What does ** (double star/asterisk) and * (star/asterisk) do for parameters?
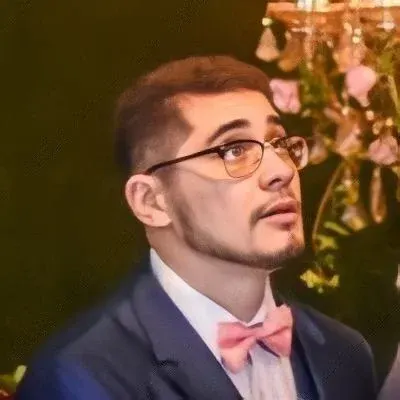

The Magic of * and ** Parameters in Python Functions 🎩✨
Have you ever come across function definitions in Python that include *args and **kwargs parameters? 🧐 Do you wonder what these mysterious symbols do and how they can be helpful? 💭 Well, you've come to the right place! In this blog post, we'll dive deep into the world of * and ** parameters and discover their magic powers. 🌟
Unleashing the Power of the Asterisks 🚀
When you see *args or **kwargs in a function definition, it means that the function can accept a variable number of arguments. Let's break it down step by step and explore each of these powerful symbols. 🔍
The * (Star/Asterisk) Parameter 🌟
The * symbol, also known as the "star" or "asterisk," is used to pass non-keyworded variable-length arguments to a function. In function definitions, it allows you to pass an arbitrary number of arguments in a tuple format. 🌌
In the example below, the function foo(x, y, *args)
accepts two mandatory arguments, x
and y
, and any additional arguments are captured in the args
tuple.
def foo(x, y, *args):
pass
🔎 Common Issue: Suppose you have a function that calculates the sum of any number of integers. Without the * parameter, you would need to explicitly define all the arguments. But with *args, you can pass any number of integers without worrying about explicitly defining them.
def sum_numbers(*args):
total = 0
for num in args:
total += num
return total
sum_numbers(1, 2, 3) # Output: 6
sum_numbers(10, 20, 30, 40) # Output: 100
Amazingly, *args gives you the flexibility to pass any number of arguments without modifying the function's signature. 🎉
The ** (Double Star/Asterisk) Parameter 🌟
The ** symbol, also known as the "double star" or "double asterisk," is used to pass keyworded variable-length arguments to a function. It allows you to pass an arbitrary number of keyword arguments in a dictionary format. 🗝️
In the example below, the function bar(x, y, **kwargs)
accepts two mandatory arguments, x
and y
, and any additional keyword arguments are captured in the kwargs
dictionary.
def bar(x, y, **kwargs):
pass
🔎 Common Issue: Have you ever needed to pass additional parameters to a function without explicitly defining them in advance? That's when **kwargs comes to the rescue! It gives you the ability to pass any number of keyword arguments without modifying the function's signature.
def print_person_info(name, age, **kwargs):
print(f"Name: {name}")
print(f"Age: {age}")
for key, value in kwargs.items():
print(f"{key.capitalize()}: {value}")
print("")
print_person_info("John", 25) # Output: Name: John \n Age: 25
print_person_info("Emma", 30, city="New York", profession="Developer")
# Output: Name: Emma \n Age: 30 \n City: New York \n Profession: Developer
With **kwargs, you can easily handle optional or dynamic arguments within your function. 💪
One Last Remark 📣
The power of * and ** parameters doesn't stop here! When calling a function, you can also use * and ** to unpack iterable objects like lists or dictionaries into separate arguments. This can be incredibly useful when passing arguments stored in a container. 📦
nums = [1, 2, 3]
sum_numbers(*nums) # Equivalent to sum_numbers(1, 2, 3)
person_info = {"name": "Alice", "age": 28, "city": "London"}
print_person_info(**person_info)
# Equivalent to print_person_info(name="Alice", age=28, city="London")
Remember, the magic of * and ** parameters allows you to handle flexibility and dynamic argument passing effortlessly. ✨💪
Your Turn! 💡
Now that you understand the power of *args and **kwargs, why not give it a try? Refactor one of your existing functions or create a new one that benefits from the flexibility these parameters provide. Share your experience and code snippets in the comments below! Let's unleash the full potential of Python together! 🚀💻
<hr />
🔍 Check out Stack Overflow for more information on arguments and function calls in Python.
Now it's your turn to become a master of Python function parameters! 💪✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
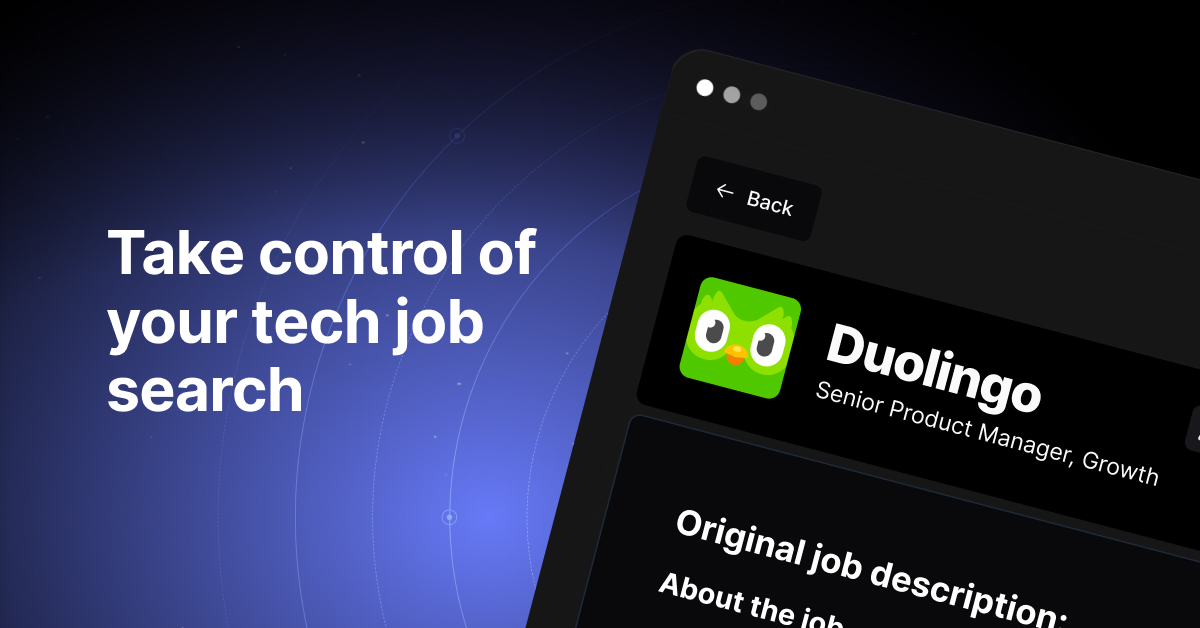