Using global variables in a function
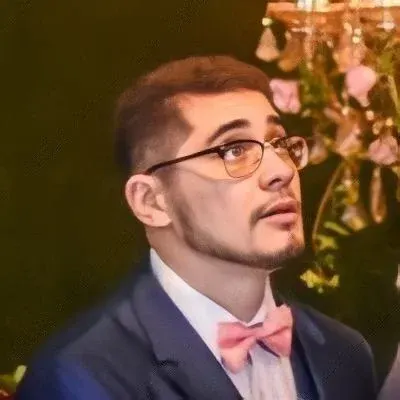
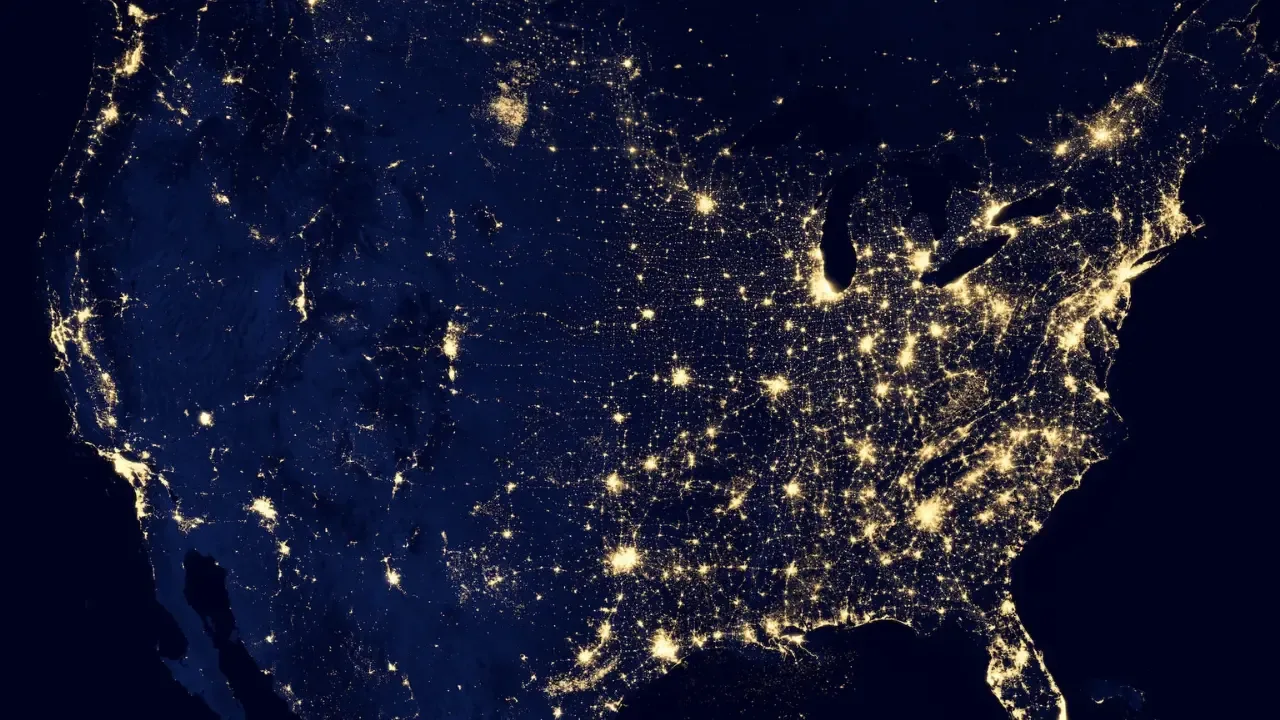
🌍 Using Global Variables in a Function
Are you struggling with creating or using global variables inside a function? Maybe you're wondering how to access a global variable defined in one function from another? Don't worry, you're not alone! Many programmers encounter these challenges, but luckily there are easy solutions. 💪
💼 Understanding the Problem
When you define a variable inside a function, it is considered a local variable. This means that the variable is only accessible within that particular function. If you try to access the variable outside of the function, you might encounter an UnboundLocalError
because the variable is not defined in the broader scope.
🤔 The Global Keyword
To overcome this issue and make a variable accessible globally, you need to use the global
keyword. By using global
, you're telling Python that you want to refer to the variable from the global scope, not just within the function. This way, you can access the variable from anywhere within your program.
📝 Easy Solutions
Solution 1: Declare the Variable as Global
To create or use a global variable inside a function, you need to declare it as global before assigning a value to it. Here's an example:
def my_function():
global my_variable
my_variable = 42
my_function()
print(my_variable) # Output: 42
In this example, we declare my_variable
as global within the my_function
before assigning the value 42
to it. As a result, we can later access the variable outside the function as well.
Solution 2: Use a Return Statement
Alternatively, if you want to use the value of a variable defined inside a function in other functions, you can use the return statement. Here's an example:
def set_global_variable():
global my_variable
my_variable = 42
def use_global_variable():
print(my_variable)
set_global_variable()
use_global_variable() # Output: 42
In this example, we set the value of my_variable
within the set_global_variable
function with the help of the global
keyword. Then, we call the use_global_variable
function, which prints the value of my_variable
. The value is accessible because it was set globally in the previous function.
📣 Call-to-Action
Now that you have a better understanding of how to use global variables in functions, it's time to put it into practice! Experiment with these easy solutions and see how they can enhance your coding skills.
Share your experiences or any additional tips in the comments below. Let's help each other grow 🌱 and conquer those programming challenges together!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
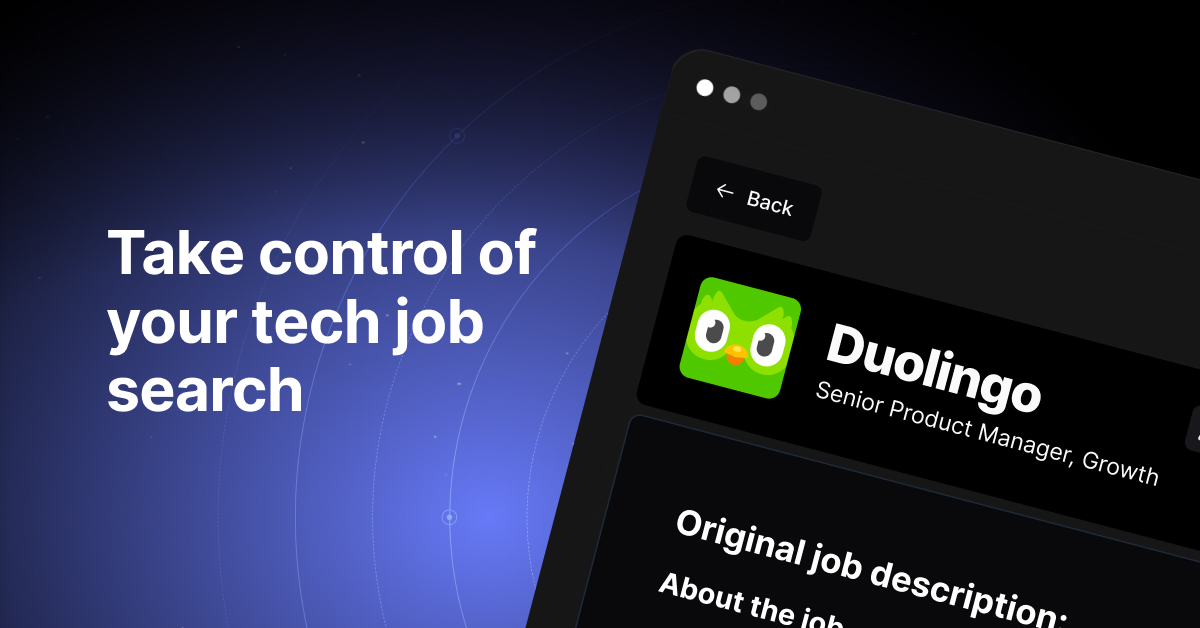