What is the difference between join and merge in Pandas?
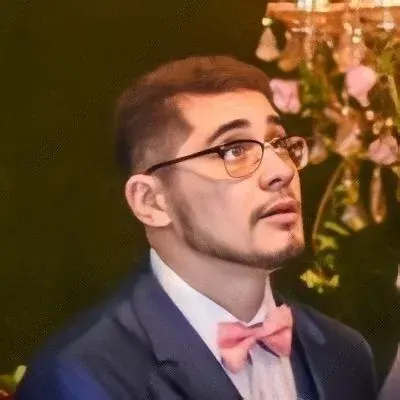
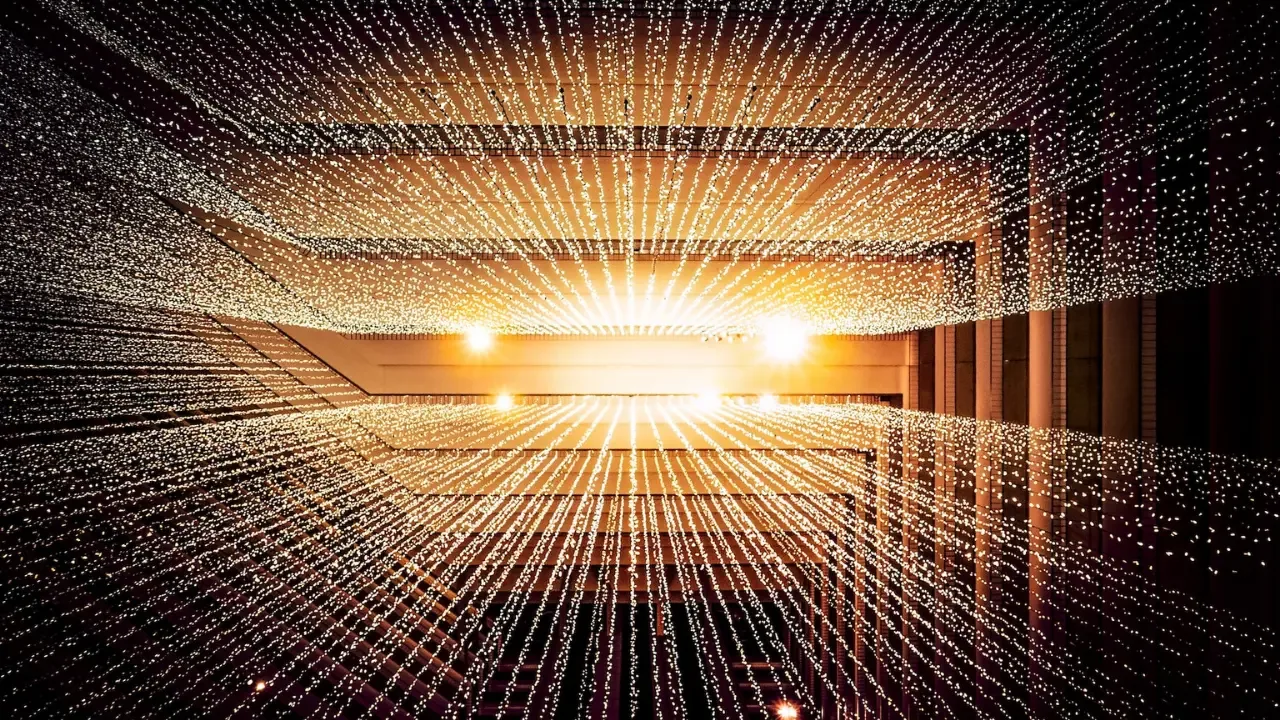
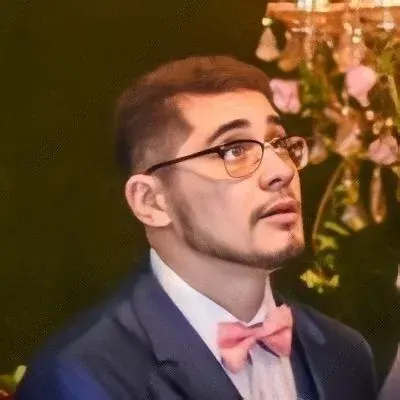
📝🔍 The Difference Between Join and Merge in Pandas: Explained with Examples!
Have you ever wondered what the difference is between the join and merge functions in Pandas? You're not alone! These two functions may seem similar at first glance, but in reality, they have a few key differences that can lead to different results. In this blog post, we'll dive deep into the differences and provide easy solutions to commonly encountered issues. So, let's get started! 🚀
The Scenario
To better understand the differences between join and merge, let's consider a scenario. Imagine we have two DataFrames:
left = pd.DataFrame({'key1': ['foo', 'bar'], 'lval': [1, 2]})
right = pd.DataFrame({'key2': ['foo', 'bar'], 'rval': [4, 5]})
The Merge Function
First, let's take a look at the merge function. You might be using it like this:
pd.merge(left, right, left_on='key1', right_on='key2')
And you get the desired result:
key1 lval key2 rval
0 foo 1 foo 4
1 bar 2 bar 5
🔑 The merge function takes two DataFrames as input and combines them based on specified column names (left_on
and right_on
). It uses these column names to match and merge the rows from both DataFrames into a single result DataFrame.
The Join Function
Now, let's move on to the join function. In your case, you tried using it like this:
left.join(right, on=['key1', 'key2'])
But instead of the desired result, you encounter an error:
AssertionError
🔑 Here's the catch: The join function is meant to merge DataFrames using their indexes, not specific column names like merge does. In your example, you specified columns key1
and key2
using the on
parameter. However, join expects you to set these columns as indexes beforehand.
The Solution
To make the join function work in this scenario, you need to set the columns as indexes before joining. Here's how you can do it:
left = left.set_index('key1')
right = right.set_index('key2')
left.join(right)
And you get the desired result again:
lval rval
key1
foo 1 4
bar 2 5
By setting the key1
column as the index for the left
DataFrame and the key2
column as the index for the right
DataFrame, you ensure that the join function merges the DataFrames based on these indexes.
Conclusion
In summary, the merge function in Pandas combines DataFrames based on specified column names, while the join function merges DataFrames based on their indexes. By understanding this distinction, you can avoid confusion and choose the appropriate function for your needs.
If you found this blog post helpful, don't hesitate to share it with your fellow data enthusiasts! And if you have any further questions or want to share your own experiences with join and merge, feel free to leave a comment below. Let's keep the discussion going! 🎉💬