What does Ruby have that Python doesn"t, and vice versa?
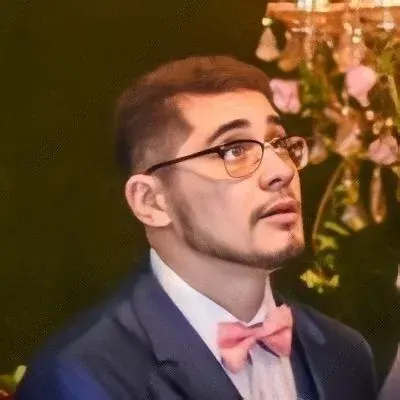
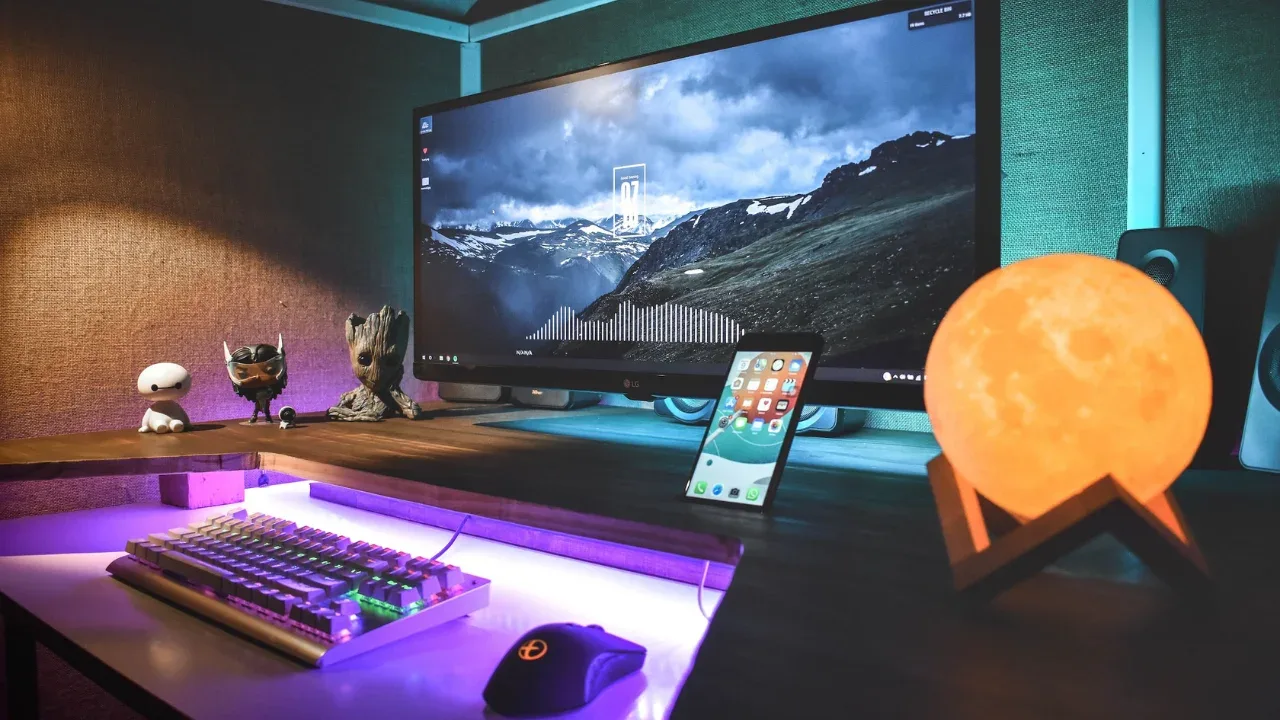
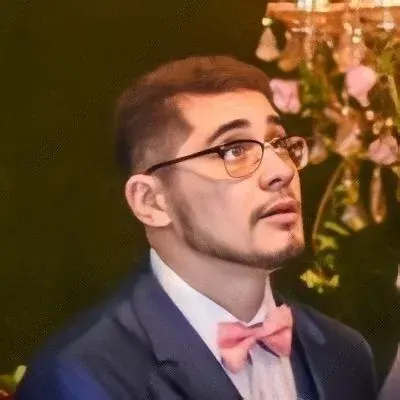
Ruby vs Python: Exploring the Differences and Unique Features
Are you stuck between choosing Ruby and Python for your next project? 🤔 Don't worry, I've got you covered! In this post, I'll be addressing the common issues and specific problems that arise when comparing Ruby and Python. Instead of biased opinions 🙅♂️, I'll provide you with objective differences and examples of what each language can do that the other can't. Let's dive in! 💪
Ruby's Class Reference in the Class Body
In Ruby, you have a reference to the class already in the class body. This allows you to access the class itself from within the class definition body. Here's an example:
class Kaka
puts self
end
In this case, self
refers to the class itself, and the code would print out "Kaka". In Python, you don't have access to the class until after the class construction is finished. So, there's no way to print out the class name or access other class-level definitions from within the class definition body.
All Classes are Mutable in Ruby
In Ruby, all classes are mutable, which means you can develop extensions to core classes. Here's an example of extending the String class in Ruby:
class String
def starts_with?(other)
head = self[0, other.length]
head == other
end
end
On the other hand, in Python, you would have to define a separate function or method to achieve the same functionality. For example:
def starts_with(s, prefix):
return s[:len(prefix)] == prefix
However, you could use it on any sequence, not just strings. To use it, you need to import it explicitly, such as from some_module import starts_with
.
Ruby's Perl-Like Scripting Features
Ruby has first-class regex, $-variables, and other features, which make it more suitable for writing small shell scripts or acting as glue code for other programs. These features are inherited from Perl's scripting capabilities.
Ruby's First-Class Continuations
Thanks to the callcc
statement, Ruby has built-in support for first-class continuations. Continuations in Ruby allow you to capture the current execution state and later resume it from that point. In Python, you can create continuations using various techniques but there's no built-in support in the language itself.
Ruby's Blocks
Ruby has a convenient syntax for creating multi-line anonymous functions called blocks. They are passed as arguments to methods using the "do" statement. Here's an example:
amethod { |here|
many = lines + of + code
goes(here)
}
In Python, you would typically achieve the same result by passing a method or using generators:
with amethod() as here: # `amethod()` is a context manager
many = lines + of + code
goes(here)
or
for here in amethod(): # `amethod()` is an iterable
many = lines + of + code
goes(here)
or
def function(here):
many = lines + of + code
goes(here)
amethod(function) # `function` is a callback
Ruby's "yield" statement, used for calling a block, has a similar effect to Python's generators.
Ruby's Support for Functional-Style Programming
Ruby supports functional-style programming more easily, allowing you to chain methods together in a pipe-like fashion. Here's an example:
myList.map(&:description).reject(&:empty?).join("\n")
In Python, you would achieve the same result using list comprehensions and other functional programming constructs.
Python's Built-in Generators
Python has first-class support for generators, which are similar to Ruby's blocks. Generators in Python allow you to create iterables that can be looped over or used with other iterable constructs. Here's an example of a generator in Python:
def reverse(data):
for index in range(len(data) - 1, -1, -1):
yield data[index]
Ruby, on the other hand, uses the generator module or blocks/procs/lambda for similar functionality.
Python's Flexible Namespace Handling
Python provides flexible namespace handling, allowing you to import only the names you need or import everything from a module. You can also create multi-level namespaces using packages. In Ruby, importing a file with require
pollutes the global namespace unless you use modules to create a namespace.
Python's Docstrings
Python has docstrings, which are strings attached to modules, functions, and methods. These docstrings can be introspected at runtime, making them useful for creating help commands and automatic documentation. Here's an example of a docstring in Python:
def frobnicate(bar):
"""frobnicate takes a bar and frobnicates it
>>> bar = Bar()
>>> bar.is_frobnicated()
False
>>> frobnicate(bar)
>>> bar.is_frobnicated()
True
"""
In Ruby, the equivalent documentation style is similar to Javadocs and is located above the method instead of within it.
Python's Multiple Inheritance
Python supports multiple inheritance, allowing a class to inherit from multiple parent classes. Ruby, on the other hand, does not support multiple inheritance on purpose. However, Ruby reuses the concept of modules as a type of abstract class.
Python's List and Dictionary Comprehensions
Python provides list and dictionary comprehensions, which are concise ways of creating lists and dictionaries. Here's an example:
res = [x * x for x in range(1, 10)]
In Ruby, you can achieve similar results using the map
method:
res = (0..9).map { |x| x * x }
Python also has generator expressions, which are similar to Ruby blocks. They allow you to create generators on the fly without allocating extra memory.
Python's Decorators
Python has decorators, which allow you to modify the behavior of functions or classes. Decorators are useful for adding functionality to existing code without modifying it directly. While similar concepts can be created in Ruby, they are not considered as necessary as in Python.
Syntax Differences
One noticeable syntax difference between Ruby and Python is the use of explicit "end" or "}" to close scopes in Ruby, while Python uses whitespace indentation. However, there have been recent efforts in Ruby to allow whitespace-only indentation as well.
By comparing the unique features and differences between Ruby and Python, I hope I've provided you with a clearer perspective on both languages. Now it's up to you to make the best choice for your project! 🚀 If you still have any lingering questions or need further guidance, feel free to leave a comment below. Happy coding! 👩💻👨💻