What are the differences between json and simplejson Python modules?
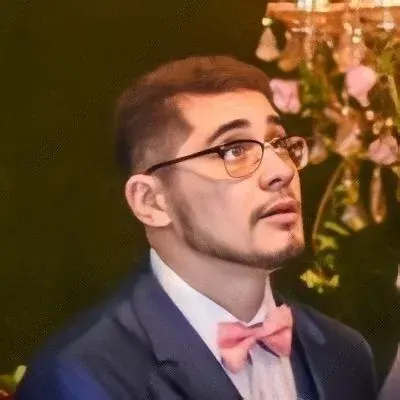
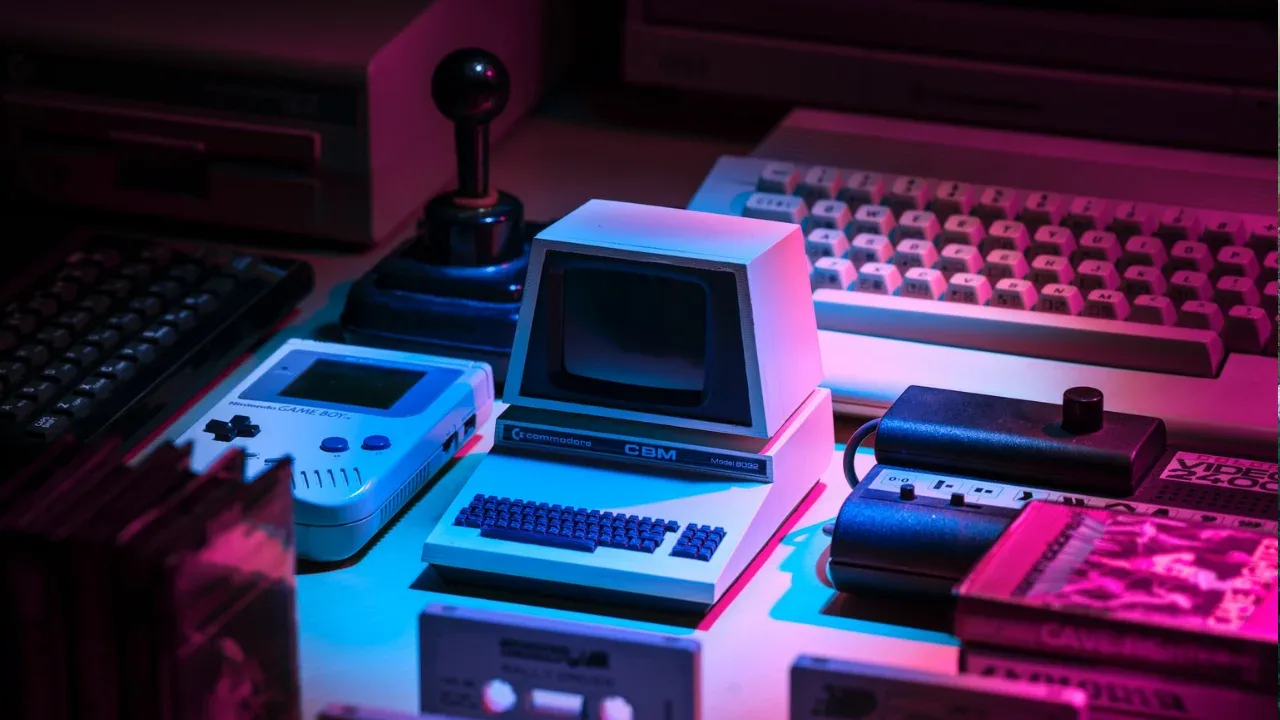
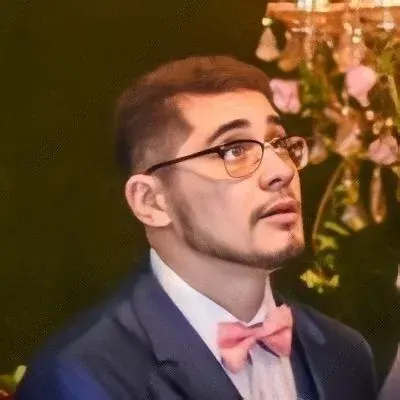
JSON vs SimpleJSON: Exploring the Differences
So you want to learn about the differences between the json
and simplejson
Python modules? You've come to the right place! 🚀
What is JSON?
JSON (JavaScript Object Notation) is a popular data interchange format widely used in web development. It provides a lightweight and human-readable way to represent data structures consisting of key-value pairs, lists, and other data types.
The json Module in Python's Standard Library 📚
Python comes with a built-in json
module as part of its Standard Library. This module provides everything you need to encode and decode JSON data in Python.
Here's some sample code to give you a taste of how it works:
import json
# JSON to Python
json_data = '{"name": "John", "age": 30, "city": "New York"}'
python_data = json.loads(json_data)
# Python to JSON
python_data["city"] = "San Francisco"
json_data = json.dumps(python_data)
The json
module is reliable, widely supported, and offers excellent performance. It's recommended to use the json
module for most scenarios, and you won't need to install any additional dependencies.
The SimpleJSON Alternative 🌟
However, there exist alternative JSON libraries, such as simplejson
, which provides extended features and better performance in certain cases.
simplejson
is designed to be compatible with the json
module in the Python Standard Library, making it a drop-in replacement. To use it, you need to install it as a separate package. To install simplejson
, you can use pip
:
pip install simplejson
Why Use SimpleJSON?
There are several reasons why you might choose to use simplejson
over the built-in json
module:
1. Better Performance ⚡
In some cases, simplejson
can provide significant performance improvements over the default json
module when encoding or decoding large JSON datasets. If your application deals with substantial amounts of JSON data, using simplejson
might be a good option.
2. Additional Features 🌈
simplejson
extends the functionality provided by the json
module, offering additional features not found in the standard library. For example, it supports serializing custom objects by defining the __json__
method, which gives you finer control over the serialization process.
3. Backward Compatibility 🔄
For Python 2 users, simplejson
offers backward compatibility with older versions of Python, allowing you to use the latest JSON features even when working with an older Python version.
A Word of Caution ⚠️
While simplejson
offers compelling benefits, it's important to note that it's a third-party library and might not receive the same level of security audits, bug fixes, and updates as the built-in json
module. For critical applications where security and stability are of utmost importance, sticking to the json
module from the Python Standard Library is generally recommended.
Conclusion and Your Turn 🎉
In most cases, the built-in json
module is sufficient for working with JSON data in Python. It's dependable, compatible, and requires no additional installations.
However, if you find yourself in need of superior performance or extra features, simplejson
presents a powerful alternative.
Now that you understand the differences, it's time to apply your newfound knowledge! Let us know in the comments below which JSON module you prefer and why. Happy coding! 💻✨