What are good uses for Python3"s "Function Annotations"?
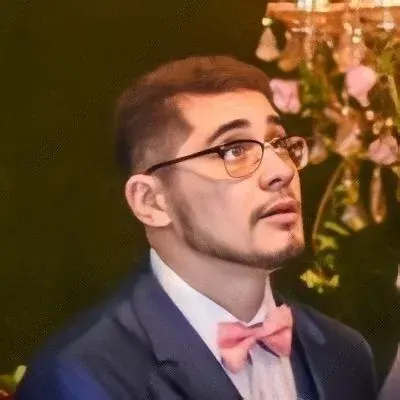
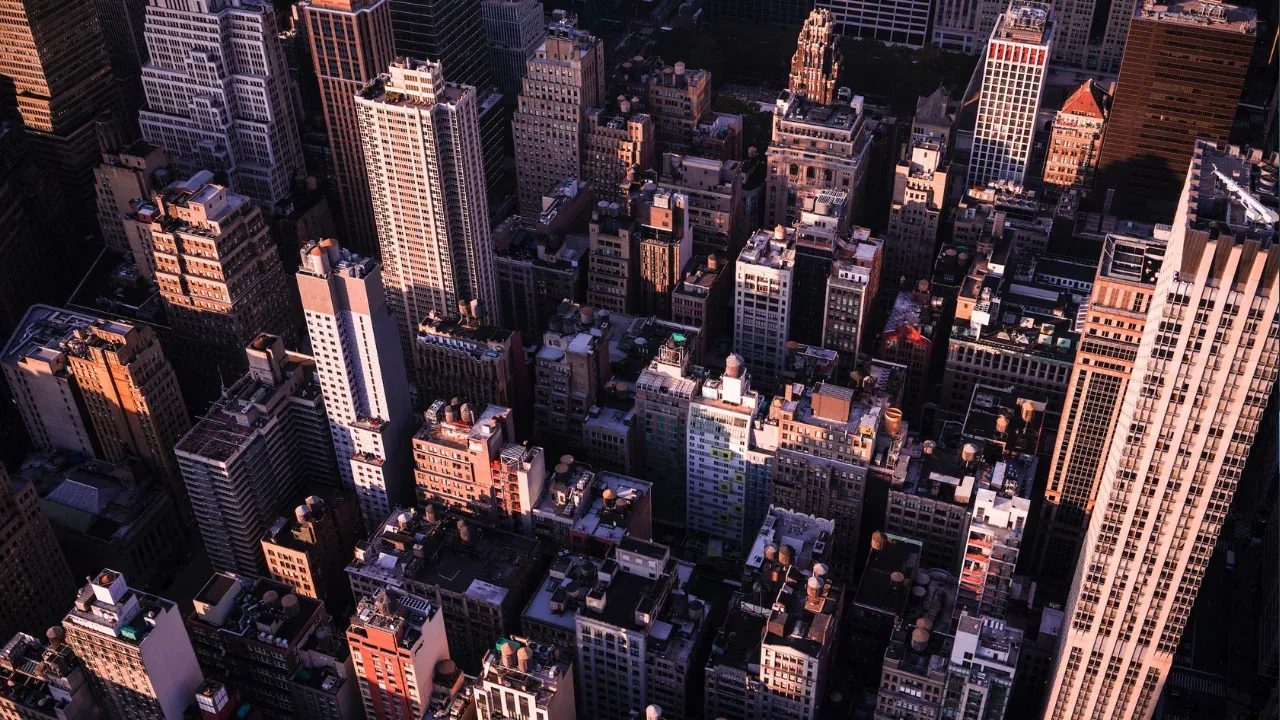
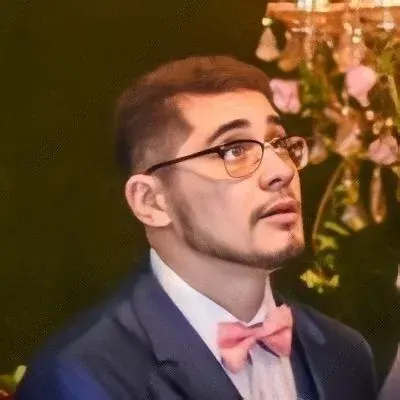
🐍 Python3's Function Annotations: Unleashing the Power of Annotations! 💪
Are you curious about those funky function annotations you've been seeing in Python3 code? Wondering what they're all about and how you can use them to level up your Python skills? Well, you're in the right place! In this guide, we'll dive deep into the world of Python3's function annotations and explore their useful applications. Get ready to transform your code into a masterpiece! 🎨👨💻
Note: If you're new to function annotations, check out PEP-3107. It's the original document that introduced function annotations to Python3.
What are Function Annotations?
In a nutshell, function annotations allow you to attach arbitrary metadata to the arguments and return value of a function. Annotations are expressed as expressions and can be any valid Python expression. This means you can use literals, variables, or even complex expressions as annotations. 📝
Let's take a look at an example to see annotations in action:
def foo(a: 'x', b: 5 + 6, c: list) -> max(2, 9):
... function body ...
In the example above, a
, b
, and c
are the function arguments, while max(2, 9)
is the annotation for the return value. The values after the colons (:
) represent the annotations.
The Power of Function Annotations: Why Should You Care?
Now that we understand what function annotations are, you might be wondering why they're so powerful and why they exist in Python3. Well, prepare to have your mind blown! 🤯
1. Improved Code Readability and Documentation 📚
Function annotations serve as self-documentation for your code. They provide valuable information about the types and purposes of function arguments, making your code easier to understand and maintain.
By including annotations, you create a clear contract between the function and its callers. This reduces confusion and helps prevent potential bugs caused by incorrect usage of the function.
2. Enables Static Type Checking ✅
Function annotations play a vital role in enabling static type checking in Python. Static type checkers like Mypy can analyze your code and identify potential type-related errors before you even run it. This leads to more robust and reliable code.
With function annotations, you can specify the expected types of your function arguments and return value. Static type checkers can then verify if your code adheres to these type annotations and provide early feedback.
3. Tooling and IDE Integration 🛠️
Function annotations open up a world of possibilities when it comes to tooling and IDE integration. IDEs like PyCharm can leverage annotations to provide intelligent code completion, better code navigation, and enhanced refactoring capabilities.
Moreover, annotations enable third-party libraries and frameworks to build upon this metadata, providing additional functionality and insights tailored to the specific needs of your codebase.
Awesome Uses for Function Annotations 🌟
Alright, now that you know why function annotations are a powerful tool, let's explore some exciting use cases where they can shine! Here are a few examples to get your creative juices flowing:
1. Type Hints and Improved Debugging
Annotations can be used to hint at the expected types of function arguments and return values. This can help both humans and tools understand your code better and catch potential type-related errors early on.
def calculate_area(length: float, width: float) -> float:
return length * width
In the example above, the annotations indicate that length
and width
are expected to be float
values, and the return value will also be a float
. This clarifies the purpose of the function and allows IDEs or linters to provide helpful hints and catch potential type mismatches.
2. Documentation Extravaganza 🎉
Annotations make it super easy to generate comprehensive documentation for your code. Tools like Sphinx can automatically extract annotations and generate beautiful documentation that not only documents the function's purpose but also its expected arguments and return value.
So, unleash your inner poet 📜 and annotate your functions to impress your teammates with self-explanatory and visually stunning documentation!
3. Runtime Validation and Contract Verification
Annotations can also be leveraged to perform runtime validation and contract verification. By adding custom annotations and using decorators or custom decorators, you can enforce a strict contract between your code and its callers.
def validate_arguments(func):
def wrapper(x: int, y: int) -> int:
if x < y:
raise ValueError("x must be greater than y")
return func(x, y)
return wrapper
@validate_arguments
def add(x: int, y: int) -> int:
return x + y
In this example, the validate_arguments
decorator ensures that x
is always greater than y
before calling the add
function. This adds an extra layer of safety to your code by validating the input arguments based on their annotations.
Ready to Level Up Your Python Game? 🚀
Congratulations! You've unlocked the hidden potential of Python3's function annotations. Now it's time to unleash their power in your own code.
Start by adding annotations to your functions, provide type hints, and make your code more readable and maintainable. Experiment with different use cases and explore how annotations can enhance your development experience. Don't forget to leverage the vast ecosystem of tools and frameworks that can take your annotations to the next level. 🙌
So go forth, annotate your functions with joy, and let your code sparkle ✨. Show the world the elegance and power of Python3's function annotations!
We'd love to hear your experiences and creative ways of using function annotations. Share your thoughts, examples, and questions in the comments below. Let's join forces and embrace the annotation revolution together! 💪💬
Happy coding! 🐍💻