What is the meaning of single and double underscore before an object name?
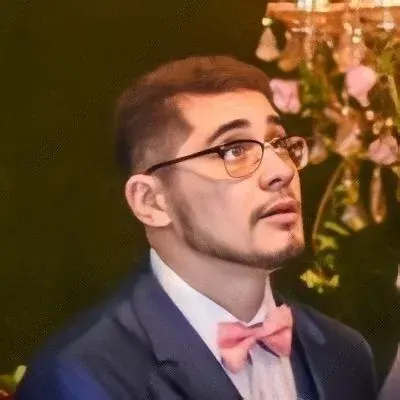
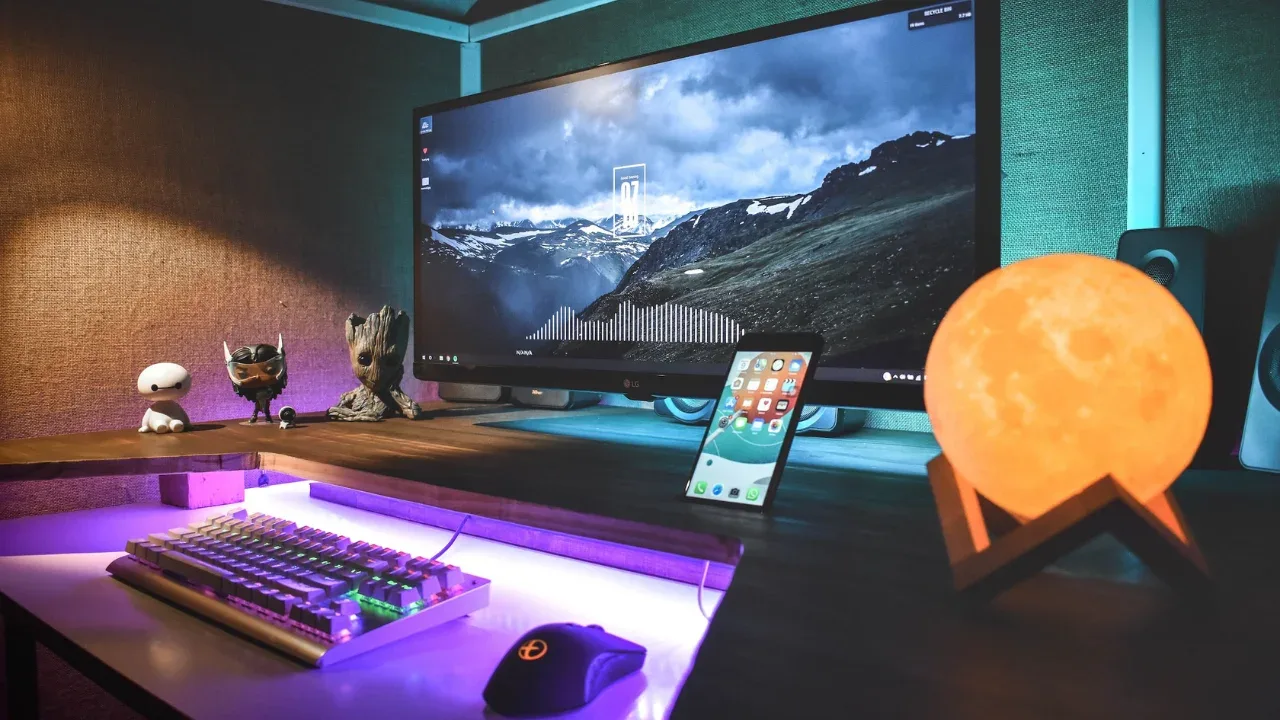
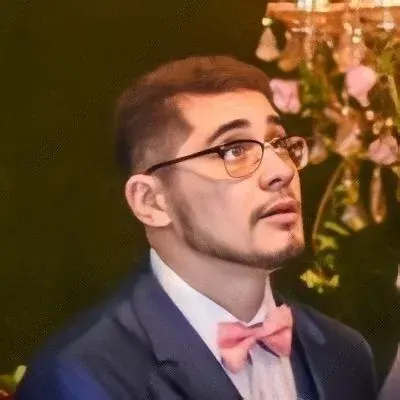
The Magic Behind Single and Double Underscores in Python 🎩✨
If you've been coding in Python for a while, you might have come across code that uses single or double underscores before an object's name. At first glance, these underscores might seem like random decorations, but they actually have a special meaning in Python.
The Mystery of the Single Underscore (_)
When it comes to using a single underscore before an object's name, there are two common scenarios:
1. Ignoring a Variable
Sometimes, you might need to assign a value to a variable, but you have no intention of using that variable later in your code. In such cases, using a single underscore is a Pythonic way to indicate that the variable is irrelevant.
name = "John"
_ = calculate_age(1990)
By convention, using a single underscore tells other developers that the variable can be ignored and is not expected to be referenced again.
2. Storing the Result of the Last Expression in a Python Shell
When you use Python interactively in a shell or a REPL (Read-Eval-Print Loop), a single underscore represents the result of the last expression you executed. It's like a handy memory bank that allows you to access previous results without explicitly assigning them to a variable.
>>> 3 + 5
8
>>> _ * 2
16
Using a single underscore in this context is a convenient way to refer to the previous result without having to type out the actual value.
The Enigma of the Double Underscore (__)
Now let's move on to the more intriguing topic of the double underscore before an object's name. This convention, also known as name mangling, has a specific purpose when used within class definitions.
Whenever you create a variable or a method with double underscores as a prefix, Python automatically adds a class-specific prefix to the name to avoid naming conflicts between different classes.
Here's a simple example to illustrate name mangling in action:
class MyClass:
def __init__(self):
self.__private_variable = 42
my_object = MyClass()
print(my_object.__private_variable)
🔍 When you run this code, you'll encounter an AttributeError
. That's because the double underscore prefix causes Python to "mangle" the variable name, making it inaccessible from outside the class.
To access the mangled variable, you need to use the modified name, which combines the class name and the original variable name:
print(my_object._MyClass__private_variable)
While this name mangling feature can be useful in certain cases, it's important to remember that it is primarily intended for internal class usage. In general, it's considered good practice to respect the naming conventions and not try to access mangled variables directly from outside the class.
A Call-to-Action: Share Your Pythonic Experiences! 🐍🚀
Now that you've unraveled the mysteries behind single and double underscores in Python, it's time to apply this knowledge in your own code.
🔹 Have you come across any situations where you found the use of single or double underscores particularly helpful?
🔹 What are your thoughts on the name mangling technique? Have you ever encountered any pitfalls when using it?
🔹 Feel free to share your thoughts, experiences, or any related tips and tricks in the comments below. Let's ignite a Pythonic discussion! 💬✨
Remember, understanding and embracing these Pythonic conventions will not only make your code more readable but will also help you collaborate more effectively with other Python developers.
Happy coding! 💻🐍