What is a "slug" in Django?
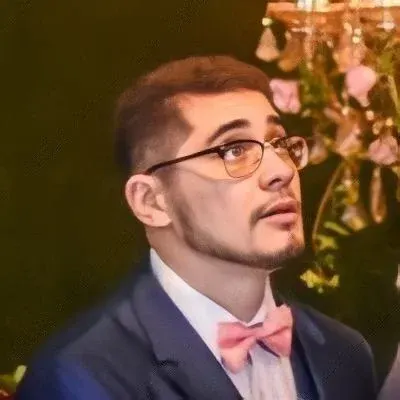
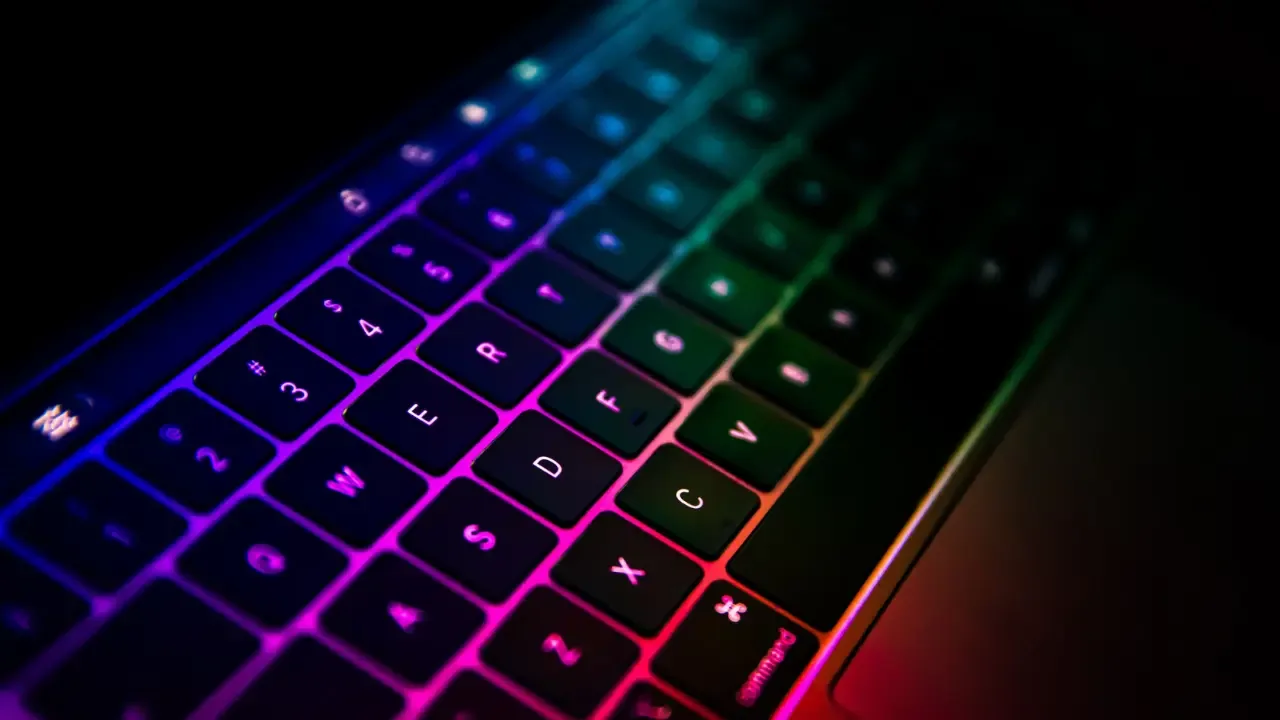
Title: Demystifying Django Slugs: Everything You Need to Know About URL Labels
Introduction: 🌟 Welcome to my blog where we dive into the world of Django! Today, we'll be unraveling the mystery behind a commonly used term - "slug." 😮 If you've ever stumbled upon this term while reading Django code and found yourself scratching your head, fret not! In this post, we'll walk you through what a slug is, how it is used in Django, and provide practical solutions to common issues. Let's get started! 🚀
What is a Slug? 🐌 A slug is a short label used to identify and categorize content, specifically within URLs. It contains only letters, numbers, underscores, or hyphens. Slugs are generally used for improved SEO and user-friendly URLs. Instead of long and cryptic URLs, slugs provide meaningful and human-readable information.
Example Use Case: 🖋️
Consider a typical blog entry URL: https://www.exampleblog.com/best-blog-post-ever/
. In this case, "best-blog-post-ever" is the slug. The slug allows the URL to convey the content's nature and makes it easier for users to navigate and remember.
Using Slugs in Django: 💡 Django provides built-in functionality for handling slugs. Slugs are commonly used in Django models to create URL-friendly representations of object fields. By leveraging Django's slug field, you can automatically generate and manage slugs.
Step 1: Adding a SlugField to a Model: ✍️ To start using slugs, add a SlugField to the model representing the content you want to generate slugs for. For example, in a blog post model, you might have:
from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=200)
slug = models.SlugField()
content = models.TextField()
# ...
Step 2: Generating and Populating Slugs: 🏭 Next, you'll need to ensure that the slug field is automatically populated based on the object's title or another relevant attribute. Thankfully, Django provides a convenient way to do this using prepopulated_fields and pre_save signals.
from django.db.models.signals import pre_save
from django.dispatch import receiver
from django.utils.text import slugify
@receiver(pre_save, sender=BlogPost)
def generate_slug(sender, instance, **kwargs):
if not instance.slug:
instance.slug = slugify(instance.title)
# ...
Step 3: Implementing URL Routing: 🛣 Once you have the slug field in place, it's time to configure Django's URL routing to handle slugs. This involves mapping the URL pattern to the corresponding view and retrieving the object based on the provided slug.
from django.urls import path
from .views import BlogPostDetailView
urlpatterns = [
path('blog/<slug:slug>/', BlogPostDetailView.as_view(), name='blog-post'),
# ...
]
Common Issues and Easy Solutions: 🔎💡
Duplicate Slugs: If you encounter duplicate slug values, consider appending a unique identifier to make them distinct. For example, appending a timestamp or a random string.
Slugifying Non-ASCII Characters: Django's
slugify()
function may not handle non-ASCII characters correctly by default. To handle this, use third-party libraries likepython-slugify
or define a custom slugify function.
Call-to-Action: 📣 Now that you have a solid grasp on slugs in Django, it's time to implement them in your own projects! Start by adding a slug field to a relevant model and experiment with Django's slug functionality. Share your experiences or any questions you have in the comments below. Let's slug it out together! 💪
Conclusion: 🎉 Slugs may seem a bit mystical at first, but they are a powerful tool for creating clean and user-friendly URLs in Django. By following the steps outlined in this guide, you can start harnessing the power of slugs in your own projects. Remember, slugs are your ticket to SEO optimization and an improved user experience. Happy slugging, fellow Django developers! 🐍💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
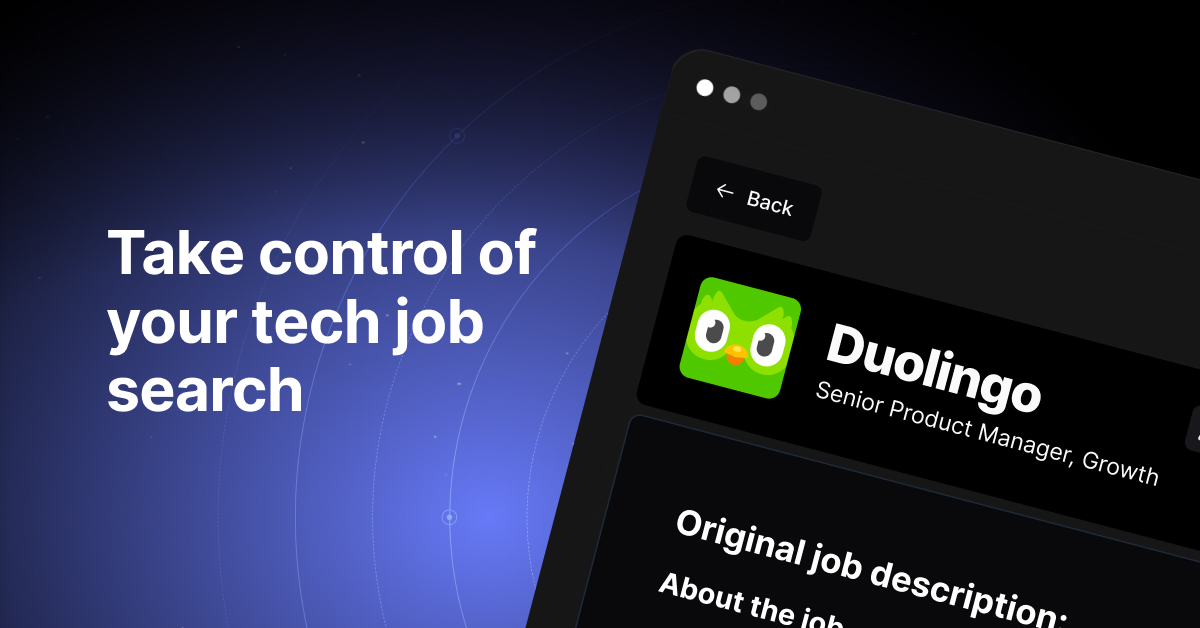