What is the difference between re.search and re.match?
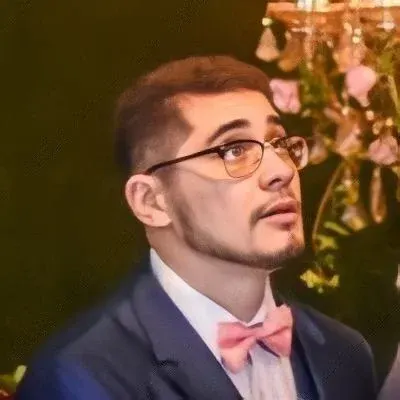
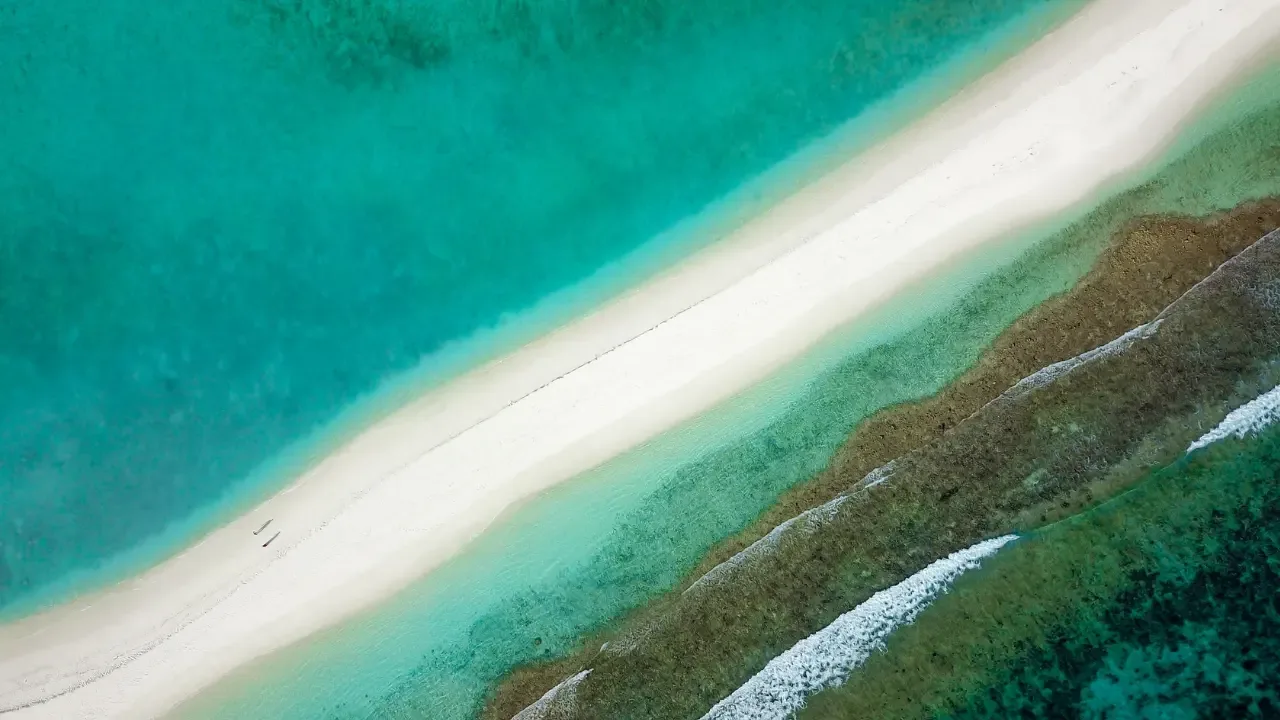
Understanding the difference between re.search and re.match functions in Python 🧐
Have you ever found yourself confused about the difference between the search()
and match()
functions in the Python re
module? Don't worry, you're not alone! This is a common source of confusion for many Python developers. But fear not, because I am here to explain it to you in a clear and simple way that you'll never forget! 💪
The basics 🔍
Before we dive into the differences, let's quickly refresh our memory on what these functions actually do.
re.search(pattern, string, flags=0)
function searches for a pattern anywhere in the string and returns a match object if found.re.match(pattern, string, flags=0)
function checks if the pattern matches the beginning of the string and returns a match object if found.
The key difference 🗝️
The main difference between the two functions lies in the way they match the pattern against the string. Let's take a closer look at each one.
re.search()
The search()
function searches for a given pattern in the entire input string. It returns the first occurrence of the pattern that it finds, regardless of where it is located within the string. This means that even if the pattern is found in the middle or at the end of the string, search()
will still find it. 🎯
re.match()
On the other hand, the match()
function only checks if the pattern matches the beginning of the string. It will return a match object only if the pattern is found at the very start of the string. If the pattern is found elsewhere in the string, match()
will not find it and return None
. So, in order to get a match object using match()
, the pattern must be found at the beginning of the string. 🎯
Let's illustrate with an example 💡
To truly understand the difference, let's see how these functions behave with a simple example.
import re
pattern = r"hello"
string = "hello world"
print(re.search(pattern, string)) # <re.Match object; span=(0, 5), match='hello'>
print(re.match(pattern, string)) # <re.Match object; span=(0, 5), match='hello'>
In this example, both search()
and match()
functions find a match because the pattern "hello" exists at the beginning of the string. But what happens if the pattern is not at the start?
import re
pattern = r"world"
string = "hello world"
print(re.search(pattern, string)) # <re.Match object; span=(6, 11), match='world'>
print(re.match(pattern, string)) # None
Here, search()
successfully finds the pattern "world" in the middle of the string, while match()
returns None
because the pattern is not found at the beginning.
Solutions and best practices ✅
Solution 1️⃣: Use search()
when you're unsure about the pattern's position
If you're not sure where the pattern might be in the string, or if it can appear anywhere, it's best to use search()
. This function will find the first occurrence of the pattern, no matter where it is located.
Solution 2️⃣: Use match()
when the pattern must appear at the start
On the other hand, if you specifically need to match the pattern only at the beginning of the string, use match()
. This function is ideal when you are looking for patterns that must start the string.
A call-to-action for you! 📢
Now that you have a clear understanding of the differences between search()
and match()
, it's time to put your knowledge to the test! Share your thoughts in the comments below and let us know which function you find more useful in your Python projects 🚀
Remember, understanding these small but crucial differences can save you a lot of time and headaches in your coding journey. Keep coding, keep learning, and keep being awesome! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
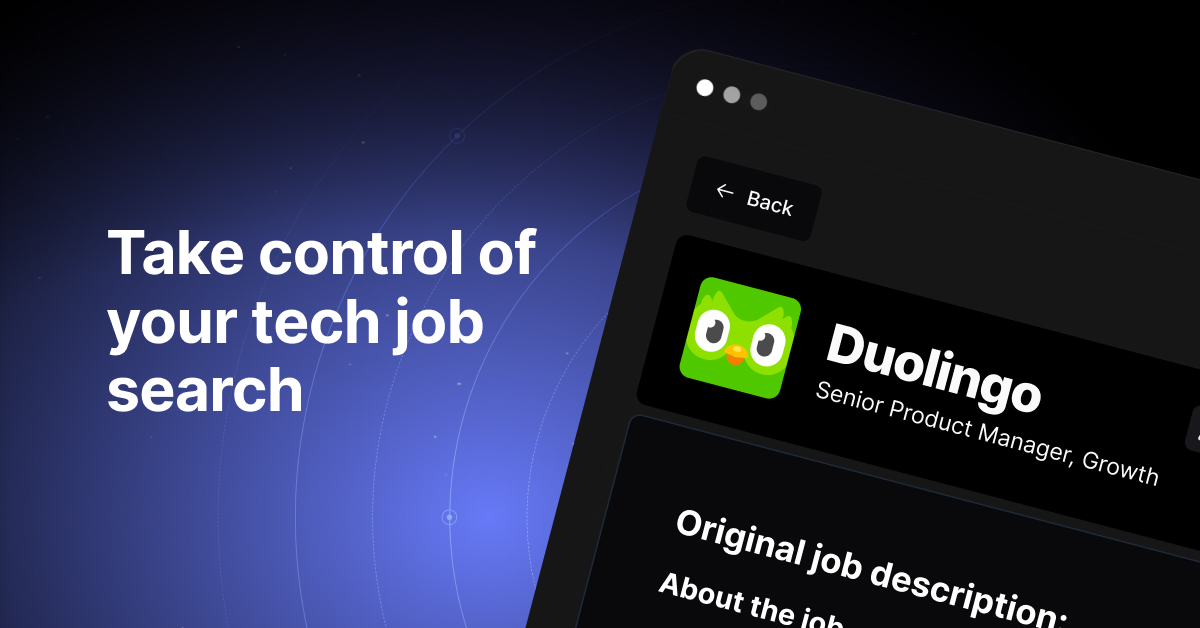