What are the differences between type() and isinstance()?
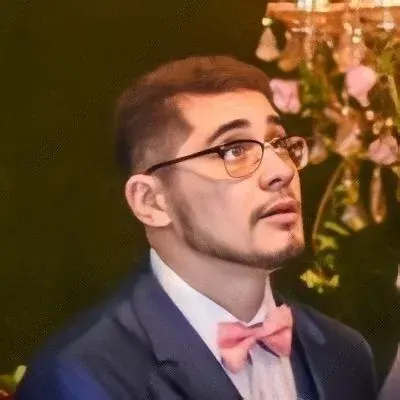
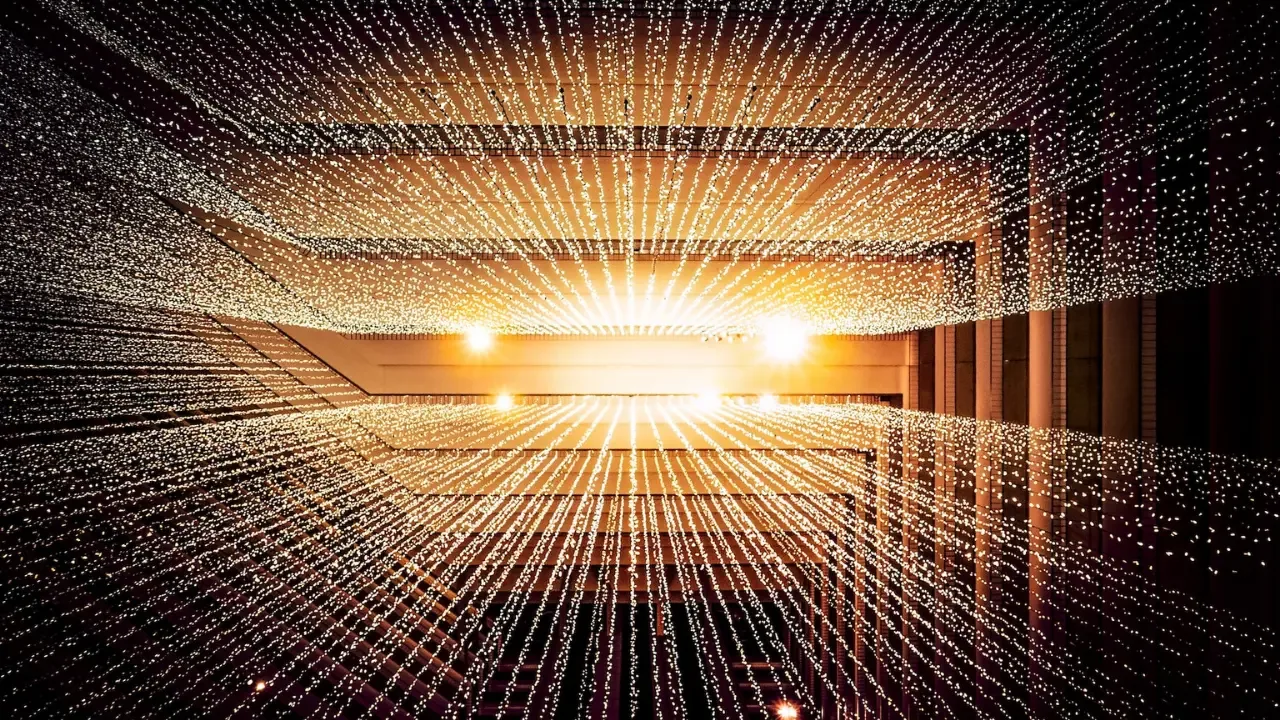
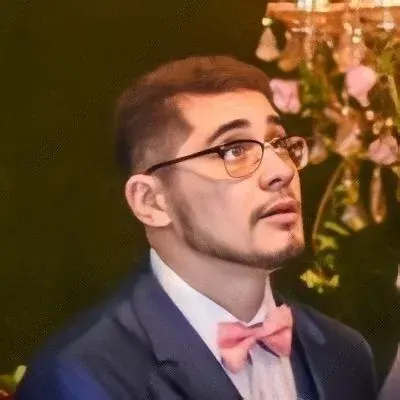
š Title: The Battle of Type() vs isinstance(): What's the Difference?
š Hey there, fellow coders! Today, we're diving into a common confusion that many Pythonistas face - the differences between type()
and isinstance()
. These two code snippets may seem similar at first sight, but they're more different than pineapple on pizza! šš
šÆ Understanding the Problem
You see, both functions serve the purpose of checking the type of an object. However, their implementation and usage differ significantly. So, let's break it down!
š type(): The Bookworm
The type()
function is as simple as it gets. It returns the exact type of an object. In the first code snippet, we imported the types
module and used type()
alongside it. This approach is somewhat old-school and not commonly seen in modern Python code. Nonetheless, we won't dismiss it just yet!
import types
if type(a) is types.DictType:
do_something()
if type(b) in types.StringTypes:
do_something_else()
In this example, types.DictType
and types.StringTypes
are specific types defined within the types
module. It's essential to note that these types are not built-in Python types but legacy artifacts from older versions. š¦
š isinstance(): The Serpent
On the other hand, isinstance()
is Python's go-to function when checking types. It not only checks for the exact type but also allows for inheritance and subclassing checks. Let's hop into the second code snippet to see how it shines:
if isinstance(a, dict):
do_something()
if isinstance(b, str) or isinstance(b, unicode):
do_something_else()
Here, we use isinstance()
directly, passing in the object and the desired type(s) as arguments. This function provides flexibility by accepting either a single type or a tuple of types. It's like having a Swiss Army knife for type checking! šŖ
š¤ The Million-Dollar Question: Which One to Use?
Now that we know the difference, it comes down to choosing the right tool for the job. Here's a handy rule of thumb:
If you need a precise type match, use
type()
.If you want to handle subclasses or check for multiple types, go for
isinstance()
.
It's worth noting that using isinstance()
is generally preferred in most scenarios due to its flexibility and robustness.
š” Easy Solutions
Solution 1: Embrace Simplicity
For a more modern approach, ditch the types
module and the outdated types like types.DictType
or types.StringTypes
. Instead, embrace simplicity and use the built-in Python types directly:
if type(a) is dict:
do_something()
if type(b) is str:
do_something_else()
By doing this, you leverage the power of type()
without adding unnecessary complexities.
Solution 2: Unleash the Serpent
However, if you require more flexibility, stick with isinstance()
. It is often the way to go, especially when dealing with inheritance or multiple types:
if isinstance(a, dict):
do_something()
if isinstance(b, (str, unicode)):
do_something_else()
Here, we pass a tuple of types to check if b
is either a string or a unicode object.
š£ Calling All Pythonistas!
Phew! We've wrangled the perplexities of type()
and isinstance()
together. šŖ
Now, it's time for you to put this knowledge into action! Experiment, have fun, and let us know in the comments which approach you prefer and why. Have you encountered any scenarios where one worked better than the other?
Remember, understanding these differences empowers you to write cleaner and more efficient code! Happy typing, and may your code be blessed with clarity! šāØ