What is the difference between __str__ and __repr__?
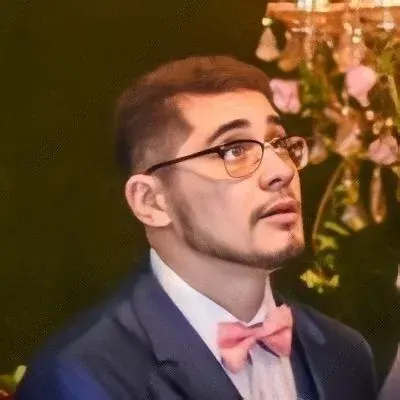
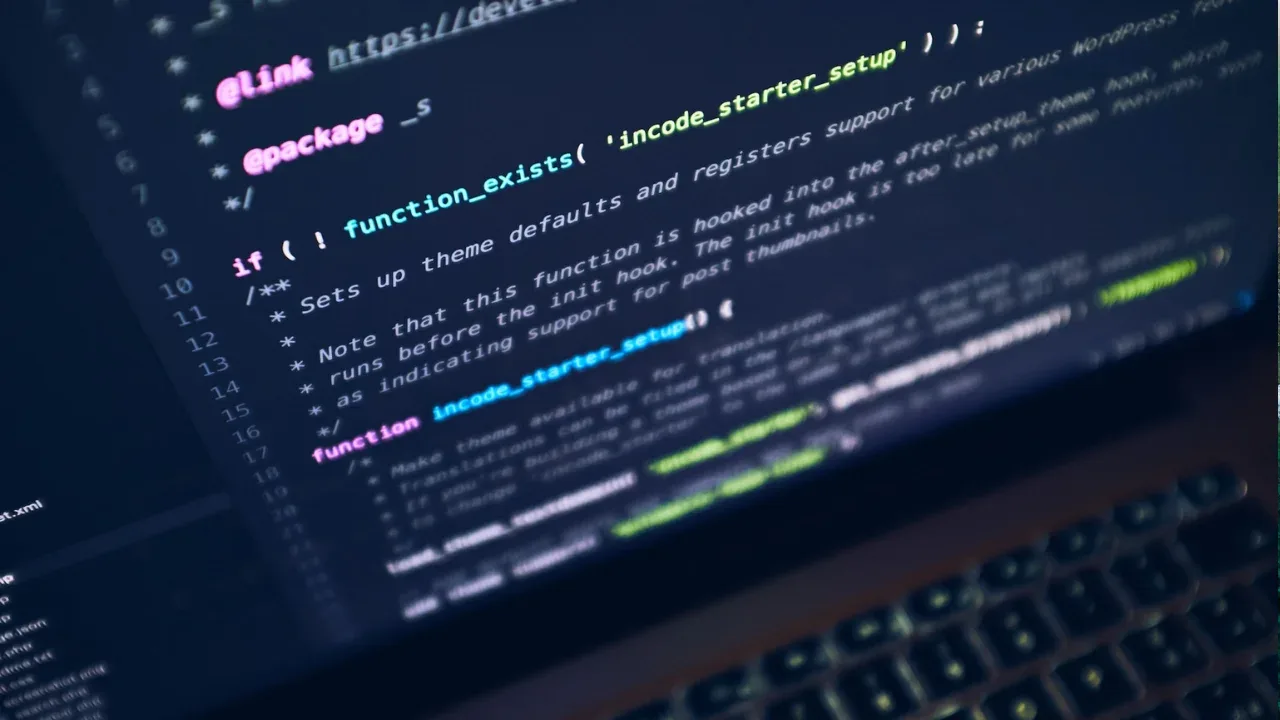
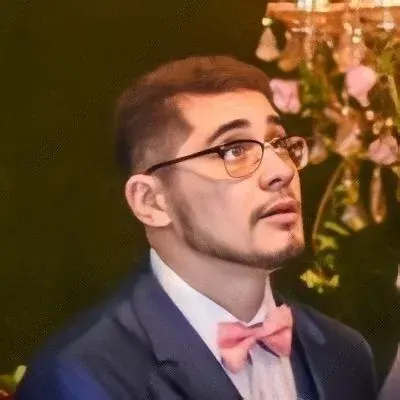
π Python Tips & Tricks: Understanding the Mysterious str and repr π
Are you a Pythonista perplexed with the difference between the enigmatic __str__
and __repr__
?π€ Fear not, my friend! In this guide, we'll unravel the mysteries surrounding these two duos and shed light on their true purpose. Sit tight, grab your coffee βοΈ, and let's dive in!
π§ What's the Big Deal?
As Python programmers, we often encounter situations where we need to represent our objects as strings. That's where __str__
and __repr__
come into play. But what exactly are they, and why should you care?
The answer lies in their distinct functionalities:
1οΈβ£ __str__
: The Human-Friendly Representation
When you want to display a readable string representation of your object, __str__
is your go-to method. Its primary purpose is to provide a user-friendly output. Think of it as a way to create a string representation that a human can easily understand and relate to.
π Example:
class Dog:
def __init__(self, name):
self.name = name
def __str__(self):
return f"Hi, I am {self.name}!"
my_dog = Dog("Buddy")
print(str(my_dog)) # Output: Hi, I am Buddy!
2οΈβ£ __repr__
: The Unambiguous Representation
Unlike __str__
, the purpose of __repr__
is to provide an unambiguous string representation of an object. It aims to be a "formal" representation that can be used to recreate the object if needed. In simpler terms, __repr__
offers a way to represent your object in such a way that Python can evaluate it back to the same object.
π Example:
class Dog:
def __init__(self, name):
self.name = name
def __repr__(self):
return f"Dog('{self.name}')"
my_dog = Dog("Buddy")
print(repr(my_dog)) # Output: Dog('Buddy')
π¨ Pitfalls and Solutions
Now that we understand the differences between __str__
and __repr__
, let's address some common pitfalls:
π§ͺ Pitfall 1: Forgetting to Implement Either Method
If you forget to implement either __str__
or __repr__
in your class, Python will fall back to the default implementations inherited from the object
class. These defaults will provide representations that are neither human-friendly nor unambiguous. π
ββοΈ
π‘ Solution: Implement Both Methods
To avoid this pitfall, it's best to implement both __str__
and __repr__
methods in your class. This way, you can control the string representation of your object for both end-users and potential developers.
π§ͺ Pitfall 2: Inconsistency Between str and repr
Another common issue arises when the string representations returned by __str__
and __repr__
are inconsistent. This can confuse users and lead to unexpected behavior in your code. π΅
π‘ Solution: Keep Them in Sync
To maintain consistency, it's advisable to make the string returned by __repr__
a valid Python expression that, when evaluated, recreates an object with the same state. This will help avoid confusion and ensure predictability.
π Your Turn to Dive In!
Now that you've grasped the essence of __str__
and __repr__
, it's time to level up your Python skills! Embrace these methods in your classes and harness their power to provide meaningful object representations.
If you're curious to learn more about Python's magic methods, check out the official Python documentationβyour ultimate guide to becoming a Pythonic wizard! π§ββοΈ
So, what are you waiting for? Go forth, code fearlessly, and craft beautiful object representations! πͺπ»
π What are your experiences with __str__
and __repr__
? Share your thoughts in the comments below! π
Remember, fellow Pythonistas, understanding the depths of the Python language is what sets us apart. Happy coding! πβ¨