What are type hints in Python 3.5?
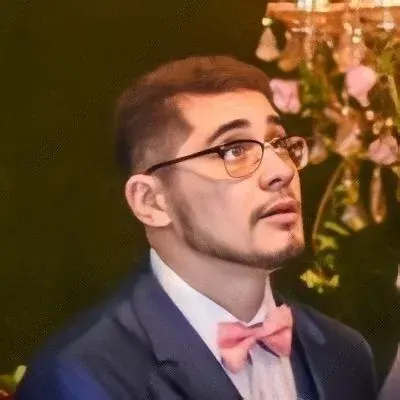
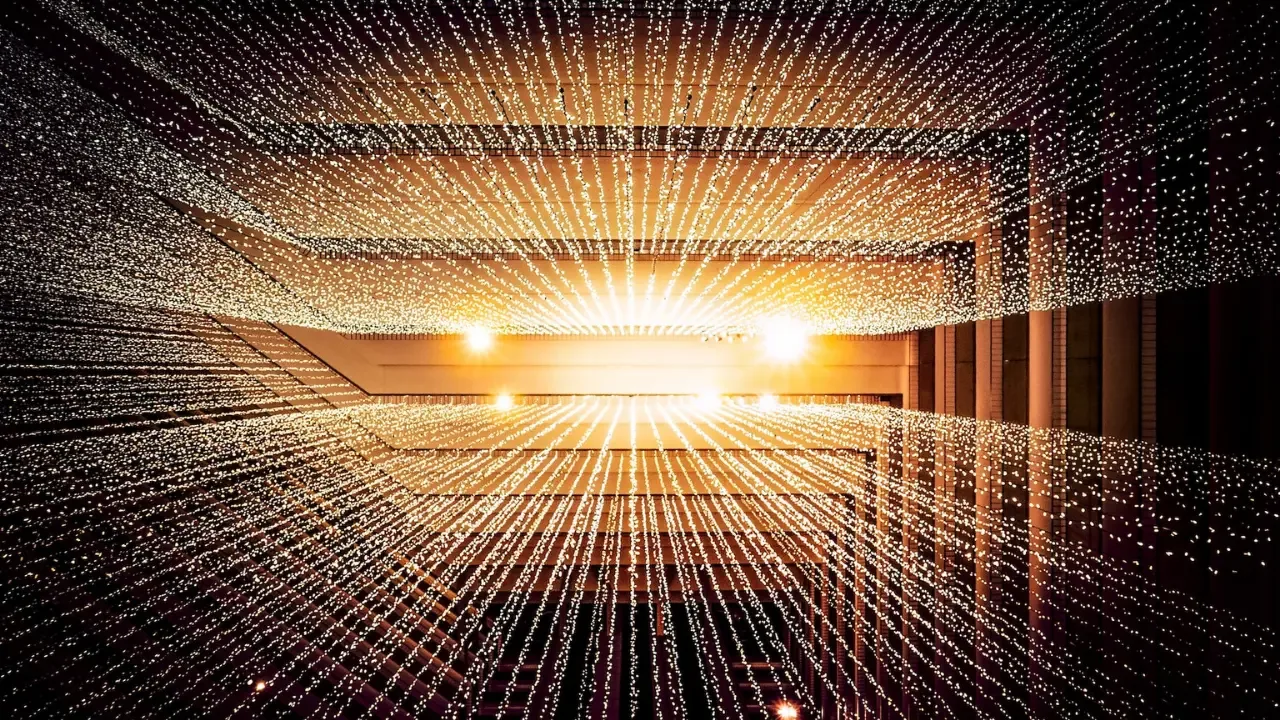
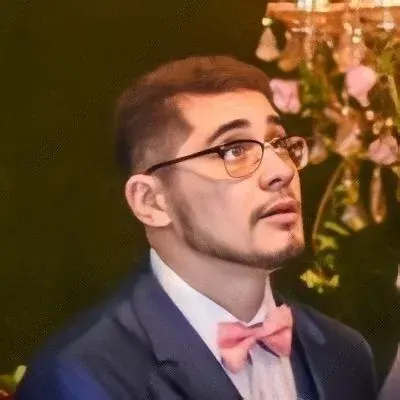
The Power of Type Hints in Python 3.5 ππͺ
One of the most buzz-worthy features in Python 3.5 is the introduction of type hints. If you've ever found yourself scratching your head while trying to understand the expected types of arguments and return values in a function, rejoice! Type hints are here to save the day! π¦ΈββοΈπ₯
What are Type Hints? π€
Type hints are a way to annotate the expected types of arguments and return values in Python functions. By adding these hints, you provide additional clarity to both developers and tools about how functions should be used and what kind of input they expect.
Imagine you're working on a project with a teammate, and they pass a string into a function that you've designed to only accept integers. π§βπ€βπ§ With type hints, you can catch these type mismatches early on and fix them before they cause any issues.
How to Use Type Hints π
Using type hints is as simple as adding a colon followed by the expected type after the argument or return value in your function definition. Let's see that in action:
def add_numbers(a: int, b: int) -> int:
return a + b
In this example, we've indicated that the add_numbers
function expects two arguments (a
and b
) of type int
and will return an int
as well.
Python itself does not enforce these type hints, as it remains a dynamically typed language. However, with the help of external tools like mypy or PyCharm, we can perform static type checking and catch potential type errors before they happen during runtime. β‘οΈπ«
When to Use Type Hints and When Not To π€·ββοΈ
Type hints can be incredibly useful in scenarios where your codebase is large or complex, or if you're collaborating with other developers. They can help improve code quality, make it easier to understand and maintain, and reduce the chances of bugs caused by type-related issues. ππ§
However, it's important to note that type hints are purely optional. If you're working on a smaller project or prototyping, you may choose to skip them. It's ultimately up to you and your team to decide the ideal balance between using type hints and the additional time required to implement them. ππ€
Your Turn to Use Type Hints π¨βπ»π©βπ»
Now that you're familiar with type hints, it's time to put them into practice! Take a look at your existing Python codebase and identify any functions or methods where type hints can add value. Start by adding hints to a few critical areas and see how it improves the readability and maintainability of your code. ππ‘
Remember, the power of type hints lies in using them responsibly. Don't get carried away with obsessively annotating every line of code; focus on using them where they provide the most benefit. βοΈπΌ
So, what are you waiting for? Start sprinkling your code with those handy type hints and watch your Python projects soar to new heights! ππ»
Have you already tried using type hints in Python 3.5? How did it impact your development experience? Share your thoughts in the comments below! Let's start a conversation and learn from each other! π£οΈπ¬