Validating with an XML schema in Python
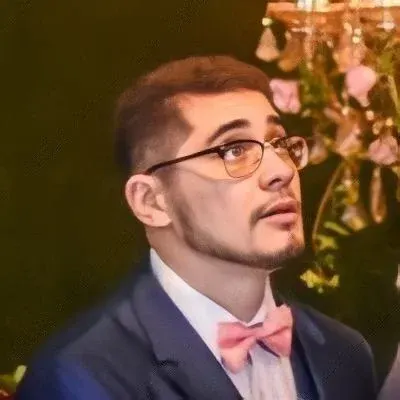
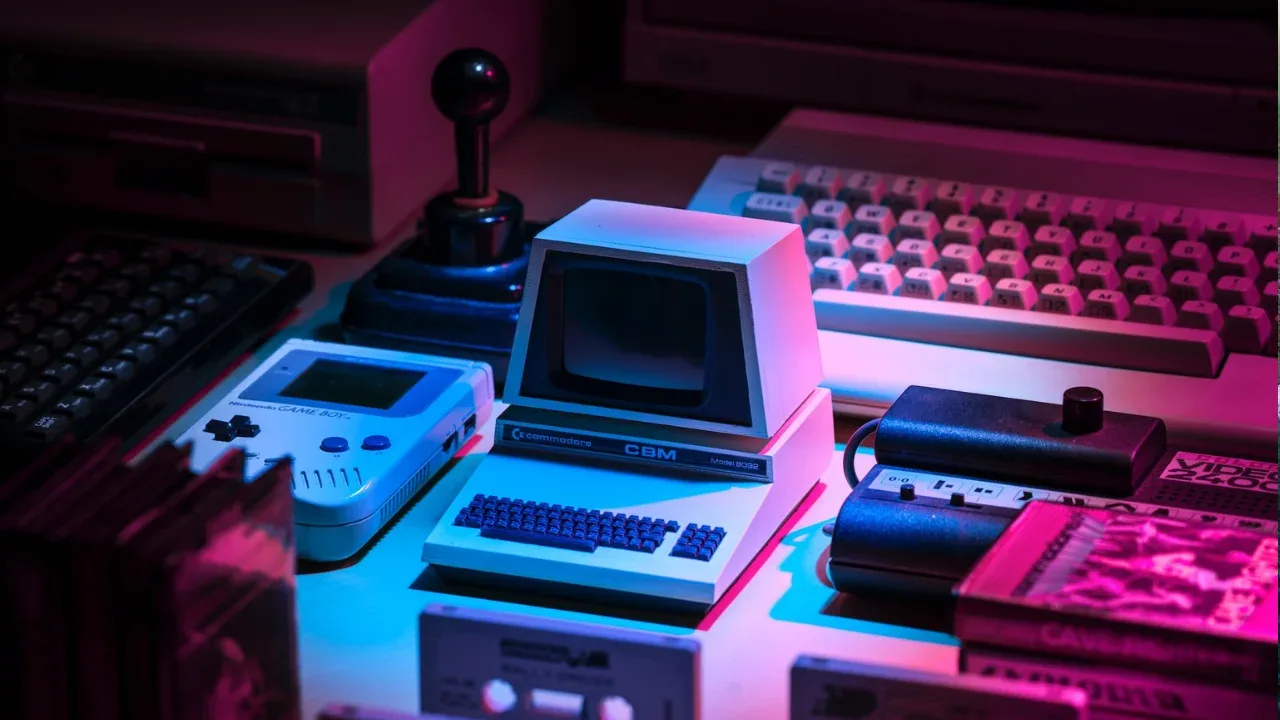
Validating with an XML schema in Python: A Complete Guide 🐍🔍
Are you facing the challenge of validating an XML file against a schema in Python? Look no further! In this article, we will explore common issues and provide easy solutions for validating XML files using Python's standard library. 📋💡
Understanding the Challenge ⚙️
The goal is to ensure that an XML file conforms to a given schema. This involves checking the structure, data types, and constraints specified in the schema. Validation helps guarantee that the XML file is properly formatted and meets the expected standards. 📄✅
Many developers prefer using the standard library for XML validation. However, if required, we will also discuss utilizing third-party packages. Let's dive into the solutions now! 💻🚀
Solution 1: Python's Standard Library 📚
Python's built-in library, xml.etree.ElementTree
, provides a simple and efficient way to parse and validate XML files against an associated schema. Here's a step-by-step process to get you started:
Load the XML file and the schema:
import xml.etree.ElementTree as ET xml_file_path = "path/to/your/xml_file.xml" schema_file_path = "path/to/your/schema.xsd" xml_tree = ET.parse(xml_file_path) schema_tree = ET.parse(schema_file_path) xml_root = xml_tree.getroot()
Create a
xmlschema
object from the schema:from xml.etree.ElementTree import XMLSchema schema = XMLSchema(schema_tree)
Validate the XML file against the schema:
is_valid = schema.validate(xml_tree)
The
is_valid
variable will beTrue
if the XML file adheres to the schema; otherwise, it will beFalse
.
And that's it! You've successfully validated your XML file using only Python's standard library. However, if you're open to third-party packages, let's explore an alternative solution. 🌟🛠️
Solution 2: Third-Party Packages 📦
If you prefer a more feature-rich XML validation experience, you can rely on third-party libraries like lxml
or xmlschema
. These packages offer additional functionalities and advanced validation options.
To install lxml
, use the following command:
pip install lxml
To install xmlschema
, use the following command:
pip install xmlschema
Both of these packages provide easy-to-use APIs for XML validation within Python scripts. You can refer to their respective documentation and examples to get started with XML validation using third-party packages! 😎🔧
Conclusion and Reader Engagement 📝💬
Congratulations! You now have the knowledge and tools to validate XML files against a schema using Python. Whether you prefer the simplicity of the standard library or the added flexibility of third-party packages, XML validation is within your grasp! 🙌✨
We hope this guide has been helpful and insightful. If you have any questions, suggestions, or additional tips regarding XML validation in Python, please share them in the comments below. Let's start a conversation! 💬🤝
Now go forth, validate your XML files with confidence, and share your success stories with the Python community! Happy coding! 🐍💻🌟
#StayCurious #KeepExploring #PythonXMLValidation
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
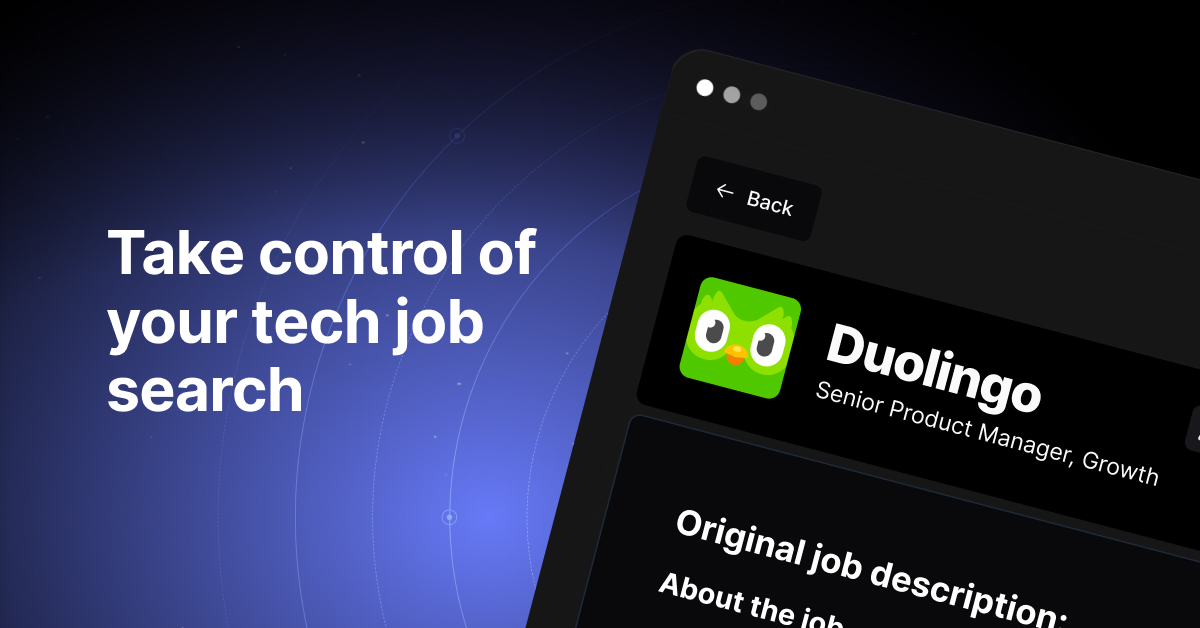