Using Pandas to pd.read_excel() for multiple worksheets of the same workbook
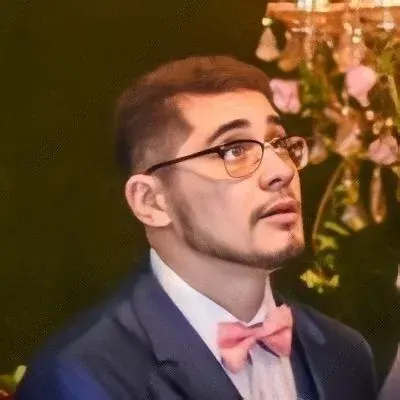
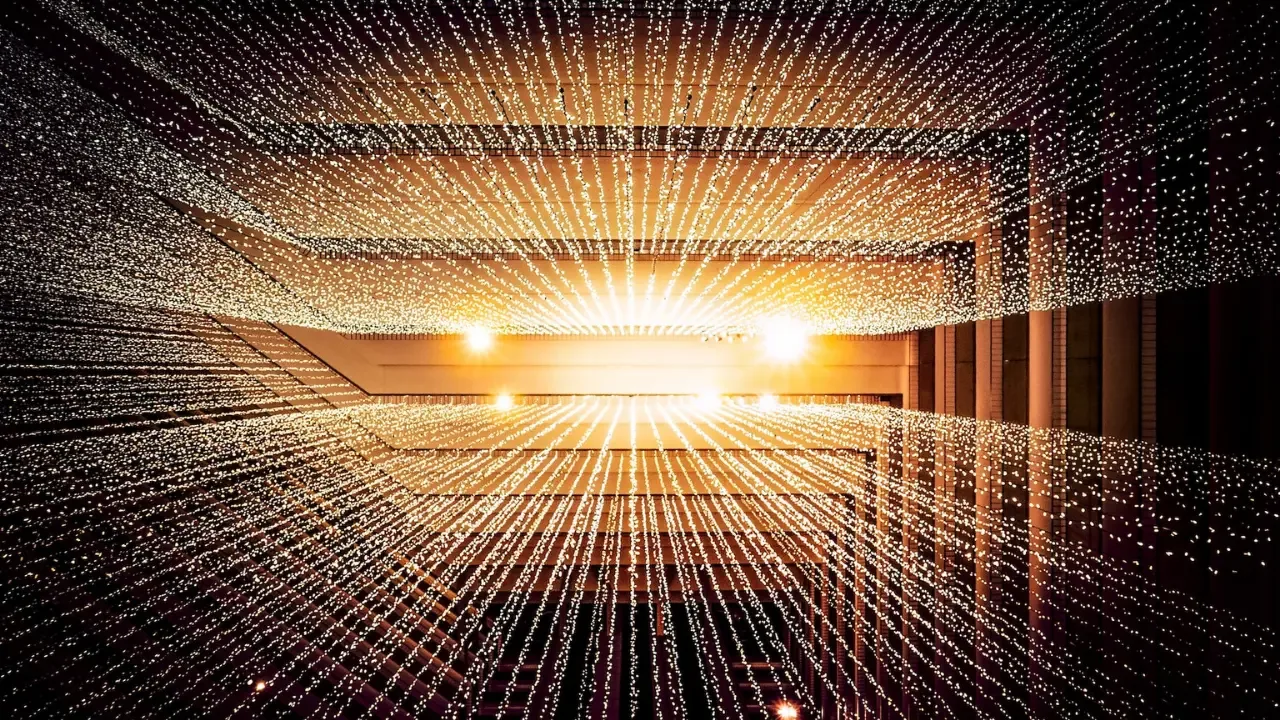
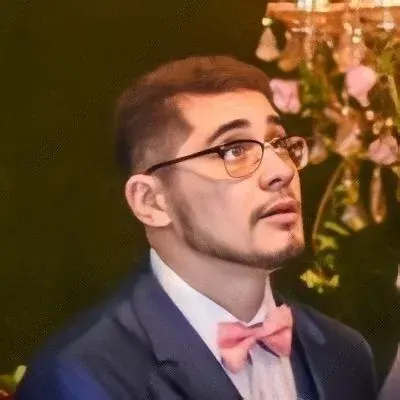
Using Pandas to pd.read_excel() for multiple worksheets of the same workbook
š Hey there pandas enthusiasts! Have you ever come across a situation where you needed to extract data from multiple worksheets of the same Excel workbook using the pandas library? š¼ Well, you're not alone! In this blog post, I'll show you how to tackle this common issue and provide some easy solutions. So, let's dive in! š»
The Problem
š So, you've got this huge Excel file (.xlsx) that you're trying to process with pandas. The catch is that you only need data from specific worksheets within that file. You realize that when you use pd.read_excel()
, it seems to load the entire workbook rather than just the worksheet you're interested in. This can be frustrating and inefficient, especially if you have a large dataset. š«
The Solution
š Luckily, pandas provides a simple solution to this problem. The key is to use the sheet_name
parameter of the pd.read_excel()
function. Let me show you some easy ways to overcome this issue. š
Solution 1: Reading a Single Worksheet
š If you only need data from a single worksheet, it's as easy as specifying the sheet_name
parameter with the name or index of the desired sheet. For example:
import pandas as pd
data = pd.read_excel('your_file.xlsx', sheet_name='Sheet1')
In this case, only the data from "Sheet1" will be loaded, saving you precious computing resources and time. š¤©
Solution 2: Reading Multiple Worksheets
š Now, what if you need data from multiple worksheets? No problem! You can pass a list of sheet names or indices to the sheet_name
parameter. Here's an example:
import pandas as pd
sheets = ['Sheet1', 'Sheet2']
data = pd.read_excel('your_file.xlsx', sheet_name=sheets)
With this approach, you can easily load the specified worksheets altogether into a dictionary, where each sheet name becomes a key and the corresponding data is stored as a DataFrame. š
The Call-to-Action
š And there you have it, my friends! By using the sheet_name
parameter wisely, you can efficiently extract data from specific worksheets within your Excel workbook using pandas. No need to suffer the whole workbook being read in twice anymore! šŖ
If you found this blog post helpful, don't forget to share it with your fellow pandas enthusiasts. š£ Also, feel free to leave a comment below if you have any questions or if there are any other pandas-related topics you'd like me to cover in future blog posts. Let's keep the pandas love going! ā¤ļø