What is the correct syntax for "else if"?
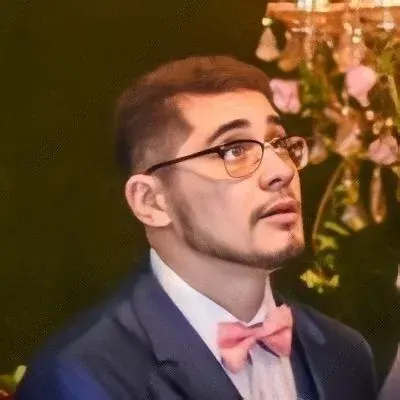
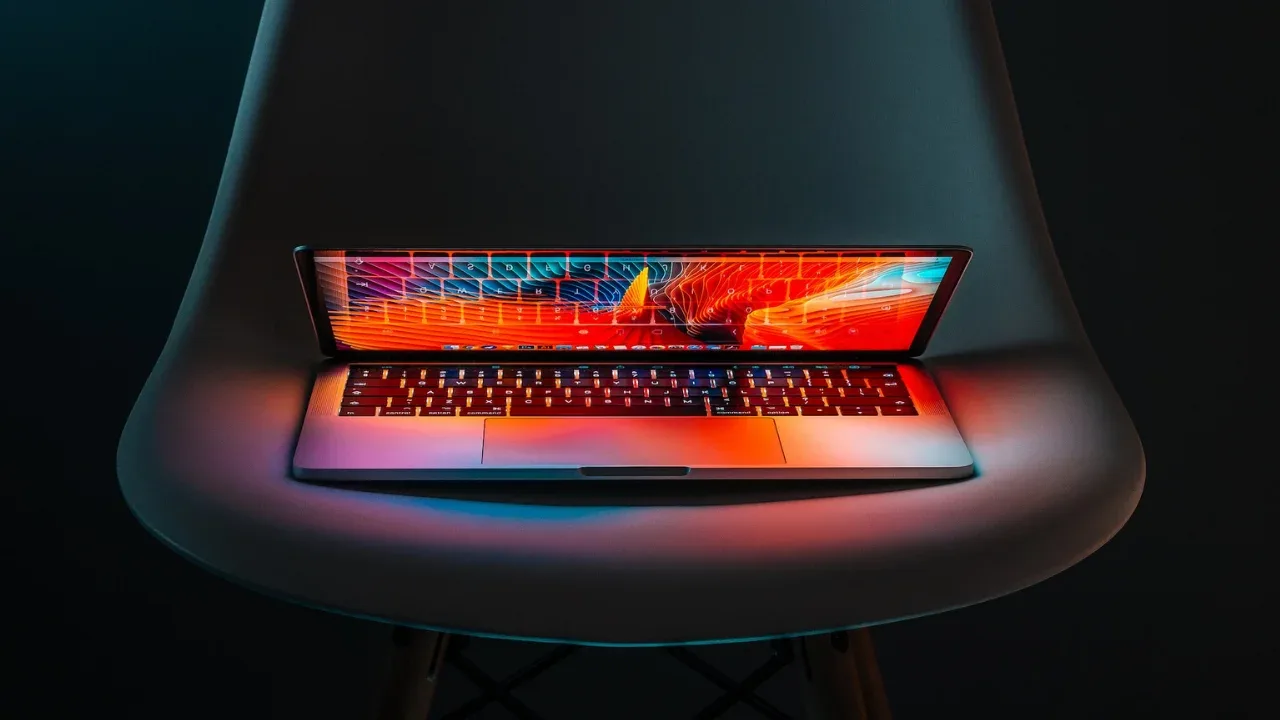
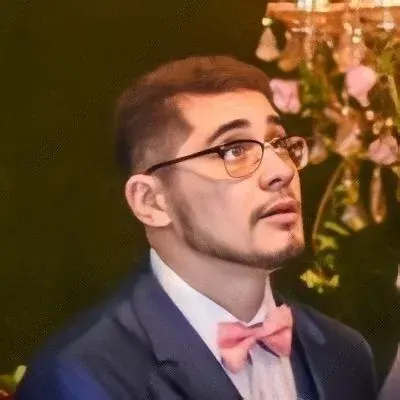
The Syntax of "else if" in Python 🐍
If you're a new Python programmer transitioning to a newer version, you may encounter syntax errors or face difficulties while using the "else if" statement. Don't worry, though! This blog post will guide you through understanding the correct syntax, explain the common issues, and offer easy solutions to get you on the right track. Let's dive in! 💻
Understanding the Issue 🔍
The syntax error mentioned in the question arises from a misunderstanding of Python syntax. Unlike some other programming languages, Python doesn't have a specific "else if" statement. Instead, Python uses the "elif" keyword to handle multiple conditional checks. Let's look at an example:
def function(a):
if a == '1':
print('1a')
elif a == '2':
print('2a')
else:
print('3a')
function(input('input:'))
Correcting the Syntax ✅
Here's what you need to keep in mind to correct the syntax:
Replace "else if" with "elif" for each subsequent condition you want to check.
Use a colon (:) at the end of each "if" and "elif" statement.
Indent the code block inside each "if" or "elif" statement for proper code structure.
If none of the conditions match, you can use "else:" followed by a colon and the desired code block.
Explaining the Example 📝
Let's break down the corrected example to understand it better:
def function(a):
if a == '1':
print('1a')
elif a == '2':
print('2a')
else:
print('3a')
function(input('input:'))
The function
function(a)
takes an input and compares it to different values using conditional statements.The first "if" statement checks if the input is equal to '1'. If true, it executes the code block
print('1a')
.If the first condition is not met, it moves on to the "elif" statement. This statement checks if the input is equal to '2'. If true, it executes the code block
print('2a')
.Finally, if neither of the previous conditions is met, the "else" statement is triggered, and it executes the code block
print('3a')
.
Engaging with the Community 🤝
At times, even the simplest syntax can be tricky, and it's normal to ask for help! If you're having trouble with any Python-related question or want to share some tricks, join our community forum at example.com/community. We have Python enthusiasts and experts ready to assist you and make your journey smoother. Together, we can level up our Python skills! 🚀
That's it for understanding the "else if" syntax in Python! Remember to use "elif" instead, follow the correct structure, and have fun with your Python coding adventures! Happy coding! 😄✨