What does axis in pandas mean?
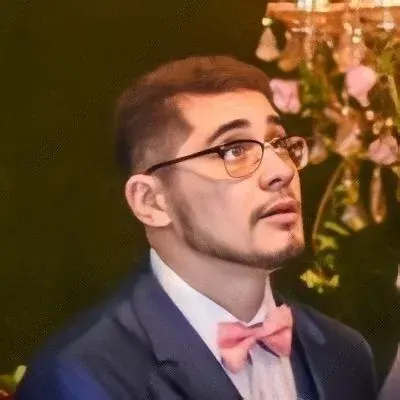
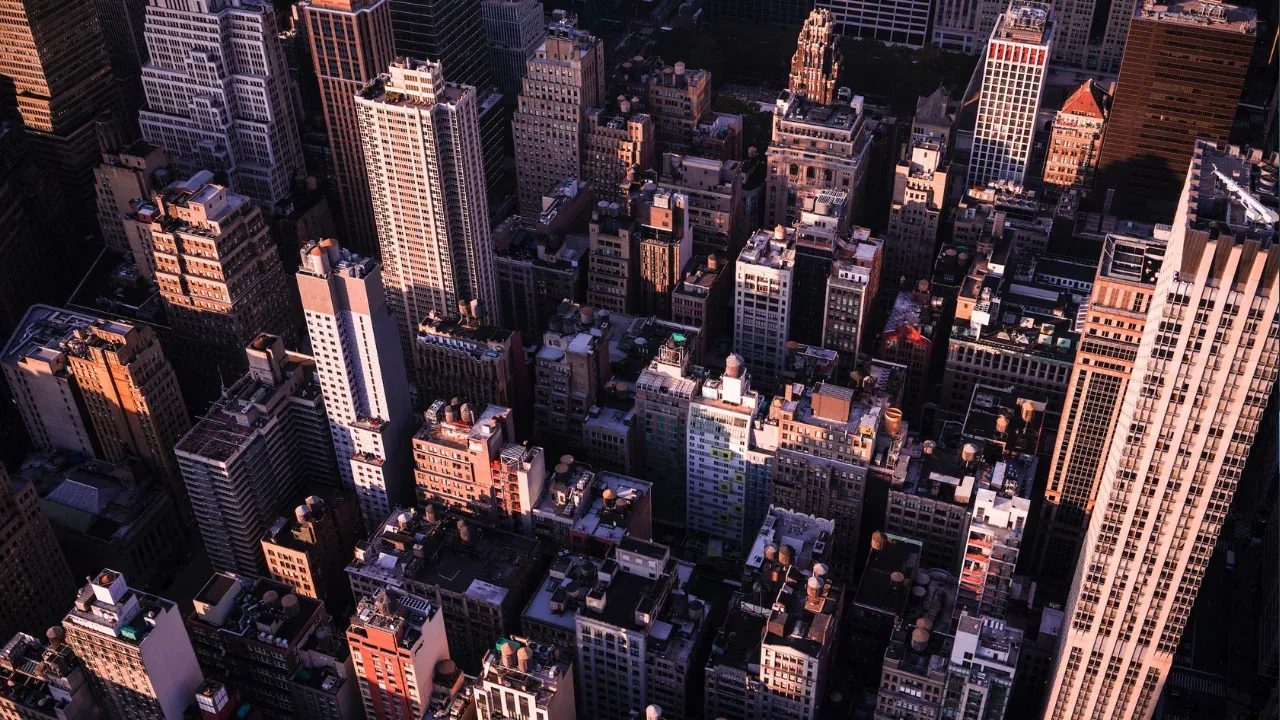
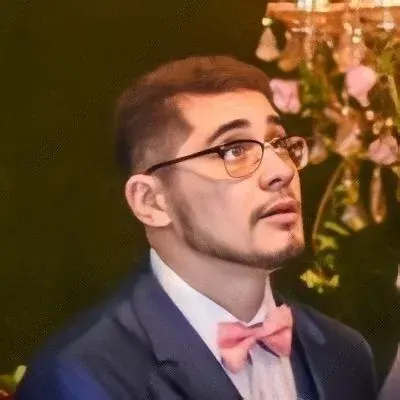
Understanding Axis in Pandas
Have you ever come across the term "axis" in the context of pandas and wondered what it actually means? π€ This blog post will demystify the concept of axis in pandas and provide easy solutions to common problems you might encounter. So, let's dive in and discover what axis is all about! πͺ
What is Axis?
In simple terms, an axis refers to either the rows or the columns of your DataFrame. Think of it as the direction along which pandas performs operations. By specifying the axis, you can control whether an operation is applied to the rows or the columns of your DataFrame.
Default Axis Values
Before we delve deeper into the concept of axis, it's important to understand its default values. In pandas, the default axis values are as follows:
0: Specifies the rows or indexes
1: Specifies the columns
Analysing the Problem
To better understand the concept of axis, let's take a look at an example. Suppose we have the following DataFrame:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.random.randn(1,2),columns=list('AB'))
Which gives us the following DataFrame:
A B
0 0.626386 1.523255
Now, if we run the following command:
df.mean(axis=1)
The resulting output is:
0 1.074821
dtype: float64
Explaining the Output
In our example, we applied the mean()
function to our DataFrame along the 1st axis, which corresponds to the columns. This means that pandas calculated the mean value for each column independently, resulting in a Series with the column names as indexes and the mean values as their respective values. So, the expected output:
A 0.626386
B 1.523255
dtype: float64
can be obtained by applying the mean()
function along the 0th axis (rows) instead.
Handling Axis-related Issues
Now that we understand the concept of axis, let's address some common issues you might encounter when working with pandas:
Issue 1: Unexpected Results
If you're getting unexpected results from a pandas operation, a good place to start is by checking whether you've specified the correct axis. Sometimes, using the incorrect axis can lead to incorrect or unexpected outputs.
Solution:
Double-check the documentation to understand the correct usage of axis for the specific operation you're performing.
Experiment with different axis values (0 or 1) to obtain the desired output.
Issue 2: Missing Data
When performing operations along an axis, pandas will typically ignore any missing or NaN values.
Solution:
Ensure that your DataFrame is clean and doesn't contain any missing values. You can use functions like
df.dropna()
ordf.fillna()
to handle missing data before applying operations along an axis.
Your Turn to Engage!
Now that you have a solid understanding of axis in pandas, why not put your newfound knowledge into practice? Try running some pandas operations, specifying different axis values, and see how the results change. Feel free to share your experiences, ask questions, or provide feedback in the comments section below. Let's keep this learning journey going! π
Remember, pandas offers a multitude of powerful operations that can be performed along different axes. Understanding the concept of axis and how to use it effectively will undoubtedly enhance your pandas skills.
So, what are you waiting for? Take your pandas knowledge to the next level and start exploring the possibilities that axis brings! πΌπ‘
Happy coding! π»β¨