What is a mixin and why is it useful?
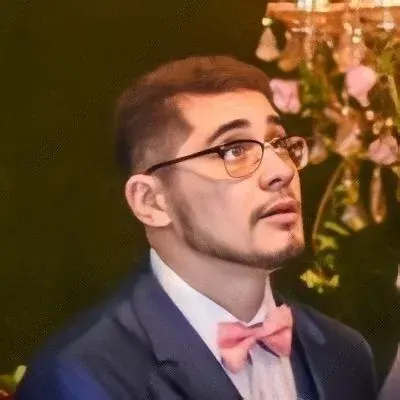
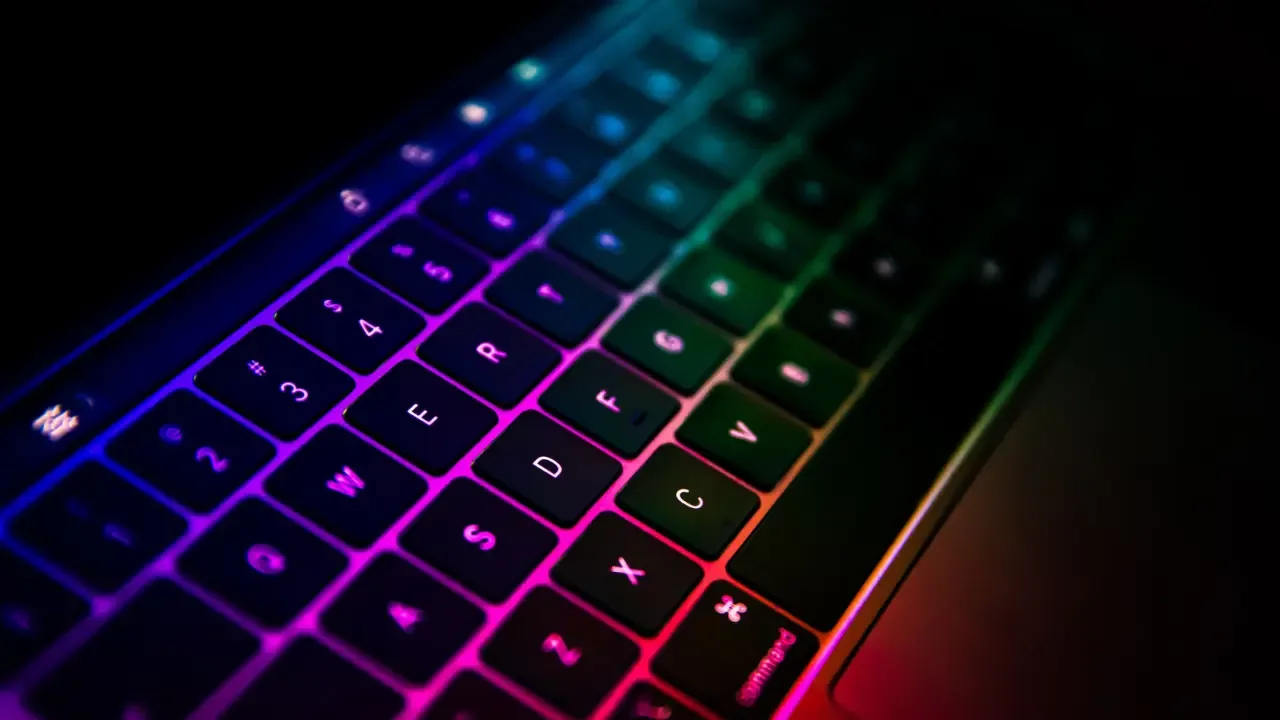
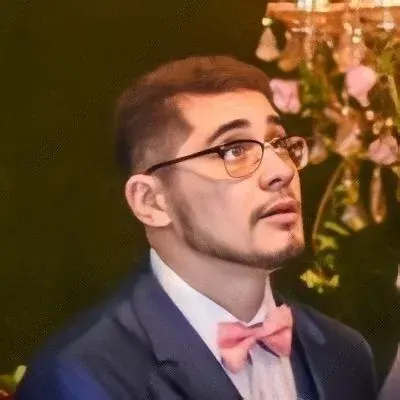
Understanding Mixins: Unlocking the Power of Multiple Inheritance 🔓💪
Have you ever come across the term "mixin" in the context of programming and wondered what it actually means? 🤔 Well, you're not alone! Many developers from different backgrounds, including C/C++/C#, have encountered this term for the first time and were left scratching their heads. In this blog post, we'll demystify the concept of mixins and explore why they are useful in the world of programming. Let's dive in! 💦
What is a Mixin? 🤔
In simple terms, a mixin is a way to reuse code across multiple classes without the need for traditional subclassing. It allows you to add functionality to a class by "mixing in" methods and attributes from one or more other classes. Essentially, a mixin acts as a building block of code that enhances the capabilities of a class without modifying its core functionality.
Mixins vs. Multiple Inheritance: 🤝
You might be wondering, "Isn't mixin just another fancy term for multiple inheritance?" 🤷 Well, not exactly. While mixins and multiple inheritance share some similarities, they are not entirely the same thing.
In multiple inheritance, a class can inherit from multiple parent classes, thus inheriting their attributes and behaviors. On the other hand, mixins are smaller, more granular pieces of code that are meant to enhance a class's functionality without creating a new class hierarchy. Think of mixins as "add-ons" that can be mixed into different classes.
Why Use Mixins? 🌟
Now that we know what mixins are, let's explore why they are so useful and why you might want to use them in your code. Here are a few compelling reasons:
1. Code Reusability ♻️
Mixins promote code reuse by encapsulating specific functionality that multiple classes can benefit from. Instead of rewriting the same code in multiple classes, you can define a mixin once and use it wherever needed. This approach not only saves you time and effort but also reduces code duplication and improves maintainability.
2. Flexibility and Modularity 🧩
By using mixins, you can modularize and separate different aspects of a class's functionality. This allows you to mix and match different mixins to create new classes with custom functionality tailored to your specific needs. It gives you the flexibility to create highly specialized classes without cluttering your codebase or bloating your class hierarchy.
3. Enhancing Existing Classes 🚀
Mixins allow you to enhance existing classes without modifying their core functionality. This can be particularly useful when you want to add new features or behaviors to a class that you don't have control over or that is part of a third-party library. By using mixins, you can extend the functionality of these classes without the need for subclassing or modifying their source code.
4. Promoting Clean and Readable Code 📖
Mixins can help promote clean and readable code by separating different concerns into distinct mixins. This makes your codebase more organized, easier to understand, and maintainable. It also allows you to selectively include or exclude specific functionality from a class, making the code more focused and purpose-driven.
How to Use Mixins? ⚙️
To use a mixin, you typically create a new class that inherits from both the desired mixin(s) and the target class. The mixin's methods and attributes are then effectively mixed into the target class, extending its functionality. Here's a simple example in Python:
class LoggerMixin:
def log(self, message):
print(f"Logged: {message}")
class User(LoggerMixin):
def __init__(self, username):
self.username = username
user = User("john_doe")
user.log("User created") # Output: Logged: User created
In this example, the LoggerMixin
provides the log
method, which the User
class can use without having to define it explicitly. By inheriting from the LoggerMixin
, the User
class gains access to the mixin's functionality.
Your Turn! 🚀
Now that you understand the concept of mixins and their benefits, it's time to put your knowledge to the test! Think of a scenario where using a mixin could greatly enhance your code. Share your thoughts and ideas in the comments section below and let's continue the conversation! 💬
Remember, mixins are a powerful tool in any developer's arsenal. They promote code reuse, provide flexibility, and help create modular and maintainable code. So don't shy away from embracing mixins in your projects!
If you found this blog post helpful, make sure to share it with your fellow developers and spread the knowledge! Until next time, happy coding! 🙌😊