What are metaclasses in Python?
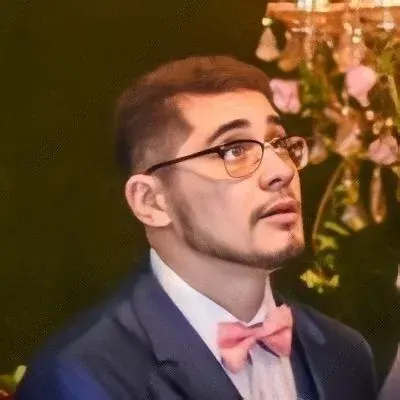
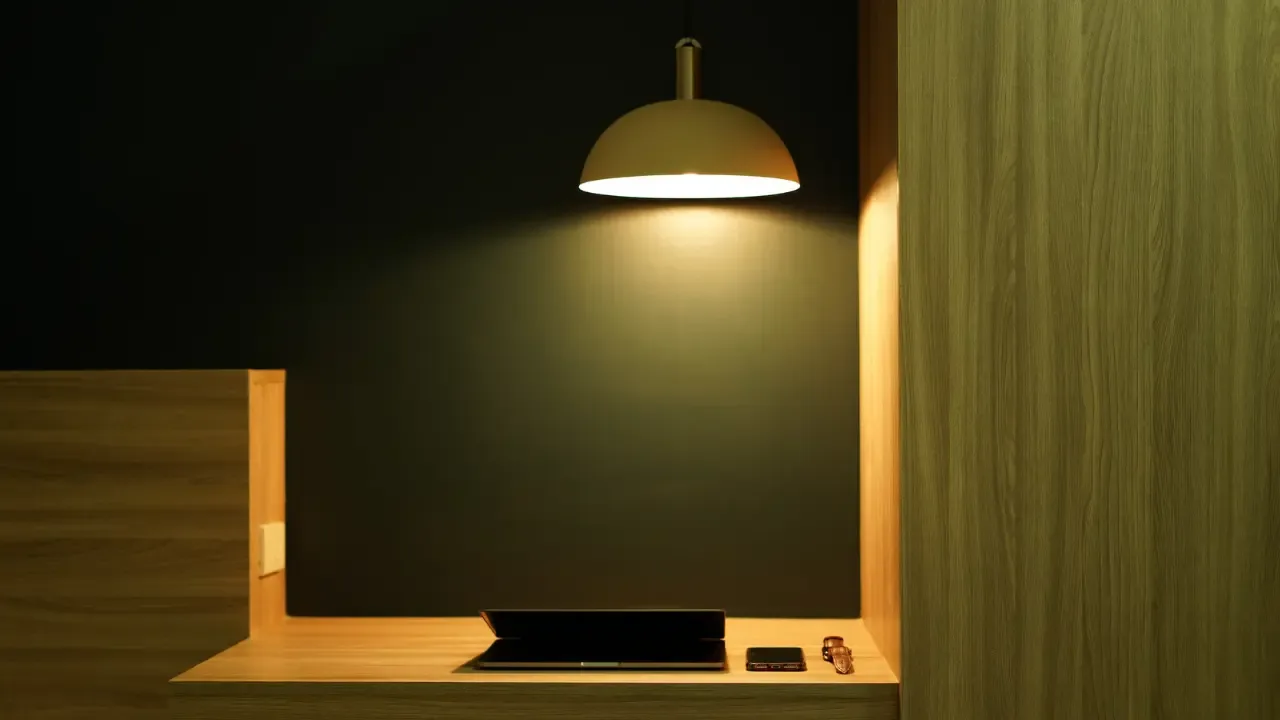
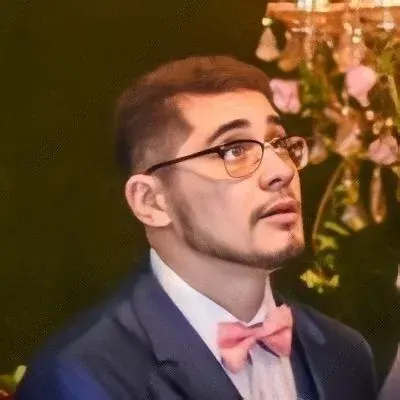
🔍 What are Metaclasses in Python? 🤔
Have you ever wondered what those mysterious metaclasses in Python are all about? 🤔 Are they just some fancy buzzword or do they actually have a practical use? Let's dive into the world of metaclasses and uncover their secrets! 🕵️♂️
📚 Understanding Metaclasses
In Python, a metaclass is a class that defines the behavior of other classes. It's like a blueprint for creating classes. Just as classes define the attributes and methods for creating objects, metaclasses define the attributes and methods for creating classes. 🏗️
Metaclasses use a special method called __new__()
to customize the creation of new classes. This gives you the power to intervene in the class creation process and modify or enhance it according to your needs. Metaclasses are often used when you want to apply some common functionality or behavior to multiple classes. 🚀
💡 Why Use Metaclasses?
Metaclasses can be very useful in certain scenarios, such as:
1. Imposing Constraints:
Metaclasses allow you to enforce certain rules or constraints on classes. For example, you can use metaclasses to ensure that all subclasses of a particular class in your codebase implement certain methods or adhere to specific coding conventions. This can help in maintaining consistency and preventing errors. 🛠️
2. Code Generation:
Metaclasses also enable you to generate code dynamically. You can use them to automatically add new attributes, methods, or even whole new classes to your code based on certain conditions. This can be handy in scenarios where repetitive code generation is required. 🤖
3. ORMs and Frameworks:
Metaclasses are extensively used in Object-Relational Mapping (ORM) libraries and frameworks, such as Django. They provide a way to define custom behavior for database models or API endpoints by leveraging metaclasses. This allows for flexible and powerful abstractions. 💪
⚡️ Simple Example
To get a better understanding of metaclasses, let's look at a simple example where we create a metaclass called MetaLogger
that adds logging functionality to classes.
class MetaLogger(type):
def __new__(cls, name, bases, attrs):
# Add logging functionality to all methods
for attr_name, attr_value in attrs.items():
if callable(attr_value):
attrs[attr_name] = cls._add_logging(attr_value)
return super().__new__(cls, name, bases, attrs)
@staticmethod
def _add_logging(func):
def wrapper(*args, **kwargs):
print(f'Logging: {func.__name__}()')
return func(*args, **kwargs)
return wrapper
class MyClass(metaclass=MetaLogger):
def method1(self):
print('Hello, World!')
obj = MyClass()
obj.method1() # Output: Logging: method1() \n Hello, World!
In this example, the MetaLogger
metaclass intercepts the creation of the MyClass
class and adds logging functionality to all of its methods. When we create an instance of MyClass
and call its method1()
, the metaclass's wrapper function is executed first, printing a log message, followed by the actual method execution.
🏁 Call-To-Action
Metaclasses can be a powerful tool in your Python arsenal. By understanding and utilizing them effectively, you can add advanced functionality to your codebase, enforce conventions, or generate code dynamically.
So, next time you encounter a scenario that calls for custom class creation or manipulation, consider giving metaclasses a try! 🚀
Share your thoughts and experiences with metaclasses in the comments below. Have you used them in your projects? What challenges did you face? Let's learn from each other! 🤝