Using pickle.dump - TypeError: must be str, not bytes
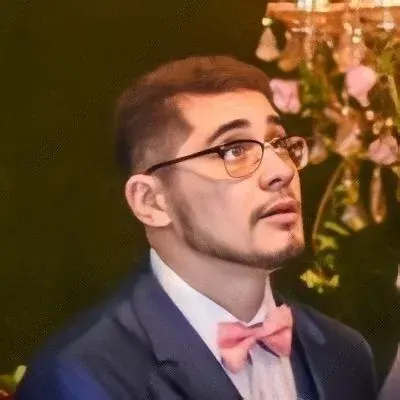
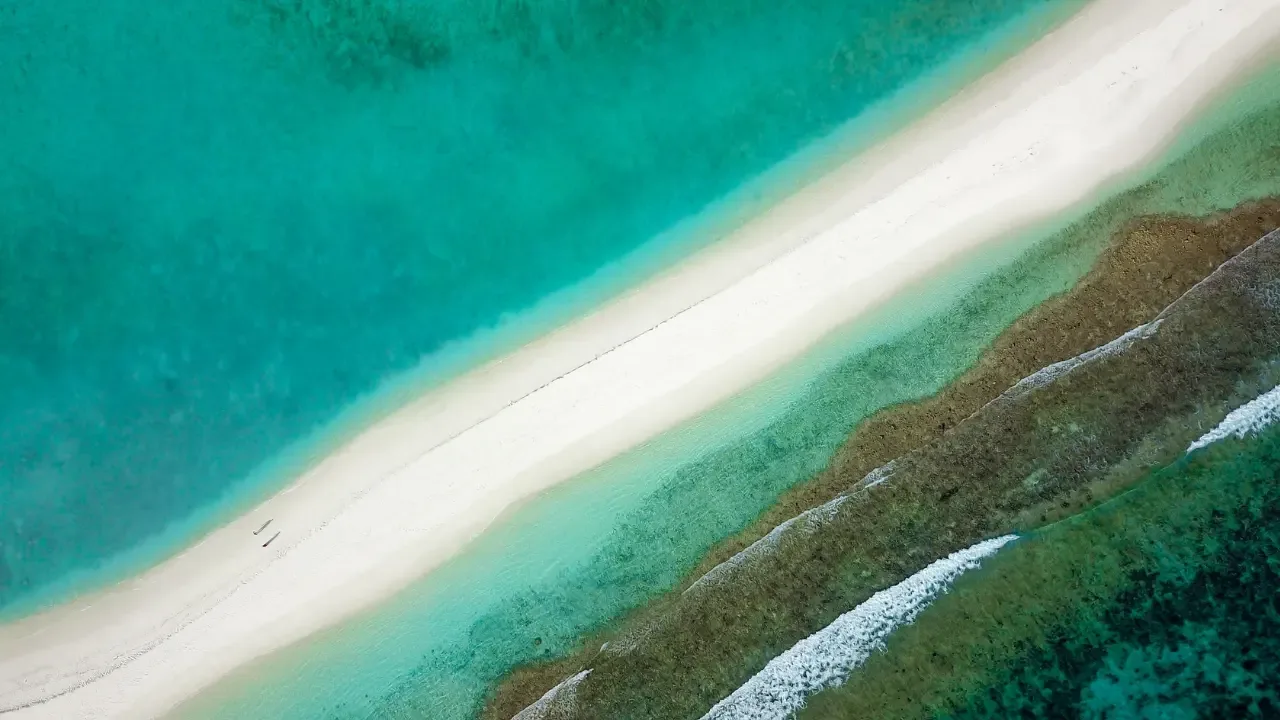
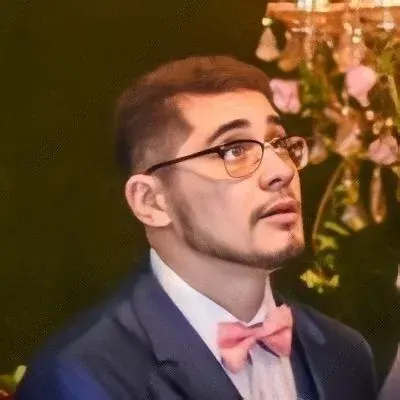
How to Fix the TypeError: must be str, not bytes
Error When Using pickle.dump
<p class="emoji">🔐💔</p>
Are you trying to pickle a simple dictionary in Python, only to be met with a cryptic TypeError: must be str, not bytes
error? Don't worry, you're not alone! This error is a common stumbling block that many Python developers encounter when using the pickle.dump
function. In this blog post, we'll explore why this error occurs and provide easy-to-implement solutions to help you overcome it. So, let's dive in and solve this pickle puzzle together!
Understanding the Error
The TypeError: must be str, not bytes
error message typically occurs when you're trying to dump an object that contains non-string data into a file using the pickle.dump
function. In the provided code snippet, the dictionary mydict
contains string values for keys like 'name'
, 'gender'
, and 'age'
. So, why are we encountering this error? Let's take a closer look at the code and find out.
The Culprit: open
in Write Mode
In the given code, the file is opened in write mode ('w'
) using the open
function. In Python 3.x, the default mode for opening files is text mode, which means that the data being written to the file is expected to be in string format. However, the pickle.dump
function deals with binary data rather than strings, causing the mismatch and resulting in the dreaded TypeError
.
Solution: Open the File in Binary Mode
To resolve this issue, we need to open the file in binary mode ('wb'
) instead of text mode. This can be achieved by changing the 'w'
argument to 'wb'
in the open
function. By doing so, we tell Python to treat the file as binary and allow the pickled binary data to be written successfully.
Here's an updated version of the code with the necessary change:
import os
import pickle
def storvars(vdict):
with open('varstor.txt', 'wb') as f:
pickle.dump(vdict, f)
return
mydict = {'name': 'john', 'gender': 'male', 'age': '45'}
storvars(mydict)
By modifying the file opening mode, we align the expectations of the file object with the format of the data being dumped, effectively resolving the TypeError
issue.
Calling All Python Enthusiasts!
<p class="emoji">💡🚀💬</p>
Did this solution help you fix the TypeError: must be str, not bytes
error? We hope it did! If you found this blog post useful or have any questions, we'd love to hear from you. Leave a comment below and let's start a conversation around Python pickling and other related topics. Happy coding!
<p class="emoji">✨🐍💻</p>