What does a bare asterisk do in a Python parameter list? (What are "keyword-only" parameters?)
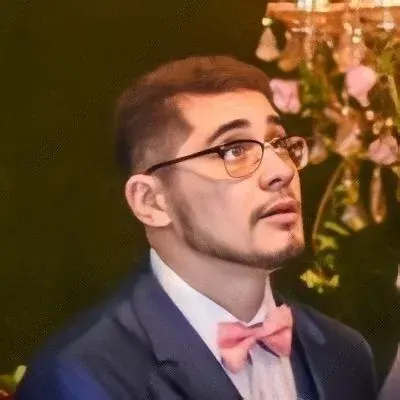
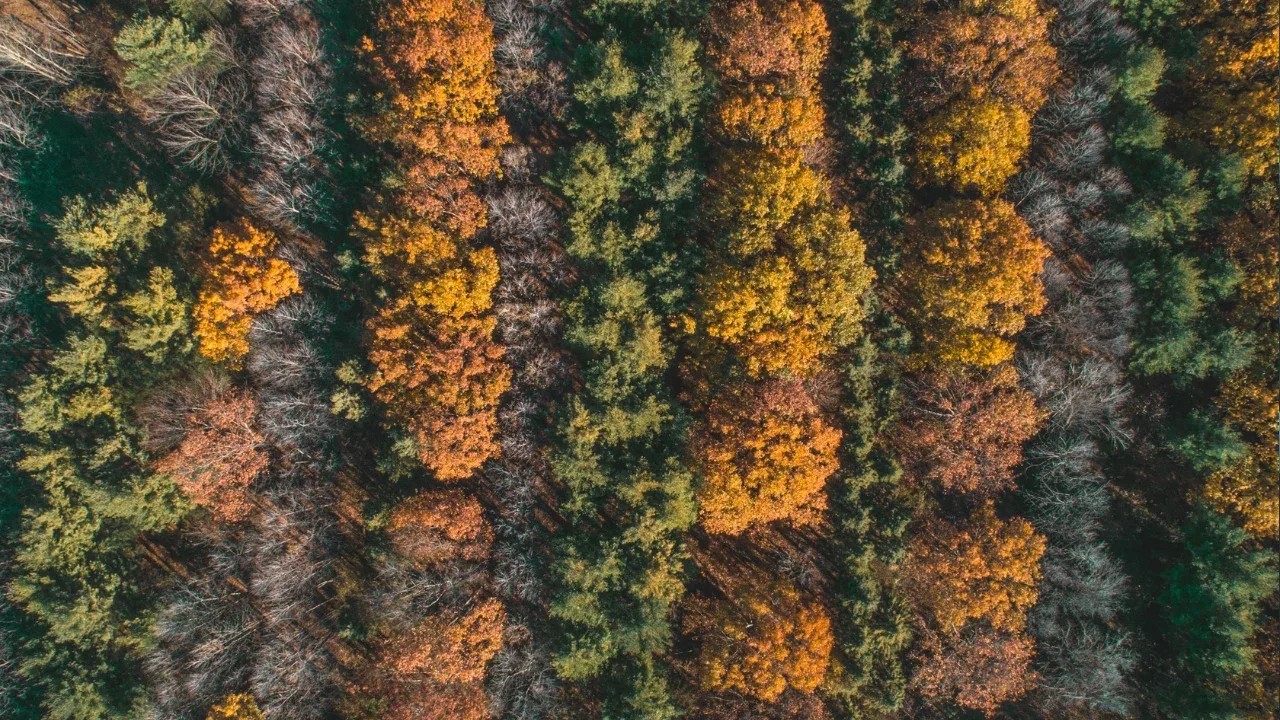
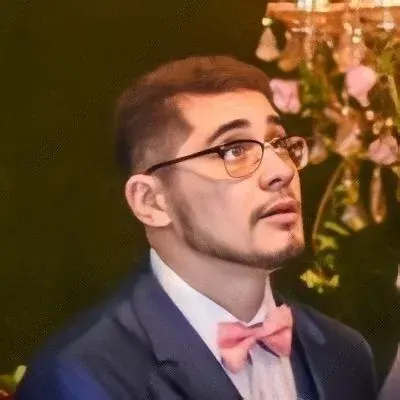
What does a bare asterisk do in a Python parameter list? 🤔
You may have come across a Python function with a bare asterisk * in its parameter list and wondered what it does. Let's break it down and understand what this mysterious symbol means.
Introduction to *args and **kwargs
Before we dive into the bare asterisk, let's quickly review *args and **kwargs. These are common notations used in Python to handle an arbitrary number of arguments in a function.
*args allows you to pass a variable number of non-keyword arguments to a function.
**kwargs allows you to pass a variable number of keyword arguments to a function.
Now, let's explore how the bare asterisk, also known as keyword-only parameters, fits into this picture.
Understanding keyword-only parameters
When defining a function, we can specify that some parameters must be passed as keyword arguments. This means that these parameters can only be passed using their names and not as positional arguments.
To indicate that a parameter is a keyword-only parameter, we use the bare asterisk * in the function's parameter list. This tells Python that all following parameters must be passed as keyword arguments.
Here's an example to illustrate this concept:
def greet(name, *, age):
print(f"Hello, {name}! I see you are {age} years old.")
greet("Alice", age=25)
In the above example, the parameter name
can be passed as a positional argument, but the parameter age
must be passed as a keyword argument. If we try to call the greet
function without specifying age
, we will get an error.
Solving the SyntaxError: named arguments must follow bare * error 💡
The SyntaxError: named arguments must follow bare \*
is raised when we use the bare asterisk * without any parameter names following it. This error serves as a reminder that keyword-only parameters should follow the bare asterisk.
To fix this error, we can either remove the bare asterisk if we don't intend to have any keyword-only parameters, or add one or more parameter names after the asterisk.
Here's a corrected version of the example that caused the error:
def func(*args):
pass
In the corrected example, we removed the bare asterisk, resulting in a function that accepts a variable number of non-keyword arguments using the *args notation.
Conclusion and call-to-action 🎉
We've learned about the bare asterisk in Python parameter lists and how it signifies keyword-only parameters. It's important to remember that keyword-only parameters must be passed using their names as keyword arguments.
Next time you encounter the bare asterisk, remember its role in specifying keyword-only parameters.
Did you find this information useful? Let us know your thoughts in the comments below! And don't hesitate to share this blog post with your Python-loving friends. Happy coding! 🚀