What is the best way to remove accents (normalize) in a Python unicode string?
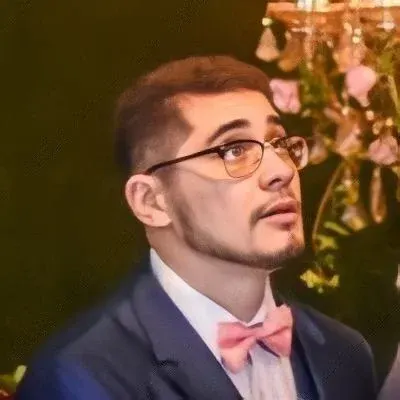
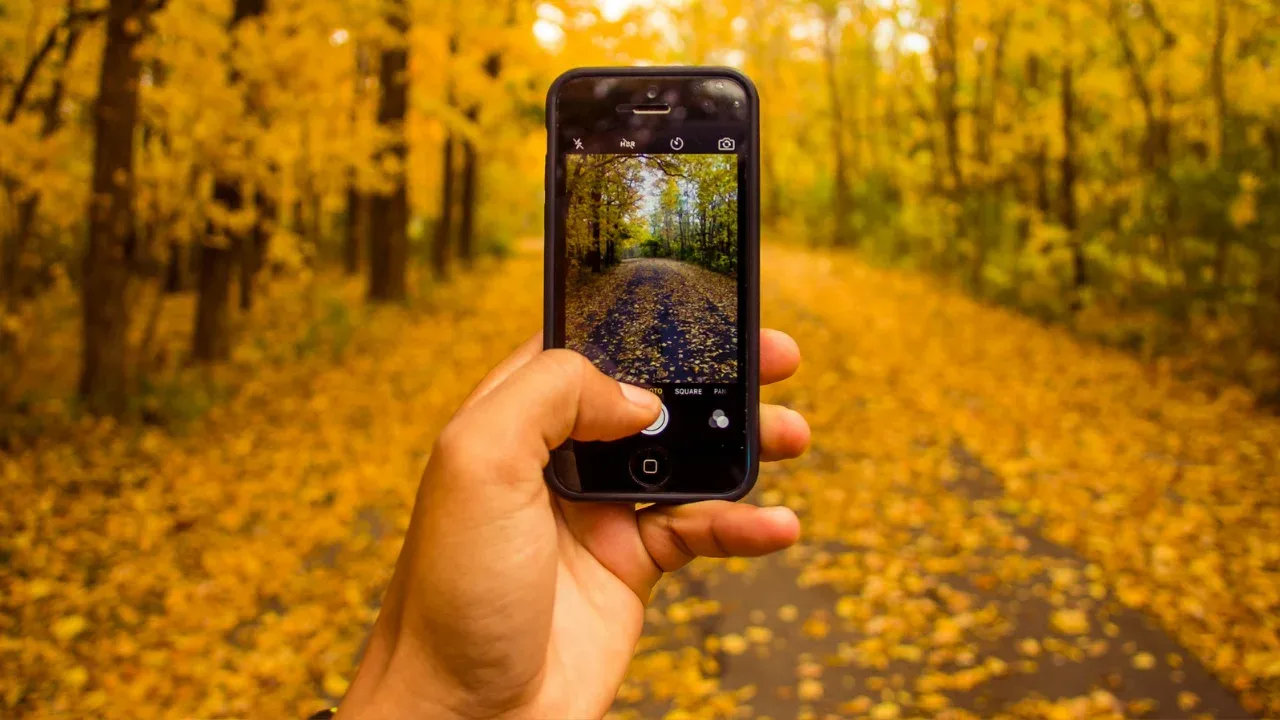
Best Way to Remove Accents in Python Unicode Strings
š„ Want to remove all those pesky accents (diacritics) from your Python Unicode string? Say no more! In this blog post, we'll explore the best approaches to tackling this common issue, providing you with easy and elegant solutions that will leave your code looking clean and efficient. š
The Challenge
You've got a Unicode string in Python, and you want to get rid of those accents. No more worrying about special characters messing up your data or causing compatibility issues. But how should you go about it? š¤
Solution 1: The Long Normalized Form
One way to achieve this is by converting your Unicode string to its long normalized form. This form represents each letter and diacritic as separate characters, making it easier to identify and remove the diacritics.
Here's how you can do it:
Import the
unicodedata
module from the Python standard library.import unicodedata
Use the
normalize()
function to convert your string to its long normalized form using the'NFD'
normalization form.normalized_string = unicodedata.normalize('NFD', your_unicode_string)
Remove all characters whose Unicode type is "diacritic" by filtering them out using a list comprehension.
without_accents = ''.join(c for c in normalized_string if unicodedata.category(c) != 'Mn')
And just like that, your string is now free from any accents! š
Solution 2: Python 3 and unicodedata2
If you're working with Python 3, you can take advantage of the unicodedata2
library. This library offers additional features and improvements over the standard unicodedata
module, making it an excellent choice for handling Unicode data effectively.
To remove accents using unicodedata2
, follow the steps below:
Install the
unicodedata2
library using pip:pip install unicodedata2
Import the
normalize
function fromunicodedata2
.from unicodedata2 import normalize
Normalize your Unicode string using the
'NFD'
normalization form.normalized_string = normalize('NFD', your_unicode_string)
Remove all diacritic characters by filtering them out.
without_accents = ''.join(c for c in normalized_string if unicodedata2.category(c) != 'Mn')
Easy-peasy! You've successfully normalized your string and bid adieu to those fancy accents. šŖ
Avoiding Explicit Character Mappings
We understand the importance of keeping your code clean and efficient. That's why both of these solutions avoid using explicit mappings from accented characters to their non-accented counterparts. By leveraging the power of Unicode normalization, you can remove accents with elegance and simplicity. š
Now, you might be wondering, do I need to install a library like pyICU
? The answer is no! Both of the solutions presented here utilize the Python standard library (unicodedata
and unicodedata2
), so you won't need any additional dependencies.
Get Rid of Accents and Level Up Your Code!
Removing accents in Python Unicode strings is now a breeze, thanks to these easy and effective solutions. Start cleaning up your data, eliminating compatibility issues, and unlocking new possibilities in your projects. š„
Have you encountered other challenges with Python or Unicode? Share your experiences and insights in the comments below! Let's learn from each other and create better, more inclusive code together. š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
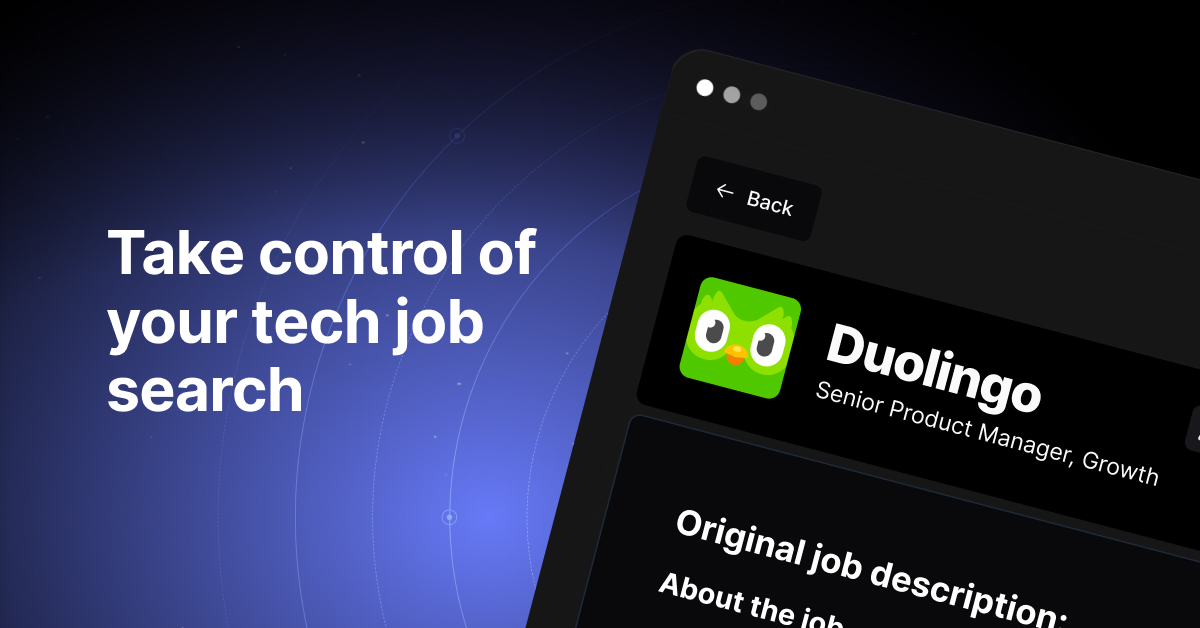