What is the difference between json.load() and json.loads() functions
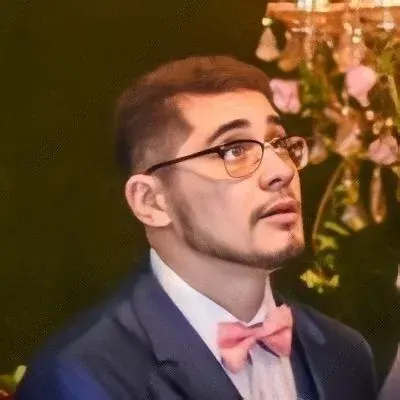
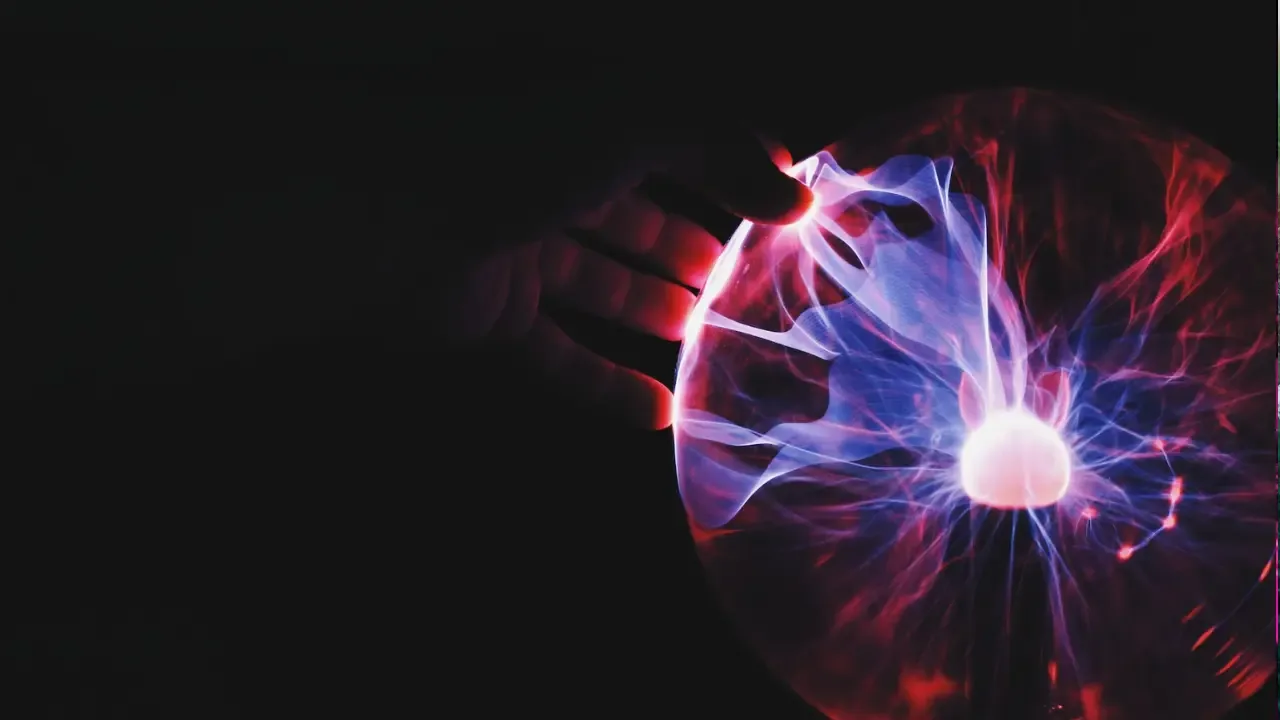
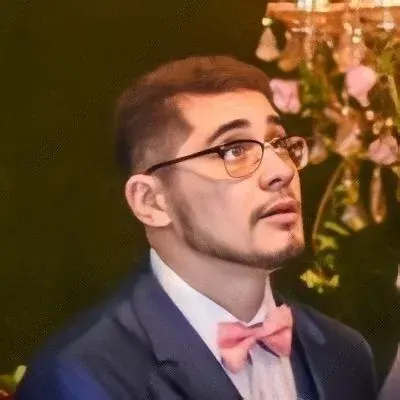
📝 The Ultimate Guide to json.load() vs json.loads() Functions 📝
Hey there! 😄 Are you facing confusion while working with Python's json.load()
and json.loads()
functions? Don't worry, I've got you covered! 🙌
Let's dive into the key differences between these two functions and clarify any common issues that may arise. By the end, you'll understand when to use which function without any confusion! 💡
The Basics: json.load() 📥
The json.load()
function is used to read JSON data from a file object. It's perfect when you have a JSON file and want to extract its data for further processing. Here's a simple example:
import json
with open('data.json') as file:
data = json.load(file)
print(data)
In this example, we open the data.json
file using a context manager and then pass the file object to the json.load()
function. The function reads the JSON data from the file and stores it in the data
variable.
The Trick: json.loads() 🎩
On the other hand, json.loads()
is used to convert a JSON-formatted string into a Python object. So if you have a JSON string and want to work with its data in your code, this is the function you're looking for. Take a look at the following snippet:
import json
json_string = '{"name": "John", "age": 30}'
data = json.loads(json_string)
print(data)
In this example, we have a JSON string assigned to json_string
. By calling json.loads()
, we convert it into a Python object, and the resultant data is stored in the data
variable. Voila! 🎉
Understanding the 's': json.loads() 🤔
Now, let's address your confusion about the 's' in json.loads()
. You hit the nail on the head! The 's' indeed stands for 'string' because this function expects a JSON string as its input.
Using json.loads()
is like telling Python, "Hey Python, take this JSON string and make it usable for me!" 😄
Common Issues and Solutions 💡
Issue 1: FileNotFoundError
Sometimes, when working with json.load()
, you might encounter a FileNotFoundError
if you pass an incorrect file path or the file doesn't exist. To avoid this, double-check your file path and make sure the file exists at the specified location.
Issue 2: JSONDecodeError
When using json.loads()
, a JSONDecodeError
can occur if the JSON string you provide is not valid. To handle this, ensure your JSON string is properly formatted, with correct braces, commas, and key-value pairs.
Share Your Thoughts! 📣
I hope this guide has helped you understand the difference between json.load()
and json.loads()
functions. Now, it's your turn to put this knowledge into practice! 💪
Have you ever encountered any challenges with these functions? What was your favorite solution from this guide? Share your thoughts in the comments below and let's discuss! 🗣️
And don't forget to share this post with your friends who might find it useful too. Happy coding! 😊✨