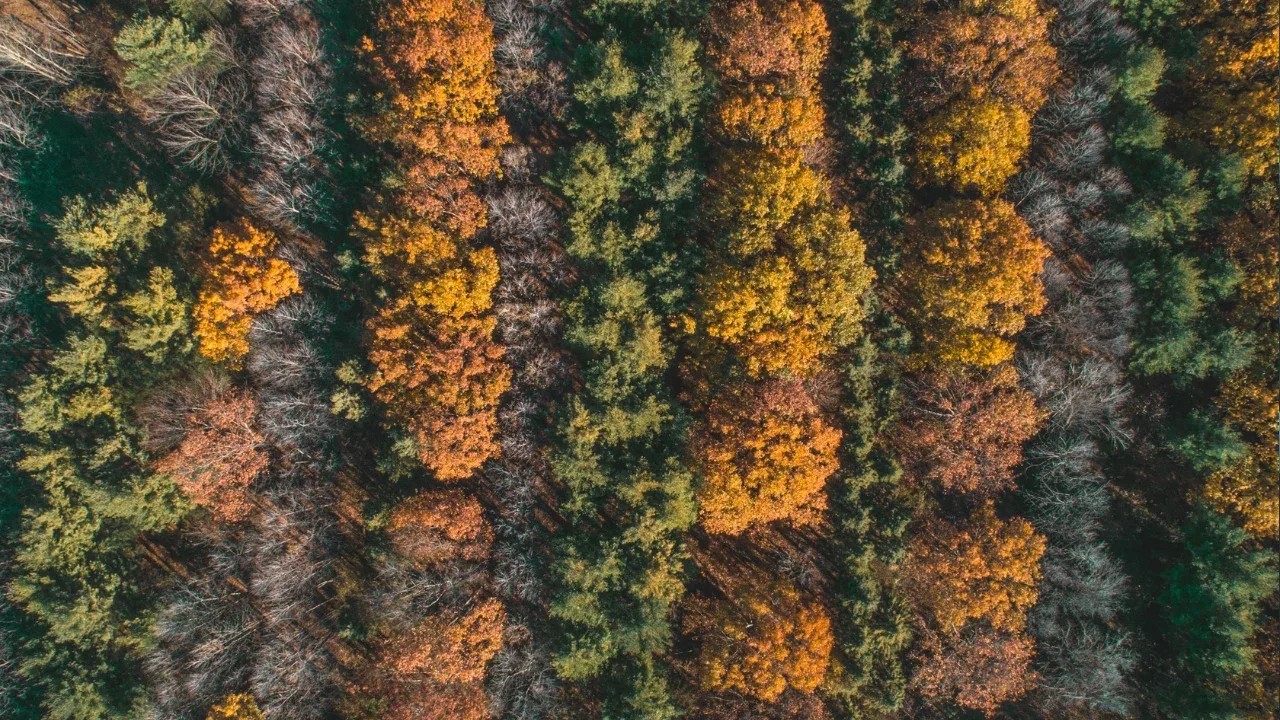
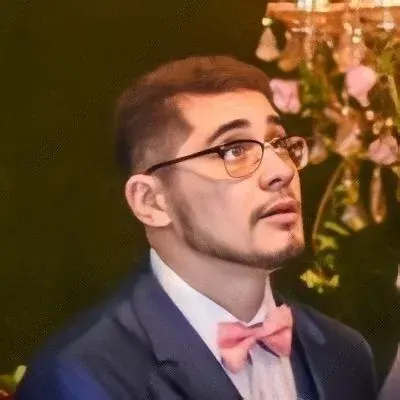
The Most Efficient Way to Loop Through Dataframes with Pandas 🐼
Do you ever find yourself working with large datasets and needing to perform operations on each row in a dataframe? If so, you might be wondering what the most efficient way to loop through dataframes with pandas is. Well, wonder no more because we have the answers you're looking for!
The Common Issue: Slow Looping with Dataframes 😩
Traditional looping methods, like using the iterrows()
function, can be slow and inefficient when working with dataframes in pandas. This is because they have to iterate through each row individually, which adds a lot of overhead and can be time-consuming for large datasets.
The Solution: Vectorized Operations and Built-in Functions 😎
The key to efficient looping with pandas is to leverage vectorized operations and built-in functions. These methods allow you to perform operations on entire columns or rows at once, rather than iterating through them one by one. This can greatly improve the speed and efficiency of your code.
Here are a few examples of how you can use vectorized operations and built-in functions to loop through dataframes more efficiently:
Example 1: Calculating the Moving Average 📈
Let's say you want to calculate the 5-day moving average of the 'Close' column in your dataframe. Instead of looping through each row and calculating the moving average individually, you can use the rolling()
function to perform this operation in a vectorized manner.
df['Moving Average'] = df['Close'].rolling(window=5).mean()
By using the rolling()
function, you can calculate the moving average for each row in just a single line of code. This is much faster and more efficient than traditional looping methods.
Example 2: Applying Custom Functions to Rows 🧑💻
Sometimes, you might need to apply a custom function to each row in your dataframe. Instead of using a traditional loop, you can use the apply()
function to apply the custom function to each row in a vectorized manner.
def custom_function(row):
# perform custom operations on the row
return some_result
df['Custom Result'] = df.apply(custom_function, axis=1)
By using the apply()
function, you can apply the custom function to each row in your dataframe without the need for a traditional loop. This can significantly improve the efficiency of your code.
The Compelling Call-to-Action: Keep Exploring and Be Efficient! 🚀
Now that you know the most efficient way to loop through dataframes with pandas, it's time to put this knowledge into practice. Start exploring your own datasets and look for opportunities to leverage vectorized operations and built-in functions. By doing so, you'll be able to write faster and more efficient code.
Don't stop here - dive deeper into the pandas documentation and explore other built-in functions and techniques that can further optimize your code. The more you learn, the more efficient you'll become as a data analyst or scientist.
So, what are you waiting for? Start looping through dataframes with pandas like a pro and take your data analysis skills to new heights!
Leave a comment below and let us know how you've used these techniques in your own projects. Happy coding! 😊
----------------------------------------------------
Additional Resources:
10 Minutes to Pandas - A quick introduction to using pandas for data analysis