What is sys.maxint in Python 3?
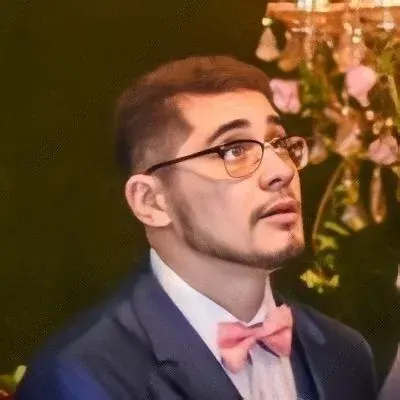
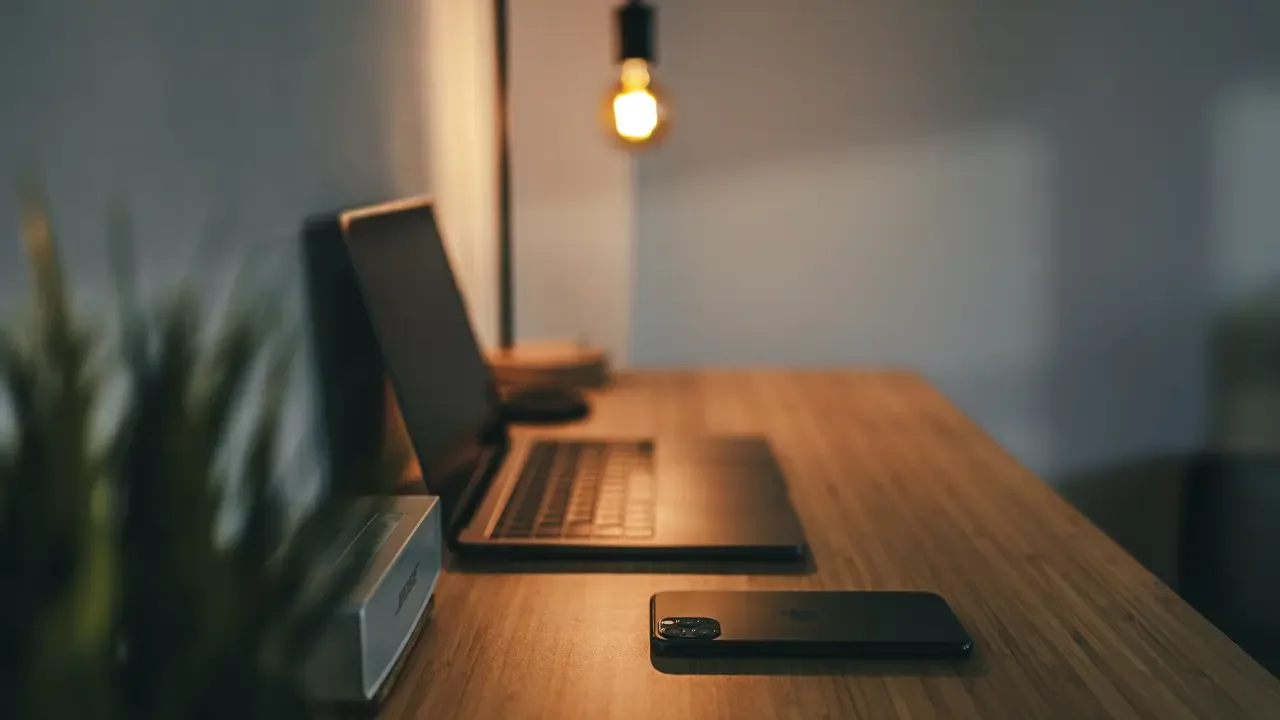
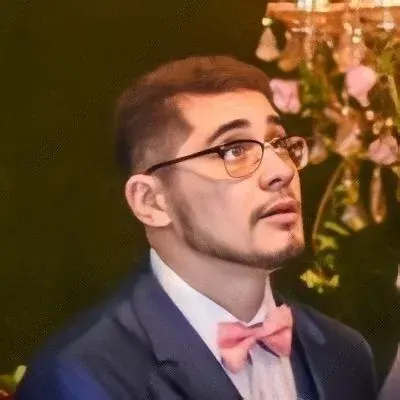
Understanding sys.maxint in Python 3 π
If you've stumbled upon the error message AttributeError: module 'object' has no attribute 'maxint'
when trying to use the sys.maxint
attribute in Python 3, don't panic! This blog post will explain what sys.maxint
is, why it doesn't exist in Python 3, and offer alternative solutions for representing maximum integers. Let's dive in! π₯
What is sys.maxint
? π€
In Python 2.x, the sys.maxint
attribute was used to represent the largest possible integer value. It provided a convenient way to access the maximum integer the Python interpreter could handle. Developers commonly used it as a quick way to determine the limits of integer values.
However, in Python 3, the sys.maxint
attribute was removed and replaced with a more descriptive and intuitive alternative. The removal of sys.maxint
was done to promote better code readability and clarity. π
The Error: 'AttributeError: module 'object' has no attribute 'maxint'' β
If you encounter the error message AttributeError: module 'object' has no attribute 'maxint'
when trying to use sys.maxint
in Python 3, it's because this attribute is no longer available.
Alternative Solutions π‘
To represent the maximum integer value in Python 3, you have a few options:
1. Using sys.maxsize
: π’
Instead of sys.maxint
, you can use sys.maxsize
. This attribute represents the largest positive integer that can be used as an index for sequences (e.g., lists, tuples) or arrays in Python.
import sys
print(sys.maxsize) # Prints the maximum integer value for your system
2. Using float('inf')
: βΎοΈ
Another way to represent an infinite or maximum integer value in Python is by using the float('inf')
float representation of infinity. Although it isn't an actual integer, it can be used in certain calculations or comparisons where a maximum integer value is needed.
maximum_integer = float('inf')
# Example use case: comparing against other integers
if some_integer > maximum_integer:
print("This number is larger than the maximum!")
3. Using math.inf
(requires import math
): π
Similar to the previous option, you can also use the math.inf
constant from the math
module to represent an infinite or maximum integer value. Remember to import the math
module before using it.
import math
maximum_integer = math.inf
# Example use case: checking if a value is infinite
if some_value == maximum_integer:
print("This value is infinitely large!")
Conclusion and Call-to-Action π
So, there you have it! In Python 3, the sys.maxint
attribute no longer exists, but you can still represent maximum integer values using alternatives like sys.maxsize
, float('inf')
, or math.inf
.
Next time you encounter the AttributeError: module 'object' has no attribute 'maxint'
error, you'll know how to handle it like a pro! If you found this post helpful, be sure to share it with your fellow Python enthusiasts and let's spread the knowledge.
Do you have any Python questions or topics you'd like us to cover? Let us know in the comments below! Happy coding! ππ»